Python os.abort() Method
-
Syntax of
os.abort()
Method in Python -
Example Code 2: Use a Conditional Statement With the
os.abort()
Method - Conclusion
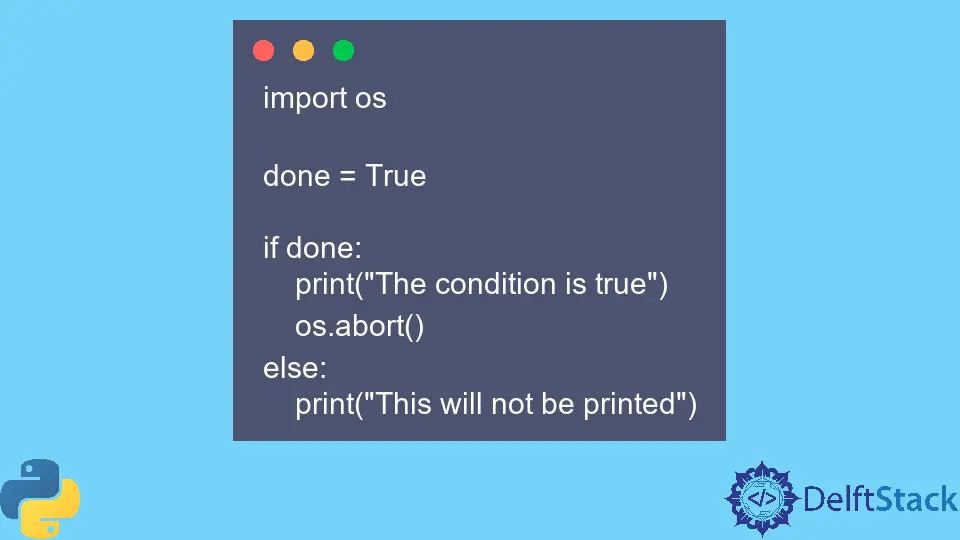
The os.abort()
method in Python is a function from the os
module that is used to generate a signal abort or SIGABRT
signal to the current process. This method is used to terminate a running process immediately, with different behaviors depending on the operating system:
- On Unix-like systems,
os.abort()
produces a core dump. - On Windows-based systems,
os.abort()
returns an exit code of3
.
Syntax of os.abort()
Method in Python
os.abort()
Parameters
The os.abort()
has no parameters.
Return
In the execution process, the method does not return any value.
Example Code 1: Use the os.abort()
Method
The os.abort()
method in Python is a function provided by the os
module. When invoked, this method initiates the generation of a process abort signal, specifically the SIGABRT
signal.
The purpose of calling os.abort()
is to forcefully terminate the current Python process. Unlike a regular program termination, which allows for cleanup and finalization routines to be executed, the use of os.abort()
results in an immediate and ungraceful exit.
This method is typically employed in scenarios where a critical and unrecoverable error occurs, and abrupt termination of the program is deemed necessary. It’s important to exercise caution when using os.abort()
, as it does not provide an opportunity for orderly shutdown procedures or resource cleanup.
Here’s a complete working code example that demonstrates the use of os.abort()
in Python:
import os
print("Hello, World!")
# Generate a SIGABRT signal to terminate the current process
os.abort()
# This line will not be executed
print("This will not be printed")
In this example, we start by importing the os
module. This module provides functions for interacting with the operating system and is part of Python’s standard utility modules.
The Hello, World!
string is then printed to the console.
Next, we generate a SIGABRT
signal to terminate the current process using os.abort()
. This method is called without any arguments and does not return any value.
After os.abort()
is executed, the process will be terminated, and the remaining code will not be executed. As such, the This will not be printed
string is not printed to the console.
Output:
Hello, World!
Example Code 2: Use a Conditional Statement With the os.abort()
Method
Using a conditional statement with the os.abort()
method involves incorporating the os.abort()
call within the control flow of an if
or while
statement. This approach allows the program to conditionally trigger the os.abort()
method based on certain criteria or conditions being met.
The decision to call os.abort()
within a conditional statement is often tied to specific error scenarios or exceptional conditions that warrant an immediate and forceful termination of the program. Care should be taken when employing this approach, ensuring that the conditions for invoking os.abort()
are well-defined and align with the desired behavior for terminating the program under exceptional circumstances.
As with using os.abort()
independently, using it within a conditional statement lacks the graceful cleanup typically associated with regular program termination.
In this example, we implement an infinite loop by setting the Boolean value of done
to True
. The os.abort()
can terminate the program and stop the loop as long as the interpreter processes it.
import os
done = True
if done:
print("The condition is true")
os.abort()
else:
print("This will not be printed")
This Python code snippet uses the os.abort()
method within a conditional statement.
The condition done
is set to True
, so the code enters the if
block. Inside the block, it prints a message (The condition is true
) and then calls os.abort()
, forcefully terminating the program.
The else
block is present but won’t be executed since os.abort()
ends the program abruptly, preventing further execution. The primary purpose is to demonstrate the immediate termination of the program when the specified condition is met.
Output:
The condition is true
Conclusion
The os.abort()
method in Python, part of the os
module, is a powerful tool for generating a SIGABRT
signal to terminate the current process. It serves as a mechanism for immediate and ungraceful program termination, with its behavior varying across operating systems.
On Unix-like systems, it produces a core dump, while on Windows-based systems, it returns an exit code of 3
. The method has a simple syntax with no parameters and does not return any value during execution.
Developers should exercise caution when using os.abort()
, ensuring that its application aligns with the desired behavior under exceptional circumstances. The examples provided demonstrate its use both independently and within a conditional statement.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn