Python os.device_encoding() Method
-
Syntax of Python
os.device_encoding()
Method -
Example 1: Use the
os.device_encoding()
When Connected to a Terminal -
Example 2: Use the
os.device_encoding()
When Not Connected to a Terminal -
Example 3: Use the
os.device_encoding()
With a Pipe - Conclusion
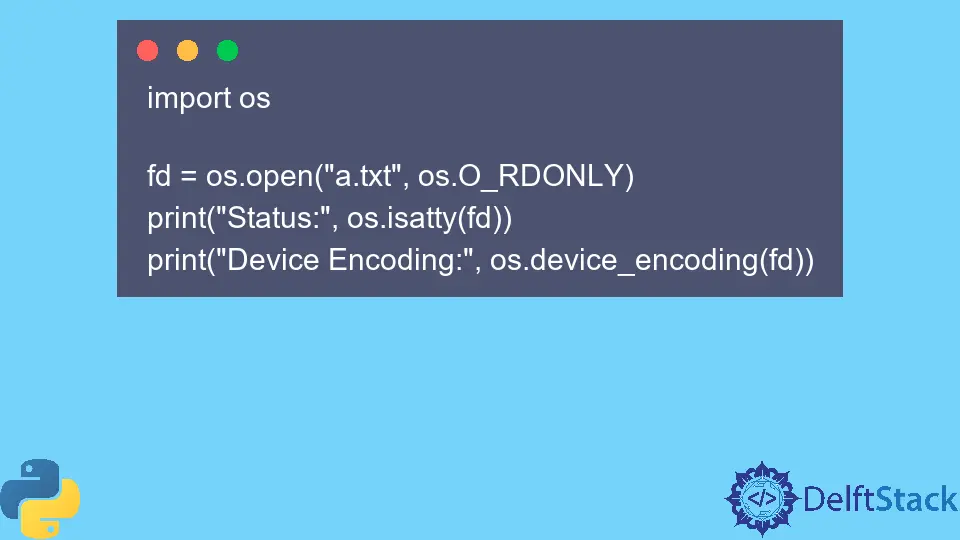
The device_encoding()
method belongs to the os
module. The os
module is a Python built-in module that offers various robust and simple utilities to interact with the operating system.
The device_encoding()
method returns the encoding of the device that is connected to the passed file descriptor. In this article, we’ll look at the different ways to use the os.device_encoding()
method.
However, it’s worth noting that this functionality is platform-specific and may not be available on all operating systems.
Syntax of Python os.device_encoding()
Method
os.device_encoding(fd)
Parameters
Parameter | Type | Description |
---|---|---|
fd |
integer | A file descriptor associated with a valid resource. |
Returns
The device_encoding()
method returns a string value. It returns the encoding of the device linked to the fd
file descriptor.
The device_encoding()
method returns a valid output only if fd
is connected to a terminal. Otherwise, it returns None
.
This means that connection with a terminal is mandatory to get a valid encoding.
Example 1: Use the os.device_encoding()
When Connected to a Terminal
In this code, we import the os
module. Then, we create a pseudo-terminal pair, which essentially simulates a terminal for our program to interact with.
This is done using the os.openpty()
function, which returns two file descriptors: master
and slave
. The master
file descriptor is used to control the terminal, while the slave
file descriptor simulates the terminal itself.
Next, we print the status of the master
file descriptor’s connection to a terminal using os.isatty(master)
. The os.isatty()
function checks whether the file descriptor is associated with a terminal, and in our case, it returns True
because master
is indeed connected to a terminal.
After that, we proceed to determine the device encoding of the connected terminal. We use the os.device_encoding(master)
function to retrieve this information, and it returns the encoding used by the terminal, which, in this case, is "UTF-8"
.
import os
master, slave = os.openpty()
print("Status:", os.isatty(master))
print("Device Encoding:", os.device_encoding(master))
Output:
Status: True
Device Encoding: UTF-8
The output shows that the status of the master
file descriptor is True
, indicating that it’s connected to a terminal. Additionally, the device encoding is revealed as "UTF-8"
, signifying the character encoding used by the connected terminal.
