How to Log to Stdout in Python
-
Log to
stdout
With thelogging.basicConfig()
Function in Python -
Log to
stdout
With thelogging.StreamHandler()
Function in Python - Conclusion
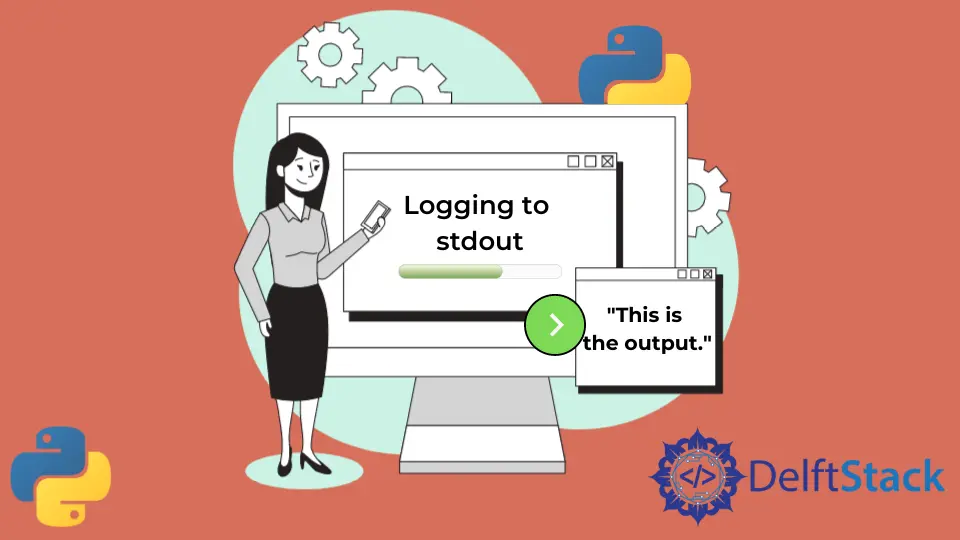
Logging is the process of recording events, actions, and status information within a program. It provides developers with a mechanism to track the execution of code, capture errors, and gain insights into the application’s runtime behavior.
This tutorial focuses on basicConfig()
—a fundamental method for quick logging configuration. Additionally, we’ll explore the power of StreamHandler()
combined with a logger object and setLevel()
.
Log to stdout
With the logging.basicConfig()
Function in Python
The basicConfig()
method in Python’s logging
module serves as a convenient and quick way to set up a basic configuration for logging. This method allows developers to establish fundamental parameters, such as the logging level, output format, and destination, without the need for extensive customization.
Syntax:
logging.basicConfig(**kwargs)
Parameters (kwargs
):
filename
: Specifies the file mode if afilename
is given (default is'a'
).filemode
: Iffilename
is given, the file mode to open it with (default is'a'
).format
: The format of the log message (default is'%(message)s'
).datefmt
: A string representing the date format (default isNone
).level
: The root logger level (default islogging.WARNING
).stream
: The stream to use for logging. If not specified,sys.stderr
is used.
Let’s start with a complete working example that demonstrates how to log messages to stdout
using basicConfig()
.
Example:
import logging
# Configure the root logger to log messages to stdout
logging.basicConfig(
level=logging.INFO, format="%(asctime)s - %(levelname)s - %(message)s"
)
# Log a sample message
logging.info("This is a log message to stdout.")
Now, let’s break down the code step by step:
Begin by importing the logging
module, which provides the necessary functionalities for logging in Python.
Then, we configure the root logger with basicConfig()
.The basicConfig()
method is a convenient way to configure the root logger.
In this example, the parameter level=logging.INFO
establishes the logging level, ensuring that messages with INFO level and above are captured. Simultaneously, the format='%(asctime)s - %(levelname)s - %(message)s'
parameter defines a comprehensive log message format, incorporating the timestamp, log level, and the content of the log message.
Finally, we log a sample message using the info
level. The configured basicConfig()
settings determine how this message will be formatted and where it will be output.
Output:
2023-11-29 12:34:56,789 - INFO - This is a log message to stdout.
This output illustrates the power of basicConfig()
in setting up a basic logging configuration. The timestamp, log level, and log message are clearly formatted, making it easy to understand and analyze the logged information.
Log to stdout
With the logging.StreamHandler()
Function in Python
The StreamHandler()
method in Python’s logging
module is a powerful tool for directing log messages to various output streams, commonly used for streaming messages to standard output (stdout
). This method provides developers with a flexible way to control the destination and formatting of log messages, enhancing the versatility of the logging framework.
Syntax:
handler = logging.StreamHandler(stream=None)
Parameters:
stream
: The stream to which the log messages will be written. If not specified, the default issys.stderr
.
Let’s dive into a complete working example that demonstrates how to use a Logger Object with StreamHandler()
to log messages to stdout
.
Example:
import logging
# Create a logger with a custom name
logger = logging.getLogger("my_logger")
# Set the logging level to INFO
logger.setLevel(logging.INFO)
# Create a StreamHandler and attach it to the logger
stream_handler = logging.StreamHandler()
logger.addHandler(stream_handler)
# Log a sample message
logger.info("This is a log message to stdout.")
Now, let’s break down the code step by step, explaining each part in detail. We start by importing the logging
module, the powerhouse for logging in Python.
Then, we create a logger object with a custom name, in this case, my_logger
. Logger names are hierarchical, allowing for better organization and categorization of log messages.
The setLevel()
is used to establish the logging level for the logger. In this case, it’s set to logging.INFO
, indicating that messages with INFO
level and above will be captured.
Here, we create a StreamHandler
object, a handler specifically designed for streaming log output to various destinations. We then add this handler to the logger using the addHandler()
method.
Finally, we log a sample message using the logger’s info
level. The StreamHandler
attached to the logger determines how this message will be handled and where it will be output.
Output:
This is a log message to stdout.
In this example, the message is logged to stdout
without additional formatting. The power lies in the ability to customize the logger and handler to suit specific needs, making it a versatile solution for a wide range of logging scenarios.
Conclusion
To recap, mastering basicConfig()
provides a swift avenue for establishing fundamental logging configurations. Its simplicity shines in setting logging levels, formats, and destinations, as demonstrated in the example.
On the other hand, StreamHandler()
enhances the logging arsenal by facilitating targeted streaming, exemplified through a logger object. Through the logging
module’s versatile tools, developers can configure and customize logging setups to suit specific needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn