How to Merge Two Data Frames With Different Number of Rows in R
-
Use the
full_join
Function to Merge Two R Data Frames With Different Number of Rows -
Use the
left_join
Function to Merge Two R Data Frames With Different Number of Rows -
Use the
right_join
Function to Merge Two R Data Frames With Different Number of Rows
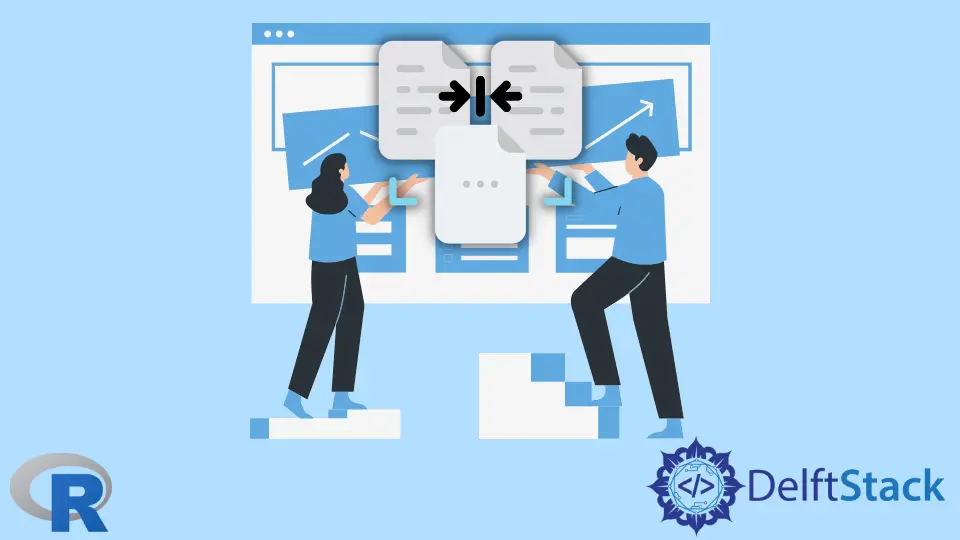
This article will demonstrate multiple methods of merging two data frames with a different number of rows in R.
Use the full_join
Function to Merge Two R Data Frames With Different Number of Rows
full_join
is part of the dplyr
package, and it can be used to merge two data frames with a different number of rows. The function takes data frames to be merged as the first two arguments and returns the same type of object as the first argument. This function can operate on data frame extension types like tibble
or lazy data frames. full_join
extracts all rows and columns from both data frame arguments. It fills elements with NA
-s when there are no matching values.
library(dplyr)
v1 <- c(1.1, 1.2, 1.3, 2.1, 2.2, 2.3)
v2 <- c(11, 12, 13, 21, 22, 23)
df1 <- data.frame(v1, v2)
v1 <- c(9.1, 9.2, 9.3, 9.1, 9.2, 9.3, 9.3, 9.2, 9.1)
v2 <- c(101, 102, 103, 201, 202, 203, 204, 403, 404)
wday <- factor(c("Wed", "Thu", "Mon", "Wed", "Thu", "Fri", "Mon", "Tue", "Wed"))
df2 <- data.frame(v1, v2, wday)
dff <- df1 %>% full_join(df2)
dff
v1 v2 wday
1 1.1 11 <NA>
2 1.2 12 <NA>
3 1.3 13 <NA>
4 2.1 21 <NA>
5 2.2 22 <NA>
6 2.3 23 <NA>
7 9.1 101 Wed
8 9.2 102 Thu
9 9.3 103 Mon
10 9.1 201 Wed
11 9.2 202 Thu
12 9.3 203 Fri
13 9.3 204 Mon
14 9.2 403 Tue
15 9.1 404 Wed
Use the left_join
Function to Merge Two R Data Frames With Different Number of Rows
left_join
is another method from the dplyr
package. It takes arguments similar to the full_join
function, but left_join
extracts all rows from the first data frame and all columns from both of them.
library(dplyr)
v1 <- c(1.1, 1.2, 1.3, 2.1, 2.2, 2.3)
v2 <- c(11, 12, 13, 21, 22, 23)
df1 <- data.frame(v1, v2)
v1 <- c(9.1, 9.2, 9.3, 9.1, 9.2, 9.3, 9.3, 9.2, 9.1)
v2 <- c(101, 102, 103, 201, 202, 203, 204, 403, 404)
wday <- factor(c("Wed", "Thu", "Mon", "Wed", "Thu", "Fri", "Mon", "Tue", "Wed"))
df2 <- data.frame(v1, v2, wday)
dfl <- df1 %>% left_join(df2)
dfl
Output:
v1 v2 wday
1 1.1 11 <NA>
2 1.2 12 <NA>
3 1.3 13 <NA>
4 2.1 21 <NA>
5 2.2 22 <NA>
6 2.3 23 <NA>
Use the right_join
Function to Merge Two R Data Frames With Different Number of Rows
right_join
works similar to the left_join
function except extracting all rows from the second data frame argument rather than the first. The function also copies all columns from both data frames to a newly constructed object.
library(dplyr)
v1 <- c(1.1, 1.2, 1.3, 2.1, 2.2, 2.3)
v2 <- c(11, 12, 13, 21, 22, 23)
df1 <- data.frame(v1, v2)
v1 <- c(9.1, 9.2, 9.3, 9.1, 9.2, 9.3, 9.3, 9.2, 9.1)
v2 <- c(101, 102, 103, 201, 202, 203, 204, 403, 404)
wday <- factor(c("Wed", "Thu", "Mon", "Wed", "Thu", "Fri", "Mon", "Tue", "Wed"))
df2 <- data.frame(v1, v2, wday)
dfr <- df1 %>% right_join(df2)
dfr
Output:
v1 v2 wday
1 9.1 101 Wed
2 9.2 102 Thu
3 9.3 103 Mon
4 9.1 201 Wed
5 9.2 202 Thu
6 9.3 203 Fri
7 9.3 204 Mon
8 9.2 403 Tue
9 9.1 404 Wed
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook