Python os.fsync() Method
-
Syntax of Python
os.fsync()
Method -
Example 1: Use
os.fsync()
With a File Descriptor -
Example 2: Use
os.fsync()
With a File Object -
Example 3: Use
os.fsync()
in a Scenario Where Data Integrity Is Critical, -
OSError: [Errno 9] Bad file descriptor
Occurrence inos.fsync()
Method - Conclusion
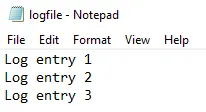
When you perform write operations to a file in Python using standard file handling mechanisms like open
, write
, and close
, there’s no guarantee that the changes are immediately flushed to disk. The operating system may buffer these changes for better performance.
While this buffering improves efficiency, it poses a risk of data loss if the system crashes before the changes are written to disk.
Python has an os
module that gives access to various system calls and operating system methods. The fsync()
method is a part of the os
module and allows us to write a file to the disk forcefully.
It’s a method that ensures that any changes made to a file’s data or metadata are physically written to disk, thereby synchronizing the file state with the operating system’s file system.
Syntax of Python os.fsync()
Method
os.fsync(fd)
Parameters
Type | Description | |
---|---|---|
fd |
integer | A file descriptor associated with a valid resource. |
Instead of a file descriptor, if the parameter is a file object, we must ensure that all the buffers linked with the passed file object are successfully written to the disk. Considering fd
is the parameter name, we have to use fd.flush()
.
Subsequently, we can use os.fsync()
as os.fsync(fd.fileno())
to forcefully save the file to the disk.
Returns
The fsync()
method doesn’t return anything.
Example 1: Use os.fsync()
With a File Descriptor
Python’s os.fsync
function provides a means to accomplish this by forcing the operating system to synchronize file updates with the underlying storage medium.
Let’s dive into a code example that demonstrates the usage of os.fsync
with a file descriptor.
data.txt
:
This is a text file.
Example Code:
import os
# Open or create a file in read-write mode
fd = os.open("data.txt", os.O_RDWR | os.O_CREAT)
# Data to be written to the file
data = "I wish to write this information to the file."
# Write data to the file descriptor
os.write(fd, data.encode()) # write to the file
# Forcefully synchronize changes to disk
os.fsync(fd) # forcefully writing to the file
# Close the file descriptor
os.close(fd) # close the file descriptor
In this code snippet, the os
module is imported to access operating system functionalities. os.open
is utilized to open an existing file in read-write mode or create a new file, returning a file descriptor (fd
) representing the opened file.
The data string to be written to the file is defined, and os.write
is used to encode and write the string data to the file referenced by fd
. Subsequently, os.fsync
is invoked to force synchronization of file updates with the underlying disk storage, ensuring immediate persistence of any changes made to the file.
Lastly, os.close
is employed to close the file descriptor, releasing associated system resources. This process facilitates reliable data handling and preserves data integrity within file operations.
The Python code above creates a data.txt
file if it doesn’t exist inside the current working directory. Next, it writes the encoded string data to the file, writes it to the disk, and closes the file descriptor.
Output:
The file "data.txt"
will contain the specified data, and the changes will be reliably persisted to disk, ensuring data integrity.
Example 2: Use os.fsync()
With a File Object
When working with file operations, it’s essential to ensure that data is reliably written to disk to maintain data integrity. This becomes particularly crucial in scenarios where system failures or crashes could lead to data loss if changes are not synchronized promptly.
Python provides methods like flush()
and os.fsync()
to handle this synchronization process effectively.
Let’s explore a code example that demonstrates the usage of these methods.
data2.txt
:
This is a text file.
Example Code:
import os
# Open or create a file in write mode with read capability
f = open("data2.txt", "w+")
# Define the data string to be written to the file
data = "I wish to write this information to the file."
# Write data to the file
f.write(data)
# Ensure all existing buffers are written to disk
f.flush() # ensuring all the existing buffers are written
# Forcefully synchronize changes to disk
os.fsync(f.fileno()) # forcefully writing to the file
# Close the file
f.close()
In this code snippet, the os
module is imported to access operating system functionalities, enabling system-level operations.
The code opens or creates a file named data2.txt
in write mode with read capability using the open
function, establishing a file object f
for file interaction. Following the definition of a data string to be written to the file, the write
method of the file object f
is employed to commit the data to the file.
In order to ensure data reliability, the flush
method is invoked, synchronizing buffered changes with the disk to enhance integrity. Subsequently, os.fsync(f.fileno())
forcefully synchronizes changes to the file with the underlying disk storage, reducing the risk of data loss in system failures by immediately persisting pending changes.
Finally, the close
method is utilized to release system resources associated with the file object, concluding the file operation process.
Output:
Example 3: Use os.fsync()
in a Scenario Where Data Integrity Is Critical,
Let’s explore a practical example demonstrating the usage of os.fsync()
in a scenario where data integrity is critical, such as logging data to a file in a multi-threaded environment.
logfile.txt
:
Log entry 1
Log entry 2
Log entry 3
Example Code:
import os
import threading
# Function to write data to a log file
def write_to_log(data):
with open("logfile.txt", "a") as f:
# Acquire lock to ensure thread safety
lock.acquire()
try:
# Write data to the file
f.write(data + "\n")
# Flush changes to disk
f.flush()
# Synchronize file state with disk
os.fsync(f.fileno())
finally:
# Release lock
lock.release()
# Create a lock to ensure thread safety
lock = threading.Lock()
# Sample data to log
log_data = ["Log entry 1", "Log entry 2", "Log entry 3"]
# Create threads to write data to the log file concurrently
threads = []
for data in log_data:
thread = threading.Thread(target=write_to_log, args=(data,))
threads.append(thread)
thread.start()
# Wait for all threads to complete
for thread in threads:
thread.join()
print("All log entries have been written to the file.")
In this example, we have a function write_to_log()
responsible for writing data to a log file (logfile.txt
).
We use a lock (threading.Lock()
) to ensure that multiple threads can write to the file safely without interfering with each other. Inside the function, we acquire the lock before writing to the file, ensuring that only one thread can write at a time.
After writing data to the file, we flush the changes to ensure they are written to disk, and then we synchronize the file state with the disk using os.fsync()
to guarantee data integrity.
We then create multiple threads to simulate concurrent logging activity. Each thread writes a log entry to the file by calling the write_to_log()
function with the log data.
Once all threads have completed writing their log entries, we print a message indicating that all log entries have been written to the file.
Output:
This message indicates that all log entries have been successfully written to the log file (logfile.txt
).
Since we have used os.fsync()
after flushing the changes to disk, we can be confident that the data has been reliably persisted, ensuring data integrity even in the event of system failures or crashes.
OSError: [Errno 9] Bad file descriptor
Occurrence in os.fsync()
Method
This demonstrates the importance of ensuring a proper sequence of operations when using file descriptors and os.fsync()
to maintain data integrity and avoid errors.
example.txt
:
Example text.
Example Code:
import os
# Open a file in write mode
fd = os.open("example.txt", os.O_RDWR | os.O_CREAT)
# Write some data to the file
os.write(fd, b"Hello, World!")
# Close the file descriptor
os.close(fd)
# Attempt to synchronize changes with disk after closing the file descriptor
os.fsync(fd)
In this example, we open a file named example.txt
in read-write mode with the ability to create the file if it does not exist. We then write the string "Hello, World!"
to the file using os.write()
.
After writing the data, we close the file descriptor using os.close()
to release system resources associated with the file.
However, instead of synchronizing changes with the disk before closing the file descriptor, we attempt to call os.fsync()
after closing it. This triggers an OSError
, as the file descriptor is no longer valid after being closed, and attempting to synchronize changes with a closed file descriptor is not allowed.
When executing this code, the output will be an error message indicating that an error occurred due to attempting to call os.fsync()
on a closed file descriptor.
Conclusion
The os.fsync()
method plays a crucial role in ensuring data integrity by synchronizing file updates with the disk.
In Python, file operations are not always immediately flushed to disk, posing a risk of data loss in the event of system crashes or failures. However, os.fsync()
addresses this issue by forcing changes to be written to disk promptly.
The method is especially useful in scenarios like logging data in multi-threaded environments, where data consistency is critical.
Although os.fsync()
enhances data reliability, it must be used correctly, as attempting to synchronize changes with a closed file descriptor can lead to errors like OSError: [Errno 9] Bad file descriptor
.
Therefore, understanding its usage and ensuring a proper sequence of operations is essential for maintaining data integrity and preventing errors in file-handling processes.