Note that this feature is specific to UNIX-based systems. Hence, it will not work on the Windows operating system.
Example 2: Use the os.device_encoding()
When Not Connected to a Terminal
In this example, we use the isatty()
method to check the terminal’s status. This method returns True
if a file descriptor is connected to a terminal.
In this code, we import the os
module. Then, we open a file named "a.txt"
using the os.open()
function with the os.O_RDONLY
flag, which indicates that we want to open the file for reading.
Once the file is opened, we check the status of the file descriptor, which is stored in the variable fd
. We use the os.isatty(fd)
function to see if the file descriptor is linked with a terminal, and in this case, the result is False
because "a.txt"
is not a terminal but a regular text file.
Following that, we attempt to retrieve the device encoding of the file descriptor using os.device_encoding(fd)
. However, since the file descriptor is not connected to a terminal (as indicated by the "False"
status), the os.device_encoding()
function returns "None"
.
This signifies that no specific device encoding is associated with this file descriptor because it is not connected to a terminal.
import os
fd = os.open("a.txt", os.O_RDONLY)
print("Status:", os.isatty(fd))
print("Device Encoding:", os.device_encoding(fd))
Output:
Status: False
Device Encoding: None
The output indicates that the status of the file descriptor fd
is "False"
, indicating that it is not connected to a terminal. Consequently, the device encoding is reported as "None"
since device encoding is only applicable when the file descriptor is associated with a terminal, and in this case, it is not.
Example 3: Use the os.device_encoding()
With a Pipe
In this code, we’re using the Python os
module to create a pipe, a mechanism for interprocess communication.
First, we create a pipe using the os.pipe()
function, which returns two file descriptors, read_fd
and write_fd
. The read_fd
is intended for reading data from the pipe, and the write_fd
is meant for writing data into the pipe.
Next, we use the os.isatty()
function to determine the status of each file descriptor. We check if each of them is associated with a terminal.
In both cases, the status is "False"
, which means that neither the read_fd
nor the write_fd
is connected to a terminal. This is expected because pipes are typically used for interprocess communication and are not associated with a terminal.
Following the status check, we use the os.device_encoding()
method to obtain the device encoding for each file descriptor. Since neither read_fd
nor write_fd
is connected to a terminal, the os.device_encoding()
function returns "None"
for both of them.
This result signifies that device encoding is not applicable to file descriptors associated with pipes, as pipes are not terminals and do not have an associated encoding.
import os
read_fd, write_fd = os.pipe()
print("Status (read_fd):", os.isatty(read_fd))
print("Device Encoding (read_fd):", os.device_encoding(read_fd))
print("Status (write_fd):", os.isatty(write_fd))
print("Device Encoding (write_fd):", os.device_encoding(write_fd))
Output:
Status (read_fd): False
Device Encoding (read_fd): None
Status (write_fd): False
Device Encoding (write_fd): None
The output demonstrates that neither read_fd
nor write_fd
is connected to a terminal, as indicated by the "False"
status. Additionally, the device encoding is reported as "None"
for both file descriptors, confirming that device encoding is not relevant in the context of pipes, which are designed for interprocess communication rather than terminal interactions.
Conclusion
The os.device_encoding()
method is a specialized utility in Python’s os
module that is primarily useful for identifying the encoding of devices connected to file descriptors, particularly in terminal-based applications. Its results depend on the presence of a terminal connection, making it less relevant for non-terminal file descriptors, such as regular text files, where it returns "None"
.
For interprocess communication through pipes, it consistently returns "None"
, highlighting its limited applicability outside of terminal-based scenarios. Note that the os.device_encoding()
method may not be suitable for broader use cases, and its use should be considered carefully depending on the specific needs of the application.