How to Move Files From One Directory to Another Using Python
-
Use the
shutil.move()
Function to Move Files in Python -
Use the
os.rename()
oros.replace()
Functions to Move Files in Python -
Use the
pathlib
Module to Move Files in Python
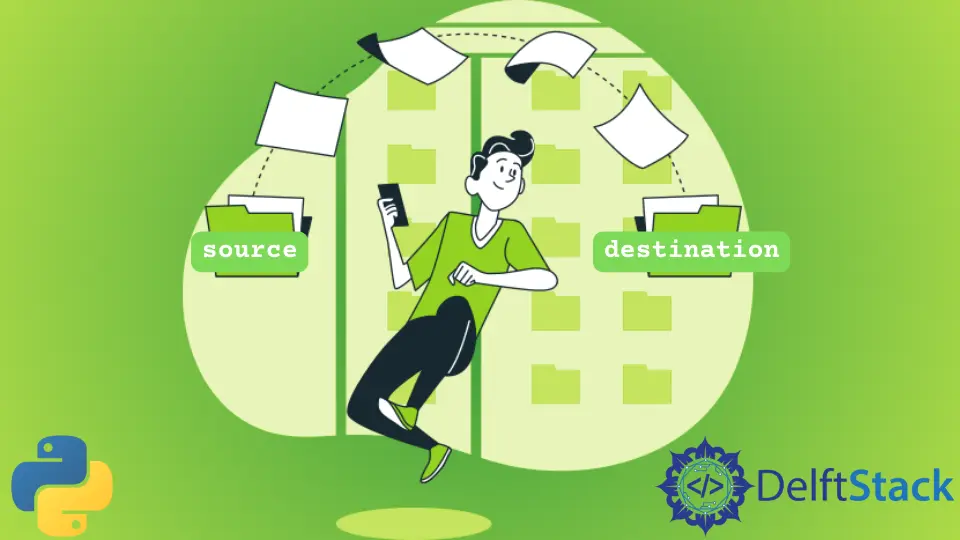
Moving files from one directory to another may sound not so big of a deal, but at times, it helps a lot in manipulating files.
This tutorial will introduce some ways to move files from one directory to another in Python.
Use the shutil.move()
Function to Move Files in Python
The shutil
module is a Python module that helps in high-level manipulations on files or a set of files. This module comes into play in operations like copying a file from somewhere or removing a file.
For moving a file from one directory to another directory with the help of the shutil
module, shutil.move()
is called.
Example:
import shutil
import os
file_source = "Path/Of/Directory"
file_destination = "Path/Of/Directory"
get_files = os.listdir(file_source)
for g in get_files:
shutil.move(file_source + g, file_destination)
Here, the listdir()
function is used from the os
module, which is used to get the complete list of all the files present in the directory. We use a for
loop to move the file and note that the move()
function of the shutil
module is used to transfer the files from one directory to another.
Use the os.rename()
or os.replace()
Functions to Move Files in Python
Many a time, the user needs to connect to the main system through Python. In this case, the os
module comes into play. The os
module basically acts as a mediator between the user and the computer’s operating system so that the user can connect with the operating system properly.
One of the functions of this module is the rename()
module used to move files from one location to another. This function moves the files by renaming the directory name of those files.
Another function of this module is the replace()
function. This function helps in renaming the file or current directory. The destination must be a file and not a directory. So if the destination is a file, then it will be replaced without any error.
In conclusion, when the file’s final destination is in the same disk as where it is from, the rename()
function is used. And if the destination of the file has to be changed, then replace()
must be used.
Example:
import os
file_source = "Path/Of/Directory"
file_destination = "Path/Of/Directory"
get_files = os.listdir(file_source)
for g in get_files:
os.replace(file_source + g, file_destination + g)
Here also, we follow the same procedure by first defining the paths of the initial and the final directories. Then we use the listdir()
function to get the list of all the files in the current directory. After that, we use a for
loop to overwrite the destination of those files.
Use the pathlib
Module to Move Files in Python
The pathlib
module in Python is a standard module used to provide an object used to manipulate different files and dictionaries. The core object to work with files is called Path.
Example:
from pathlib import Path
import shutil
import os
file_source = "Path/Of/Directory"
file_destination = "Path/Of/Directory"
for file in Path(file_source).glob("randomfile.txt"):
shutil.move(os.path.join(file_source, file), file_destination)
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedInRelated Article - Python File
- How to Get All the Files of a Directory
- How to Append Text to a File in Python
- How to Check if a File Exists in Python
- How to Find Files With a Certain Extension Only in Python
- How to Read Specific Lines From a File in Python
- How to Check if File Is Empty in Python