Pandas DataFrame.rolling() Function
Minahil Noor
Jan 30, 2023
-
Syntax of
pandas.DataFrame.rolling()
: -
Example Codes:
DataFrame.rolling()
Method to Find the Rolling Sum With a Window of Size 2 -
Example Codes:
DataFrame.rolling()
Method to Find the Rolling Mean With a Window of Size 3
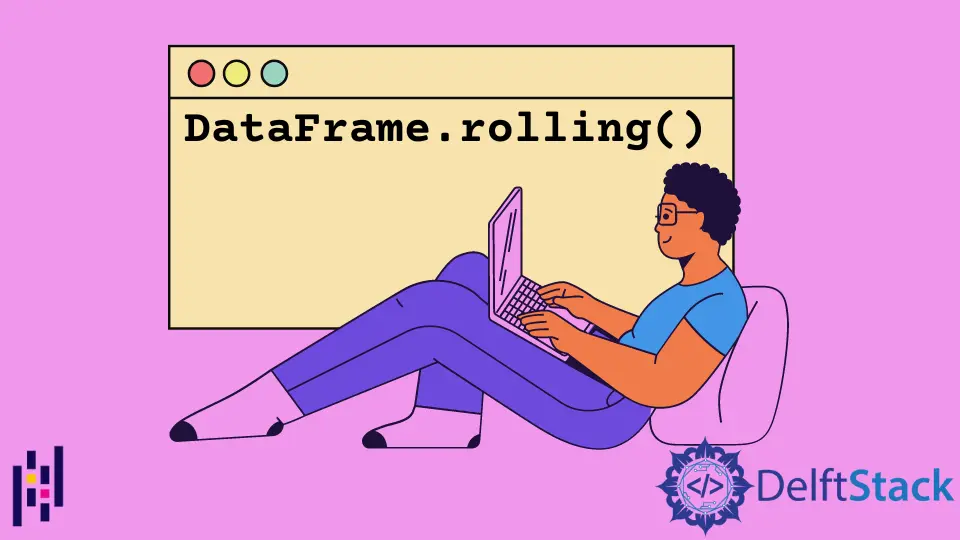
Python Pandas DataFrame.rolling()
function provides a rolling window for mathematical operations.
Syntax of pandas.DataFrame.rolling()
:
DataFrame.rolling(
window, min_periods=None, center=False, win_type=None, on=None, axis=0, closed=None
)
Parameters
window |
It is an integer, offset, or BaseIndexer subclass type parameter. It specifies the size of the window. Each window has a fixed size. This parameter specifies the number of observations used for calculating the statistic. |
min_periods |
It is an integer parameter. This parameter specifies the minimum number of observations in a window. The number of observations should have a value; otherwise, the result is a null value. |
center |
It is a Boolean parameter. It specifies setting the labels at the center of the window. |
win_type |
It is a string parameter. It specifies the type of window. To read further click here. |
on |
It is a string parameter. It specifies the column name on which to calculate the rolling window rather than the index. |
axis |
It is an integer or string parameter. |
closed |
It is a string parameter. It specifies the interval closure. It has four options: right, left, both, or neither. |
Return
It returns a window after performing the particular operation.
Example Codes: DataFrame.rolling()
Method to Find the Rolling Sum With a Window of Size 2
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.rolling(2).sum()
print("The Rolling Window After Calculation is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Attendance Obtained Marks
0 60 90
1 100 75
2 80 82
3 78 64
4 95 45
The Rolling Window After Calculation is:
Attendance Obtained Marks
0 NaN NaN
1 160.0 165.0
2 180.0 157.0
3 158.0 146.0
4 173.0 109.0
The function has returned the rolling sum over the index axis. Note that for the index 0, the function has returned NaN
because of the size of the rolling window.
Example Codes: DataFrame.rolling()
Method to Find the Rolling Mean With a Window of Size 3
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.rolling(3).mean()
print("The Rolling Window After Calculation is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Attendance Obtained Marks
0 60 90
1 100 75
2 80 82
3 78 64
4 95 45
The Rolling Window After Calculation is:
Attendance Obtained Marks
0 NaN NaN
1 NaN NaN
2 80.000000 82.333333
3 86.000000 73.666667
4 84.333333 63.666667
The function has returned the rolling mean window.