Python os.error() Method
-
Syntax of Python
os.error()
Method -
Example 1: Use the
os.error()
Method in Python -
Example 2: Understanding the
os.error()
Method in Python
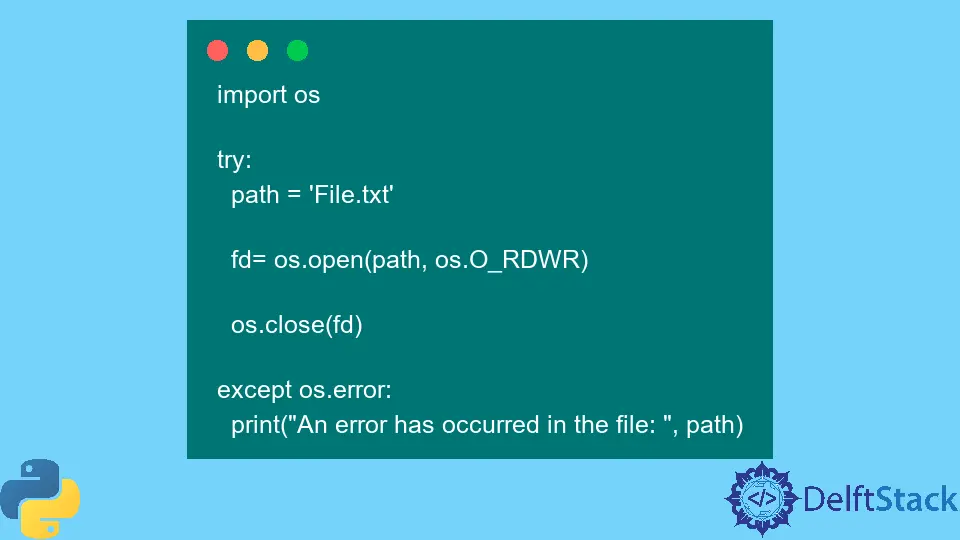
Python os.error()
method is an efficient way of finding an inaccessible or invalid file path. It is an alias of the OSError
exception.
Sometimes, all methods in the OS module might raise the os.error
exception.
Syntax of Python os.error()
Method
os.error()
Parameters
This method has no parameters.
Return
There is no return type for this method.
Example 1: Use the os.error()
Method in Python
import os
try:
path = "File.txt"
fd = os.open(path, os.O_RDWR)
os.close(fd)
except os.error:
print("An error has occurred in the file: ", path)
Output:
An error has occurred in the file: File.txt
The except
block is used in the above code to catch any os.error
exception.
Example 2: Understanding the os.error()
Method in Python
import os
try:
print(os.ttyname(7))
except os.error as error:
print(error)
print("The file descriptor is not linked with any terminal device.")
Output:
[Errno 9] Bad file descriptor
The file descriptor is not linked with any terminal device.
We can use the method os.error()
to find out the error in the code. For example, in the above code, the error is associated with a wrong file descriptor.
The method os.ttyname()
is used to get the terminal device of a specified file descriptor and raises an error if no match exists.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn