How to Set Logging Levels Using setLevel() in Python
- Function of Logging in a Program in Python
- Levels of Log Messages in Python
-
Set Logging Levels Using the
setLevel()
Function in Python Logging Module - Logging Handlers in Python
- Conclusion
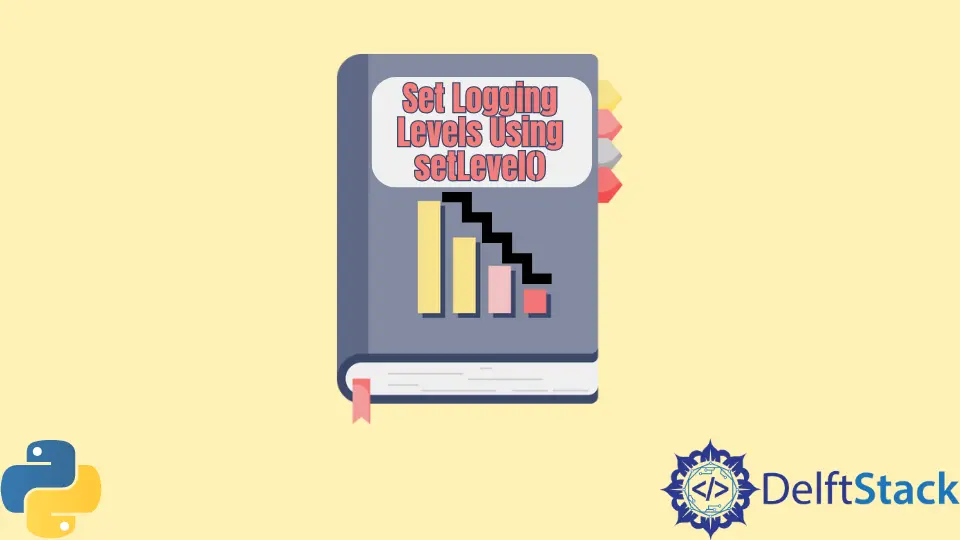
Logging is a very efficient tool in any programmer’s life. It not only makes us better understand the flow of the program but also the possibilities of errors that might occur during the program’s execution.
Python provides a separate logging
module as a part of its standard library to make logging easier. This article will discuss the logging setLevel
and how it works in Python.
Function of Logging in a Program in Python
Logging is a process to track down the flow of the program when the software runs. However, if you do not implement logging into your program, whenever the program crashes, it is difficult to find the root of the problem that has occurred.
With logging implemented in your program, you can easily find the root of the problem and resolve it in no time. It is very useful while debugging and developing software.
Sometimes, people use the print
statement to find the problems in the software. Printing can solve the issues for simple scripts, but they are not a good option for larger and more complex software.
Python provides a built-in module called logging
from its standard library that writes status messages to any output stream or a file about which part of the program is running and what problems have been caused.
Levels of Log Messages in Python
The logging
module in Python has different status/log message levels based on their importance. Therefore, the level of a log message tells you how important that log message is.
The different levels of the log messages are as follows: DEBUG
, INFO
, WARNING
, ERROR
, and CRITICAL
, where CRITICAL
has the most importance.
Log Level | Description |
---|---|
DEBUG |
It is used for debugging purposes in the software. Typically gives detailed information when a problem occurs. |
INFO |
It ensures that everything works fine in a program. |
WARNING |
It is used to tell that some problem might occur in the future and should be resolved to avoid future problems. |
ERROR |
It is used to signify that the software has not been able to perform a specific function due to a serious problem. |
CRITICAL |
This level signifies a serious problem that results in the stoppage of the software. |
All these levels are set for the handler or logger so that appropriate messages can be shown at appropriate times when the error occurs. The logging levels are explained above based on their importance, with the first being the least important and the last (CRITICAL
level) being the most important.
Set Logging Levels Using the setLevel()
Function in Python Logging Module
The setLevel(level)
function is used to set the threshold for a logger to the specified level. The logging messages less severe than the specified level are ignored, whereas the messages with higher severity are emitted by the corresponding handler that service the logger.
Logging in Python has a concept of an effective level. Initially, when a logger is created, it is set to a level NOTSET
.
However, this NOTSET
is not the effective level. The effective level is the one that has been set explicitly with the help of setLevel(level)
.
When a logger is created, if its level is not explicitly set, then its parent logger’s level is examined to get an effective level for the logger, which would have been explicitly set using the setLevel(level)
. If the parent logger has also not been set to an effective level, then its parent logger is checked.
The process continues until a level other than NOTSET
is found or the root is reached. The root logger is set with a default level of WARNING
; therefore, the root’s default level would be considered the effective level in such cases.
Now, let us see the usage of the setLevel(level)
using some code examples.
import logging
logging.debug("Debug message")
logging.info("Info message")
logging.warning("Warning message")
logging.error("Error message")
logging.critical("Critical message")
Output:
WARNING:root:Warning message
ERROR:root:Error message
CRITICAL:root:Critical message
As seen in the above output, only the WARNING
, ERROR
, and CRITICAL
messages are printed because the root logger’s default level WARNING
has been considered the effective level as no other logger or its parent is specified. Therefore, all messages from the level WARNING
and after are being printed, and the less important ones are ignored.
Let us see an example where the logger considers the effective level as the parent’s level.
import logging
parent_logger = logging.getLogger("parent")
parent_logger.setLevel(4)
child_logger = logging.getLogger("parent.child")
print(parent_logger.getEffectiveLevel())
print(child_logger.getEffectiveLevel())
Output:
4
4
As you can see, child_logger
has not been set to an effective level, so the level of parent_logger
is used as the effective level.
Logging Handlers in Python
Handlers in Python are objects responsible for logging appropriate log messages to the handler’s specified destination; these handlers also work like loggers. If a logger has no handler set, its ancestors are searched for a handler.
Let us now see the usage of handlers in logging:
import logging
logger = logging.getLogger("example")
logger.setLevel(logging.INFO)
fileHandler = logging.FileHandler("p1.log")
fileHandler.setLevel(logging.INFO)
chl = logging.StreamHandler()
chl.setLevel(logging.INFO)
logger.addHandler(fileHandler)
logger.addHandler(chl)
logger.info("Information")
Output:
Information
We created two handlers in the above code: fileHandler
and chl
. The fileHandler
sends the records to the p1.log
file, and the chl
handler sends the records to the stream.
However, if the stream is not specified, then sys.stderr
is used. In the end, the handlers are added to the logger using the addHandler
.
Now you must wonder why we have set the level twice: one for the logger and the other for the handlers. You can remove the setLevel()
on the handlers, which will leave all the level filtering of the messages to the logger.
However, if you set the level for the handlers and the logger, there is a different scenario. The logger is the first to filter the messages based on the level; therefore, if you set the logger to WARNING
, INFO
, or any higher levels and the handler to DEBUG
, you won’t receive any DEBUG
log message as the logger at the first place ignores it.
Similarly, if you set the logger to DEBUG
and the handlers to any higher level, such as INFO
, you also won’t receive any DEBUG
messages as the handlers will reject them. The handler rejects it even if the logger approves it (since INFO
> DEBUG
).
Therefore, one should be careful enough while setting the levels for the logger and handlers to ensure the proper working of the software.
Conclusion
In this article, we have discussed the logging setLevel()
and how it works in Python.
Logging is a very efficient tool to manage the code of the software or a program by logging the program’s flow and finding the possibilities of the error. For logging in Python, we set different levels for different log messages based on their importance.
All these levels are set using the setLevel
, which has been explained in great detail in this article.