Python os.close() Method
-
Syntax of
os.close()
: -
Example Codes: Create a File Descriptor and Close Using the
os.close()
Method -
Example Codes: Automatically Close the File Descriptor Using the
os.close()
Method and thewith
Statement -
Example Codes: Close Multiple File Descriptors Using the
os.closerange()
Method -
Example Codes: Possible Errors While Using the
os.close()
Method - Conclusion
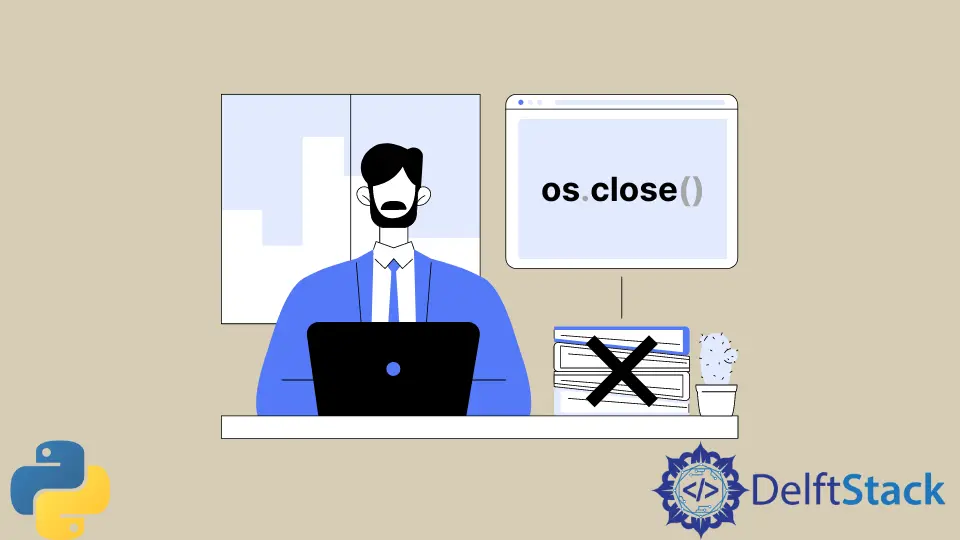
The os.close()
method is used to close a file descriptor. This method is a part of the os
module.
Syntax of os.close()
:
os.close(fd)
Parameter
fd |
This is the file descriptor to be closed. |
Return
This method does not return any value.
Example Codes: Create a File Descriptor and Close Using the os.close()
Method
The os.close()
method in Python is used to close a file descriptor. A file descriptor is a low-level integer representing an open file in the operating system.
The file descriptor could be obtained by functions like os.open()
or certain file-related system calls.
sample.txt
:
Hello, world
In the following example, we will create a file descriptor and then close it using the os.close()
method:
import os
import time
# Create a file descriptor
fd = os.open("sample.txt", os.O_RDWR)
# Print the file descriptor value before closing
print("File Descriptor before closing...")
# Pause
time.sleep(3)
# Close the file descriptor
os.close(fd)
# Print the file descriptor value after closing
print("File Descriptor after closing...")
In the code snippet above, it opens the file "sample.txt"
in read and write mode (os.O_RDWR
) and assigns the resulting file descriptor to the variable fd
.
To visually see the process, the code prints a message File Descriptor before closing...
and will pause for three seconds using the time.sleep(3)
function. Then, it will print File Descriptor after closing...
to see the file descriptor being closed.
Output:
File Descriptor before closing...
File Descriptor after closing...
It printed the second line after a three-second pause. It indicates that the file descriptor has been closed.
Example Codes: Automatically Close the File Descriptor Using the os.close()
Method and the with
Statement
The os.close()
method and the with
statement can be used together to ensure proper handling of file descriptors and to guarantee that they are closed when they are no longer needed.
The with
statement is particularly useful because it automatically takes care of resource management, including closing files.
sample.txt
:
Hello, world
In the following example, we will use the with
statement to close the file descriptor automatically:
import os
# Open a file object in read-write mode
with open("sample.txt", "r+") as fd:
# Write to the file
fd.write("Hello, world!")
# Move the file pointer to the start of the file
fd.seek(0)
# Read from the file
print(fd.read())
The code snippet above opens the file named "sample.txt"
in read-write mode ("r+"
) and creates a file object referred to by the variable fd
. The with
statement ensures that the file is properly closed after the indented block.
Using the fd.write()
function, it will write the string to the opened file object.
Then, the read()
method is used to read the contents of the file from the current file pointer position until the end of the file.
The contents are then printed to the console. The file is automatically closed when the with
block is exited.
Output:
Hello, world!
Hello, world!
was shown as the output since it was the recently written string to the file.
Example Codes: Close Multiple File Descriptors Using the os.closerange()
Method
The os.closerange()
method is used to close a range of file descriptors in Python.
This method takes two arguments, start
and end
, which specify the inclusive range of file descriptors to close.
sample1.txt
:
Hello, world
sample2.txt
:
Hello, world 2
We can also close multiple file descriptors at once using the os.closerange()
method:
import os
# Open two file descriptors
fd1 = os.open("sample1.txt", os.O_RDWR)
fd2 = os.open("sample2.txt", os.O_RDWR)
# Close both file descriptors
os.closerange(fd1, fd2)
print("Two file descriptors have been closed.")
This code opens two files, "sample1.txt"
and "sample2.txt"
, using os.open()
with read-write access (os.O_RDWR
). The resulting file descriptors are assigned to variables fd1
and fd2
.
The os.closerange(start, end)
function is used to close a range of file descriptors.
In this case, it closes all file descriptors from fd1
to fd2
inclusive. Both fd1
and fd2
will be closed.
Output:
Two file descriptors have been closed.
It printed the Two file descriptors have been closed.
to indicate that the file descriptor has been closed.
Example Codes: Possible Errors While Using the os.close()
Method
Some of the possible errors that can occur while using the os.close()
method are:
EBADF
- This error occurs when the file descriptor is not valid.EINTR
- This error occurs when the close operation is interrupted by a signal.
EBADF
Error
sample.txt
:
Hello, world
In the following example, we will try to close a file descriptor that is not valid:
import os
# Create a file descriptor
fd = os.open("sample.txt", os.O_RDWR)
# Try to close an invalid file descriptor
os.close(fd + 1)
Output:
Traceback (most recent call last):
File "sample.py", line 6, in <module>
os.close(fd + 1)
OSError: [Errno 9] Bad file descriptor
EINTR
Error
sample.txt
:
Hello, world
In the following example, we will try to close a file descriptor that is interrupted by a signal:
import os
import signal
# Create a file descriptor
fd = os.open("sample.txt", os.O_RDWR)
# Interrupt the close operation with a signal
os.kill(os.getpid(), signal.SIGINT)
# Try to close the file descriptor
os.close(fd)
Output:
Traceback (most recent call last):
File "sample.py", line 8, in <module>
os.close(fd)
OSError: [Errno 4] Interrupted system call
Conclusion
In conclusion, the os.close()
method is a crucial tool for managing file descriptors in Python.
This method ensures proper resource management, whether closing a single file descriptor obtained through os.open()
or handling multiple descriptors with os.closerange()
.
Additionally, combining os.close()
with the with
statement simplifies file handling, automatically closing files when the code block is exited.
It’s essential to use these methods judiciously, considering potential errors like EBADF
or EINTR
.
Overall, mastering these techniques empowers developers to efficiently and safely work with file descriptors in Python applications.