Python os.fdopen() Method
-
Syntax of Python
os.fdopen()
Method -
Example 1: Use the
os.fdopen()
Method in Python -
Example 2: Read the File After Using the
os.fdopen()
Method -
Example 3: Use the
os.fdopen
Method With theos.open()
Method - Conclusion
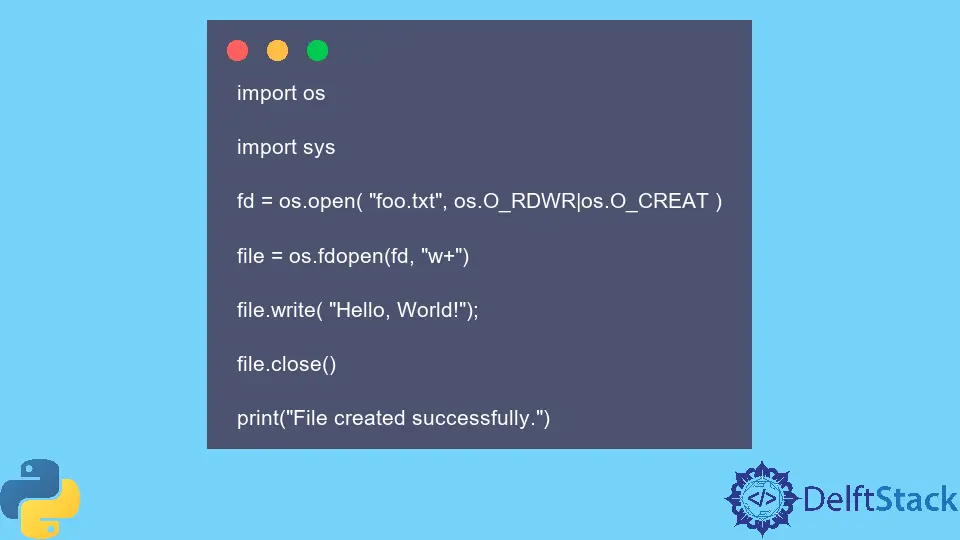
The os
module is a valuable tool for file operations in Python, offering various functions to communicate with the operating system. One such function that often goes unnoticed is os.fdopen()
.
This function allows us to convert a file descriptor (fd
) into a corresponding file object, unleashing a range of possibilities for handling files with greater flexibility.
Python’s os. open ()
method returns an open file object connected to the fd
file descriptor. File descriptors are integer values that represent open files in an operating system.
It is an alias of the os.open()
method.
Both methods don’t have a difference in their working except that the os.fdopen()
method’s first argument should be an integer.
Syntax of Python os.fdopen()
Method
os.fdopen(fd, mode)
Parameters
fd |
It is a file descriptor for which a file object is needed to open. |
mode |
It is an optional parameter representing the mode of the newly opened file. The default value of the mode is an octal numeric integer 0o777 . |
The most used values of the mode parameter are r for reading a file, w for writing/truncating a file, and a for appending the file. |
Return
This method returns the newly opened file’s path connected to the file descriptor.
Example 1: Use the os.fdopen()
Method in Python
The code below exemplifies the use of os.fdopen
to open or create a file, write content to it, and close the file object.
foo.txt
:
Text file.
Example Code:
import os
import sys
# Open or create a file named 'foo.txt' in read-write mode
fd = os.open("foo.txt", os.O_RDWR | os.O_CREAT)
# Convert the file descriptor to a file object in write-plus mode ('w+')
file = os.fdopen(fd, "w+")
# Write the text "Hello, World!" to the file
file.write("Hello, World!")
# Close the file object
file.close()
# Display a success message
print("File created successfully.")
The code begins by importing the necessary modules, including os
, which provides file and directory manipulation functions.
In creating the file, the os.open
function is employed to open or create the file named 'foo.txt'
with read-write permissions (os.O_RDWR
) and creation flag (os.O_CREAT
). The resulting file descriptor (fd
) is a reference to the opened file.
Then, os.fdopen
converts the file descriptor (fd
) to a file object (file
) with write-plus mode ('w+'
). This mode allows both reading and writing, positioning the file pointer at the beginning.
The write
method is used to add the text Hello, World!
to the file. Proper resource management is ensured by closing the file object using the close
method.
Finally, a success message is printed to the console, indicating that the file creation and writing processes were successful.
Output:
Assuming the code is executed successfully, the output will be File created successfully.
While using the os.fdopen()
method, we can use one or more modes simultaneously by using the vertical bar |
.
Example 2: Read the File After Using the os.fdopen()
Method
The Python code below showcases the utilization of the os.fdopen
function along with other functionalities from the os
module to perform essential file operations.
It will read the file after using the os.fdopen()
method.
File.txt
:
This is a sample text file.
Example Code:
import os
# Define the path for the new file
path_address = "File.txt"
# Set file permissions (mode)
mode_set = 0o666
# Define flags for opening the file
flags = os.O_CREAT | os.O_RDWR
# Open the file with specified flags and mode
descriptor = os.open(path_address, flags, mode_set)
# Convert the file descriptor to a file object in write-plus mode ('w+')
file = os.fdopen(descriptor, "w+")
# Display a message indicating the creation of the new file
print("The new file has been created.")
# Define a line to be written to the file
line = "Hello, World! Programming is fun!"
# Write the encoded line to the file using the file descriptor
os.write(descriptor, line.encode())
# Display a success message for the text entry
print("The text has been successfully entered into the file.")
# Close the file object
file.close()
# Display a message indicating the successful closure of the file descriptor
print("The file descriptor has been closed successfully.")
Initially, it defines the path for a new file, "File.txt,"
and specifies file permissions. Leveraging the os.O_CREAT
and os.O_RDWR
flags, the code opens or creates the file, acquiring a file descriptor.
Subsequently, it employs the os.fdopen
function to seamlessly convert this file descriptor into a file object in write-plus mode ('w+'
), enabling higher-level file operations with standard Python file handling methods.
The code then proceeds to write a designated line of text, "Hello, World! Programming is fun!"
, to the file using the os.write
method and the obtained file descriptor.
The file object is closed through the close
method, ensuring proper resource management and subsequently releasing the associated file descriptor.
Throughout the execution, the console displays informative messages, confirming the successful creation of the new file, the entry of text, and the meticulous closure of the file descriptor.
Output:
It is a healthy practice for any programmer to close all the newly opened files using the os.close()
method.
Example 3: Use the os.fdopen
Method With the os.open()
Method
In this example, it illustrates the effectiveness of using os.fdopen
in conjunction with os.open
to handle files efficiently.
Encapsulating the process within a with
statement ensures both streamlined code and automatic closure of the file descriptor.
File.txt
:
This is a sample text file.
Example Code:
import os
# Open or create the file using os.open and convert to file object with os.fdopen
with os.fdopen(os.open("File.txt", os.O_CREAT | os.O_RDWR), "w") as fd:
# Write "Hello, World!" to the file using the file descriptor
fd.write("Hello, World!")
# Display a success message after the file descriptor is closed
print("The file descriptor has been closed successfully.")
The code uses the os.open
function to open or create the file "File.txt"
with specified flags (os.O_CREAT | os.O_RDWR
).
It then immediately converts the resulting file descriptor to a file object using os.fdopen
. The with
statement ensures the file is automatically closed after the indented block.
The file object (fd
) writes the string "Hello, World!"
to the file.
Due to the with
statement, the file object is automatically closed at the end of the block, ensuring proper resource management.
A success message is printed to the console, confirming the closure of the file.
Output:
Note that the returned file descriptor from this method is non-inheritable.
Conclusion
The os.fdopen
method in Python, often overlooked in the plethora of file handling tools provided by the os
module, plays a pivotal role in seamlessly converting file descriptors (fd
) into file objects.
The method’s functionality is crucial for enhanced file manipulation, offering greater flexibility in handling files.
In the presented code examples, the method is employed to create, write to, and close files efficiently. The initial example demonstrates the basic usage of os.fdopen
to create a file, write content to it, and close the file object, showcasing the versatility of this method.
The second example extends the functionality by reading the file after its creation, emphasizing the bidirectional capabilities of the method. Additionally, the code emphasizes the importance of proper resource management by automatically closing the file object within a with
statement.
The final example combines os.fdopen
with os.open
within a with
statement, demonstrating a concise and effective approach to file handling with automatic closure of the file descriptor.
These examples collectively underscore the utility of os.fdopen
for Python developers seeking a robust and streamlined method for file operations, enriching their toolkit for efficient file handling.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn