Python os.fspath() Method
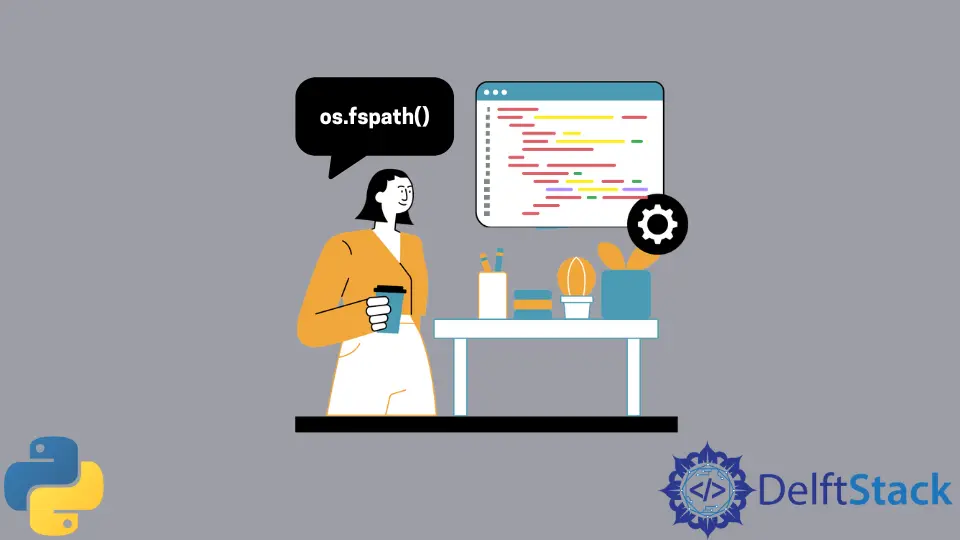
The fspath()
method belongs to the os
module of Python programming. The os
module offers utilities to work with the operating system.
The fspath()
method returns the file system representation of the path. Remember, the __fspath__()
is called, which returns either bytes
, os.PathLike
, or str
.
Syntax
os.fspath(path)
Parameters
Type | Explanation | |
---|---|---|
path |
string/bytes | A file system path. |
Returns
The fspath()
method returns the file system representation of a path. The output’s type depends on the type of input.
- If the input is a string, the output is a string.
- If the input is a bytes string, the output is a bytes string.
- If the input is
os.PathLike
, the output is anos.PathLike
.
Exception
We will get the TypeError
exception if the path
is neither the bytes
type nor the str
type.
Examples
String Path
Example Code:
from os import fspath
# here, the `path` is a string type
path = "/home/users/documents/data.json"
print(fspath(path))
In the code snippet provided, the from os import fspath
statement imports the fspath
function from the os
module.
The fspath
function is used to convert a path-like object to a string. It’s particularly useful when dealing with objects that represent file paths, such as pathlib.Path
objects.
Since path
is already a string, using fspath
in this case doesn’t have any effect, and the output will be the same as the input string.
However, the fspath
function becomes more relevant when dealing with objects that represent paths, such as instances of the pathlib.Path
class.
Output:
/home/users/documents/data.json
The output is the same string /home/users/documents/data.json
.
The purpose of fspath
is more evident when dealing with objects that represent paths, such as pathlib.Path
objects, where it ensures that the object is converted to its string representation.
Bytes String Path
Example Code:
from os import fspath
# here, the `path` is bytes string type
path = b"/home/users/documents/data.json"
print(fspath(path))
In this example code, we use the fspath
function from the os
module to convert a bytes string (path = b"/home/users/documents/data.json"
) to a regular string.
The fspath
function is designed to handle various types of path-like objects, including bytes string.
Output:
b'/home/users/documents/data.json'
In this case, fspath
helps handle the conversion from a bytes string representing a file path to a regular string that can be more easily manipulated or used in various file-related operations.
Example Code:
import os
# create bytes' type instance
bytesObject = bytes(10)
print(repr(os.fspath(bytesObject)))
Output:
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
Neither String Nor Bytes Path
Example Code:
import random
import os
# generate a random value between 1-5
# and assign to `path` variable
path = random.randint(1, 5)
# It will raise an exception
print(os.fspath(path))
In the code snippet above, it is generating a random integer between 1 and 5 using random.randint(1, 5)
and assigning it to the variable path
. Then, it attempts to use os.fspath(path)
to convert this random integer to a path-like object, but this will raise an exception.
The reason is that os.fspath()
expects its argument to be a path-like object, such as a string or an object with a __fspath__
method, and not an integer. In this case, path
is an integer generated by random.randint(1, 5)
, and integers are not valid path-like objects.
Next time, if the user wants to use os.fspath()
successfully, the user should provide a valid path-like object, such as a string or a pathlib.Path
object.
Using an integer directly as a path will result in a TypeError
.
Output:
Traceback (most recent call last):
File "/home/jdoodle.py", line 6, in <module>
print(os.fspath(path))
TypeError: expected str, bytes or os.PathLike object, not int
According to the documentation, if we pass bytes
or str
, it would be returned unchanged. Otherwise, the __fspath__()
function would be called and will return its value as long as it’s bytes
or str
object.
In all other cases except this, the TypeError
exception is raised. So, remember that if we have the correct path
type but are not written correctly, for instance, if we miss double quotes, then we will get SyntaxError: invalid syntax
.
Conclusion
The os.fspath()
method is a part of Python’s os
module, providing utilities for working with the operating system.
This method converts a path-like object to its string representation, supporting various types like strings, bytes, or os.PathLike
objects. The output’s type depends on the input, and it raises a TypeError
if the input is neither bytes nor a string.
Examples demonstrate its usage with string and bytes paths, ensuring proper conversion. If the input is neither a string nor bytes, attempting to convert an integer path results in a TypeError
. Correct usage involves providing valid path-like objects for successful conversion.