How to Use Log4j With the Help of Logging Library in Python
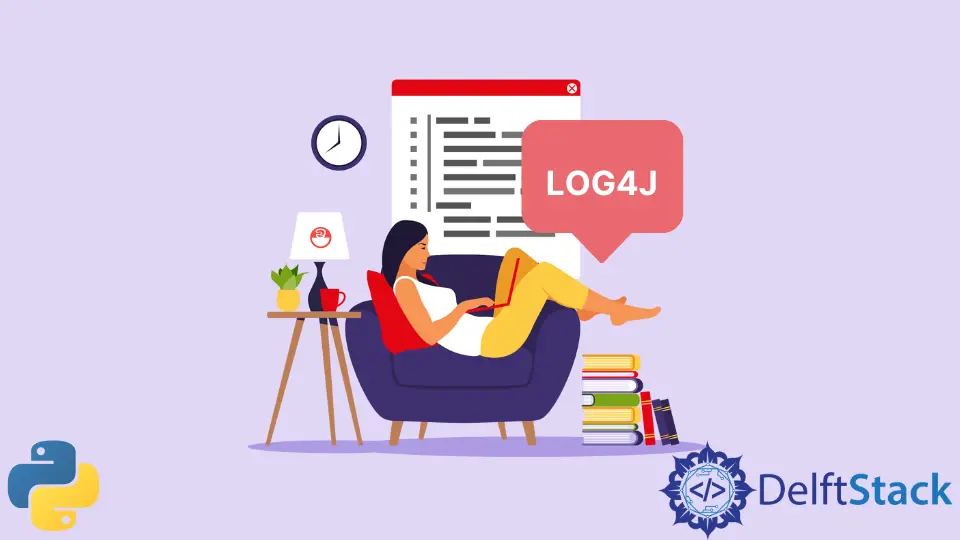
This article explains what is log4j
and, how it works and why we should use it. We’ll also see how to use log4j
inside the Python programming language with the help of the logging
library.
Overview of Log4j
and Its Importance to Use
The log4j
is a piece of software leveraged by programmers to help them when they are logging
data in their application. And, logging
data means keeping a diary of the activities or actions performed in the application.
We can use log4j
for security reasons, like looking at the various authentications. But, it could also be for keeping a record of things that have happened in the application for debugging purposes.
Or to generally know what the application has been doing. For example, the log4j
is a framework in the Java programming language that is a semi-build application.
Similarly, in Python, we used a logging
module instead of log4j
. logging
is a process of recording application actions and states to a secondary interface. In other words, write your program actions to a file, but how do you start logging?
Well, you need to get acquainted with logging
levels. Every logging
library allows you to lock information on a certain level. There are five primary logging levels that you must know.
Levels | Description |
---|---|
debug |
debug level is used during the development process or bug fixing and troubleshooting. All the developer-specific information goes under this level. |
info |
info level is used for logging any significant default operations of your program, like default user or system viewing actions. |
warning |
warning level is used for logging events that could potentially become an error in the long run. This logging level is supposed to help you track down errors. |
error |
error level is used for writing down errors and errors are mistakes that can affect the execution of the program in some wrong way. |
critical |
The critical level is doomsday; the program is dead or severely damaged. |
Use Log4j
With the Help of logging
Library in Python
Now we are just going to run a simple basic code, and there is no such logic, but we want to show you some understanding, like how you can write all the logs inside a file.
Let’s jump into the code and first configure the logging
system, call the basicConfig()
method, and pass in a file name using the filename
parameter. Python will write all the log messages to this file; if it does not exist, Python will create it.
The following parameter is filemode
, which means file mode means whether it is an append mode to write mode or whatever you put there, and by default, the file will be created in append mode. The next one is the format
that represents a lot of information such as asctime
, levelname
, and message
.
asctime
basically shows what kind of time it is printing inside this particular text file concerning the logs. The second parameter value is something called levelname
; this parameter shows us what kind of error occurred during the execution.
The message
is all the messages we are trying to print inside that log message. We are using datefmt
; this parameter will get printed the time in a specific order.
The basicConfig()
function has a different attribute, we can read about all attributes from here.
import logging
logging.basicConfig(
filename="demo.txt",
filemode="a",
format="%(asctime)s %(levelname)s-%(message)s",
datefmt="%Y-%m-%d %H:%M:%S",
)
Now we have written a simple logic where we compare the percentile with some numbers and append some logs inside the text file.
for i in range(0, 15):
if i % 2 == 0:
logging.error("Log error message")
elif i % 3 == 0:
logging.warning("Log warning message")
elif i % 5 == 0:
logging.debug("Log debug message")
elif i % 7 == 0:
logging.critical("Log critical message")
else:
logging.info("Log info message")
Output:
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 WARNING-Log warning message
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 CRITICAL-Log critical message
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 WARNING-Log warning message
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 ERROR-Log error message
2022-09-01 23:21:28 ERROR-Log error message
After running the program, we can notice the info
and debug
log are not added inside the text file because, by default, levelname
calls error
and error
levels do not show info
and debug
.
However, we can use other levels by using the level
parameter while passing logging.DEBUG
.
level = logging.DEBUG
Now, if we run and open the demo.txt
file, we will see all log messages, but if we update the log level to logging.ERROR
, we see the error
and critical
messages.
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 INFO-Log info message
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 WARNING-Log warning message
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 DEBUG-Log debug message
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 CRITICAL-Log critical message
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 WARNING-Log warning message
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 INFO-Log info message
2022-09-01 23:23:57 ERROR-Log error message
2022-09-01 23:23:57 INFO-Log info message
2022-09-01 23:23:57 ERROR-Log error message
Let’s take a common problem where we are going to divide any number by zero. To determine this operation, we will use the try
block.
If the operation gets failed, we will go inside the except
block and display the log error.
try:
1 / 0
except:
logging.error("Log zero division error occurs")
Output:
2022-09-02 00:29:48 ERROR-Log zero division error occurs
It is an essential part of a project because you probably have to write this kind of logging
mechanism whenever you are working on a project.
Complete Python Code:
import logging
logging.basicConfig(
filename="demo.txt",
filemode="w",
format="%(asctime)s %(levelname)s-%(message)s",
datefmt="%Y-%m-%d %H:%M:%S",
level=logging.DEBUG,
)
# for i in range(0,15):
# if i%2==0:
# logging.error('Log error message')
# elif i%3==0:
# logging.warning('Log warning message')
# elif i%5==0:
# logging.debug('Log debug message')
# elif i%7==0:
# logging.critical('Log critical message')
# else:
# logging.info('Log info message')
try:
1 / 0
except:
logging.error("Log zero division error occurs")
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn