Python os.get_exec_path() Method
-
Understanding
os.get_exec_path()
-
Syntax of Python
os.get_exec_path()
Method -
Example 1: Use the
os.get_exec_path()
Method in Python -
Example 2: Use an
environment
Parameter in theos.get_exec_path()
Method in Python -
Example 3: Use a
for
Loop in theos.get_exec_path()
Method in Python - Example 4: Executing External Commands
- Example 5: Dynamic Script Behavior
- Example 6: System Configuration Analysis
- Considerations and Caveats
- Conclusion
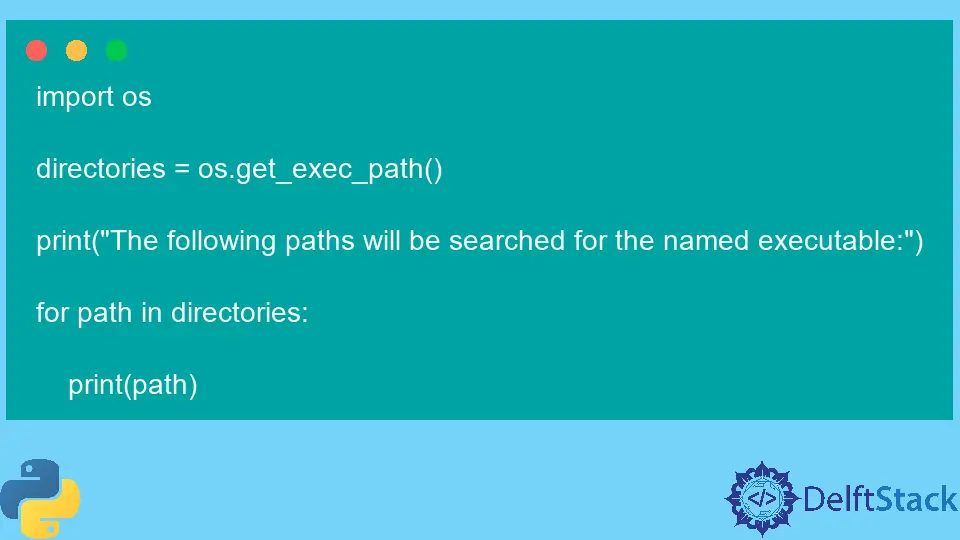
Python, a versatile and powerful programming language, provides a rich set of libraries and modules to simplify various tasks. The os
module, which is part of the Python Standard Library, offers functionalities related to interacting with the operating system. One such useful method is os.get_exec_path()
.
Python os.get_exec_path()
method is an efficient way of extracting a list of required directories. While launching any process, the directories will be searched for the specified executable.
In this article, we will delve into the details of this method, exploring its purpose, syntax, and practical use cases.
Understanding os.get_exec_path()
Python os.get_exec_path()
method returns the list of directories that will be searched for executable files when launching a new process.
In simpler terms, it provides the system’s executable search path. This path is crucial when running external commands or programs from within a Python script.
Syntax of Python os.get_exec_path()
Method
os.get_exec_path(environment=None)
Parameters
environment = None |
Optional. The default value is None ; when the environment is set to None , the environ environment is used. This parameter is a dictionary of environment variables offered by Python. |
Return
The return type of this method is a list of all the paths searched for the specified executable while launching a process.
Example 1: Use the os.get_exec_path()
Method in Python
This example utilizes the os.get_exec_path()
method to retrieve and print the list of directories that the system will search for a named executable. The code provides a simple and informative way to inspect the executable search path on the system, aiding in understanding and verifying the locations where the system looks for executables.
import os
print("The following paths will be searched for the named executable:")
print(os.get_exec_path())
Output:
The following paths will be searched for the named executable:
['/opt/swift/swift-5.0-RELEASE-ubuntu14.04/usr/bin/', '/usr/local/sbin', '/usr/local/bin', '/usr/sbin', '/usr/bin', '/sbin', '/bin']
No parameter is entered in the above code, so the default environment environ
is used. The environ
is a mapping object that contains all the user’s environmental variables.
Example 2: Use an environment
Parameter in the os.get_exec_path()
Method in Python
This example uses the os.get_exec_path()
method with an environment variable dictionary (environment
) to retrieve and print the list of directories that the system will search for a named executable. The provided environment variable (HOME
) influences the executable search path, resulting in a customized list of directories that includes the specified environment variable.
import os
environment = {"HOME": "/home/user"}
print("The following paths will be searched for the named executable:")
print(os.get_exec_path(environment))
Output:
The following paths will be searched for the named executable:
['/bin', '/usr/bin']
When a system-related error occurs while using this method, then an OSError
is thrown.
Example 3: Use a for
Loop in the os.get_exec_path()
Method in Python
This example uses os.get_exec_path()
to retrieve the system’s executable search path and prints each directory in the path. The script provides a clear display of the directories where the system will search for a named executable, aiding in understanding the order of the search path.
import os
directories = os.get_exec_path()
print("The following paths will be searched for the named executable:")
for path in directories:
print(path)
Output:
The following paths will be searched for the named executable:
/opt/swift/swift-5.0-RELEASE-ubuntu14.04/usr/bin/
/usr/local/sbin
/usr/local/bin
/usr/sbin
/usr/bin
/sbin
/bin
The os.get_exec_path()
method uses a set of environment variables available to Python through the os.environ
of the os
module.
Example 4: Executing External Commands
This example utilizes os.get_exec_path()
to retrieve the system’s executable search path. It then constructs and executes an external command, such as 'ls'
on Unix-like systems, by combining the first directory in the executable path with the desired command.
The script demonstrates running commands based on the system’s executable search path, offering flexibility and adaptability in external command execution.
import os
# Get the executable path
exec_path = os.get_exec_path()
# Run an external command (e.g., ls on Unix-like systems)
os.system(f"{exec_path[0]}/ls")
In this example, we obtain the executable path using os.get_exec_path()
and then run the 'ls'
command using the first directory in the list. This ensures that the correct executable is invoked, avoiding potential issues.
It’s worth noting that the example command ('ls'
) is specific to Unix-like systems. If you intend to run different commands or work on non-Unix systems, adjustments may be needed.
Additionally, caution should be exercised when constructing and executing commands to ensure security and compatibility.
Example 5: Dynamic Script Behavior
The example below defines a function, run_executable
, that takes the name of an executable as an argument. The function searches through the system’s executable search path using os.get_exec_path()
and attempts to run the specified executable if found.
The script provides a dynamic way to execute programs, enhancing adaptability across various environments. The example usage demonstrates running the 'python'
executable based on the system’s executable search path.
import os
def run_executable(executable_name):
exec_path = os.get_exec_path()
for path in exec_path:
full_path = os.path.join(path, executable_name)
if os.path.isfile(full_path):
os.system(full_path)
break
else:
print(f"{executable_name} not found in the executable path.")
# Example usage
run_executable("python")
Here, the run_executable
function takes an executable name as an argument and searches through the executable path to find and run the corresponding executable. This dynamic behavior allows scripts to adapt to different system configurations.
Example 6: System Configuration Analysis
The example below defines a function, analyze_executable_path
, which retrieves and prints the system’s executable search path using os.get_exec_path()
. The script then calls this function to analyze and display the executable search path, providing a quick overview of the directories where the system will search for executables.
import os
def analyze_executable_path():
exec_path = os.get_exec_path()
print("Executable Path:")
for path in exec_path:
print(f"- {path}")
# Analyze the system's executable path
analyze_executable_path()
This code provides a simple script to analyze and print the executable path. Understanding the path can be crucial for debugging or ensuring compatibility across different environments.
Considerations and Caveats
While os.get_exec_path()
is a powerful tool, there are a few considerations to keep in mind:
- Platform Differences: The executable path may vary across different operating systems. It’s essential to account for platform-specific behaviors when designing scripts that rely on this method.
- Security: Avoid blindly trusting and executing commands based on the executable path without proper validation. Always sanitize inputs to prevent potential security vulnerabilities.
- Permission Issues: Ensure that the script has the necessary permissions to execute files in the directories listed in the executable path.
Conclusion
The os.get_exec_path()
method in Python’s os
module provides a convenient way to access the system’s executable search path. Whether you’re running external commands, adapting script behavior dynamically, or analyzing system configurations, understanding and utilizing this method can enhance the robustness and portability of your Python scripts.
As with any system-related functionality, it’s crucial to be aware of platform differences and handle potential security concerns appropriately.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn