Python os.get_terminal_size() Method
-
Syntax of Python
os.get_terminal_size()
Method -
Example 1: Use
os.get_terminal_size()
to Get the Terminal Size -
Example 2: The
os.get_terminal_size()
Raises anOSError
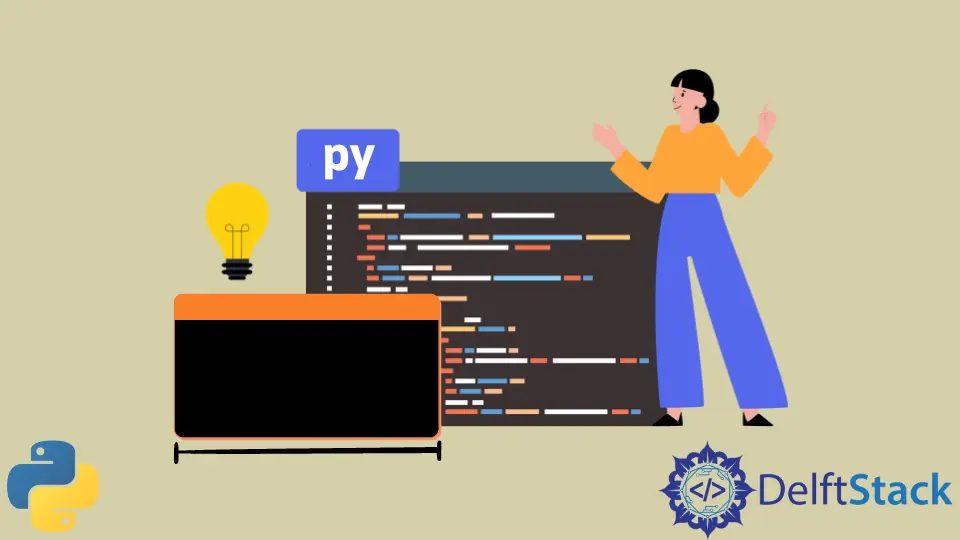
The get_terminal_size()
method is a part of the os
module. The os
is a Python module containing various methods and system calls that enable us to interact with the operating system.
As the name suggests, the get_terminal_size()
method returns the size of the terminal window associated with the passed file descriptor.
Syntax of Python os.get_terminal_size()
Method
os.get_terminal_size(fd=STDOUT_FILENO)
Parameters
Type | Description | |
---|---|---|
fd |
integer | A file descriptor associated with a terminal. The default value is set to STDOUT_FILENO , which stands for standard output. |
Returns
The get_terminal_size()
method returns an object of class terminal_size
. This class is a subclass of the class tuple
.
The object represents a tuple
of 2
values, columns
and lines
. These two values represent the size of the terminal window.
Example 1: Use os.get_terminal_size()
to Get the Terminal Size
Create a Python file and enter the following Python code into it. Next, open any terminal and execute the Python file using this command python <filename>.py
.
import os
size = os.get_terminal_size()
print(size)
Output:
os.terminal_size(columns=120, lines=30)
As we can see, the output returns the number of columns and the number of lines (or rows) in the terminal. Try resizing the terminal and executing the code to see changes in the output.
Note that this will only work if any terminal is open in the system.
Example 2: The os.get_terminal_size()
Raises an OSError
If the file descriptor is not associated with any terminal, the get_terminal_size()
raises an OSError
, which signifies a system-related error. To learn more about the OSError
, refer to the official Python documentation here.
A better and more secure way to use the get_terminal_size()
method would be using the try
and except
blocks. Refer to the following Python code.
import os
try:
size = os.get_terminal_size()
print(size)
except OSError:
print("Please open a terminal.")
If a terminal window is not open, Please open a terminal.
will be the output; otherwise, it will output the size of the terminal window. Note that we are explicitly catching the OSError
in the above Python snippet.