Python os.closerange() Method
- Understanding File Descriptors
-
the
os.closerange()
Method in Python -
Syntax of the Python
os.closerange()
Method -
Example 1: Use the
os.closerange()
Method to Close a Range of Consecutive File Descriptors in Python -
Example 2: Use the
os.closerange()
Method to Close Specific File Descriptors From a List in Python -
Example 3: Use the
os.closerange()
Method to Close All File Descriptors Above a Certain Threshold in Python - Use Cases
- Conclusion
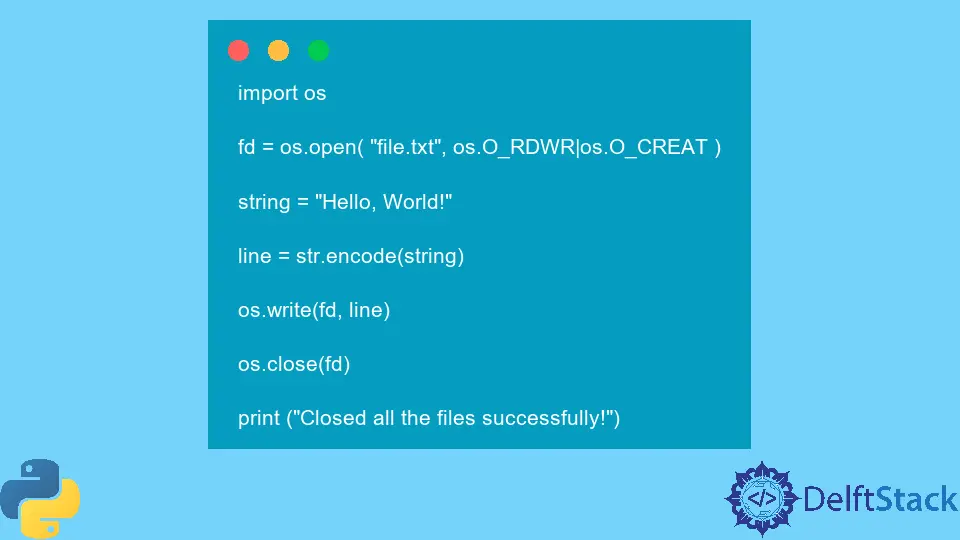
The os.closerange()
method in Python is a powerful tool for managing file descriptors. It allows you to close a range of file descriptors, which are unique identifiers associated with open files or resources in an operating system.
Python’s os.closerange()
method is an efficient way of closing all file descriptors from fd_low
(inclusive) to fd_high
(exclusive). Any errors from fd_low
to fd_high
while closing file descriptors are ignored.
This method is particularly useful when you want to close multiple file descriptors at once, which can improve efficiency and prevent resource leaks.
Understanding File Descriptors
In Unix-like operating systems, everything is treated as a file, including regular files, directories, sockets, and more. Each of these entities is uniquely identified by a non-negative integer known as a file descriptor.
The operating system uses file descriptors to keep track of open files and other resources. They serve as a reference to the actual file or resource in the underlying file system.
When you open a file or create a new socket, the operating system assigns a file descriptor to it.
the os.closerange()
Method in Python
The os.closerange()
method is part of the os
module in Python. It allows you to close a range of file descriptors, effectively releasing associated resources.
Syntax of the Python os.closerange()
Method
os.closerange(fd_low, fd_high)
Parameters
fd_low |
This is the lowest file descriptor in the range to be closed. |
fd_high |
This is the highest file descriptor in the range to be closed. |
Return
In the execution process, this method does not return any value.
Example 1: Use the os.closerange()
Method to Close a Range of Consecutive File Descriptors in Python
The simplest and most straightforward use of os.closerange()
is to close a range of consecutive file descriptors. This method is suitable when you have a continuous sequence of file descriptors that need to be closed.
Example Code:
import os
# Open a range of files (file descriptors will be assigned)
files = [os.open(f"file{i}.txt", os.O_CREAT | os.O_RDWR) for i in range(5)]
# Close file descriptors in the range [2, 4]
os.closerange(2, 5)
# Attempting to use closed file descriptors will raise an OSError
for file_descriptor in files:
try:
os.write(file_descriptor, b"Hello")
except OSError as e:
print(f"Error: {e}")
The example code attempts to open five files (file0.txt
to file4.txt
) and then closes the file descriptors in the range from 2
to 4
(inclusive). Finally, it attempts to write the bytes "Hello"
to each file descriptor.
However, since file descriptors 2
, 3
, and 4
have been closed using os.closerange()
, attempting to write to them will result in an OSError
.
The output of the code will be:
Error: [Errno 9] Bad file descriptor
Error: [Errno 9] Bad file descriptor
Error: [Errno 9] Bad file descriptor
Example 2: Use the os.closerange()
Method to Close Specific File Descriptors From a List in Python
Often, you may want to selectively close specific file descriptors from a list while keeping others open. This is where os.closerange()
becomes valuable.
By specifying the range of file descriptors you want to close, you can efficiently manage your I/O resources.
Example Code:
import os
# Open a range of files (file descriptors will be assigned)
files = [os.open(f"file{i}.txt", os.O_CREAT | os.O_RDWR) for i in range(5)]
# Define a list of file descriptors to close
to_close = [files[1], files[3]]
# Close specific file descriptors
os.closerange(min(to_close), max(to_close))
# Attempting to use closed file descriptors will raise an OSError
for file_descriptor in files:
try:
os.write(file_descriptor, b"Hello")
except OSError as e:
print(f"Error: {e}")
In this example, we open a range of files and store their file descriptors in the files
list. We then define a list to_close
containing the file descriptors we want to close (in this case, file descriptors 1
and 3
).
The os.closerange()
method is then used to efficiently close these file descriptors.
Output:
Error: [Errno 9] Bad file descriptor
Error: [Errno 9] Bad file descriptor
Example 3: Use the os.closerange()
Method to Close All File Descriptors Above a Certain Threshold in Python
In some scenarios, you may want to close all file descriptors above a certain threshold. This can be useful for managing a large number of open files.
Example Code:
import os
# Open a range of files (file descriptors will be assigned)
files = [os.open(f"file{i}.txt", os.O_CREAT | os.O_RDWR) for i in range(10)]
# Define a threshold (e.g., close all file descriptors above 6)
threshold = 6
# Close file descriptors above the threshold
os.closerange(threshold + 1, len(files))
# Attempting to use closed file descriptors will raise an OSError
for file_descriptor in files:
try:
os.write(file_descriptor, b"Hello")
except OSError as e:
print(f"Error: {e}")
In this code example, we first import the os
module. We create a list called files
containing file descriptors generated by opening files named file0.txt
to file9.txt
.
Then, we define a threshold
(in this case, 6
), above which we want to close all file descriptors. We call os.closerange(threshold + 1, len(files))
to close all file descriptors from threshold + 1
to the end of the list.
Finally, we attempt to use the closed file descriptors in the loop, which will raise an OSError
.
The output of the code will be:
Error: [Errno 9] Bad file descriptor
Error: [Errno 9] Bad file descriptor
Error: [Errno 9] Bad file descriptor
Use Cases
The os.closerange()
method is particularly useful in scenarios where you have opened multiple files or resources and want to efficiently close a range of them. This can be crucial for preventing resource leaks and managing system resources effectively.
Some common use cases include:
- Handling a large number of open files in a server application.
- Managing network sockets in a multi-client server.
- Dealing with file descriptors in low-level system programming.
Conclusion
The os.closerange()
method in Python provides a powerful tool for efficiently managing file descriptors. By understanding the different methods of using this function, you can effectively release associated resources, prevent resource leaks, and optimize the performance of your applications.
Whether you need to close a range of consecutive file descriptors, selectively close specific ones, or close those above a certain threshold, os.closerange()
offers versatile options for efficient resource management in your Python programs.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn