How to Set Python Log Formatter
- What Are the Various Logging Levels in Python?
- What Is a Python Log Handler?
- What Is Python Log Formatter?
- Steps to Create a Python Log Formatter
- Conclusion
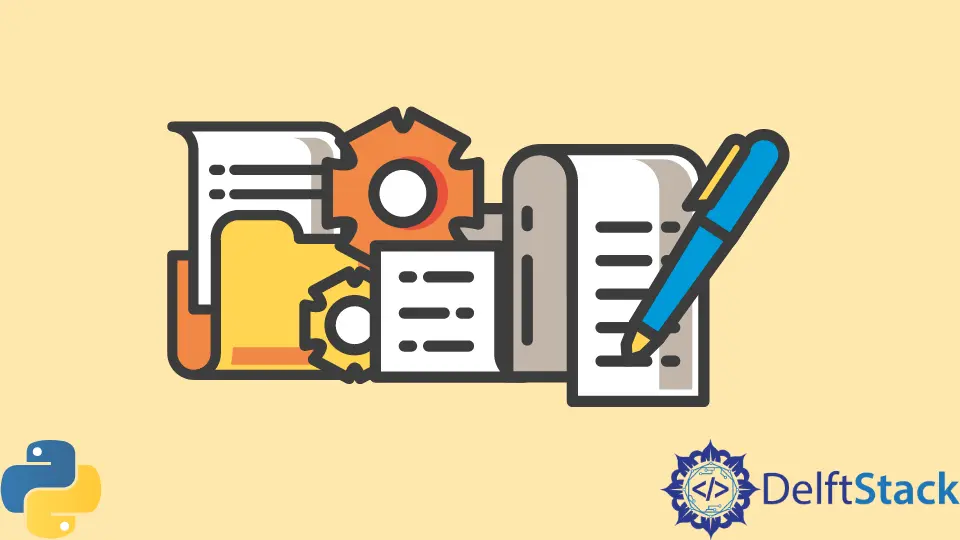
We use logging to store information about the execution of a program. When software runs, various warnings are raised, and sometimes errors occur. The data stored by logging helps us identify the causes of the error.
This article will discuss how we can use a log formatter in Python to store the log data in a desirable format.
What Are the Various Logging Levels in Python?
The log level is used to identify the severity of a warning or message in a log file. In Python, we have six logging levels: critical
, error
, warning
, info
, debug
, and notset
. The critical
level has the highest priority out of these, while the notset
level has the lowest priority. Usually, when we create logs in a program, the logs are printed to the standard output. We can print an error
log using the error()
method in the logging
module. As shown below, the error()
method takes a string message and prints it to the standard output.
import logging
logging.error("This is a sample error message")
Output:
ERROR:root:This is a sample error message
Similarly, you can print a warning
log using the warning()
method, debug
log using the debug()
method, critical
log using the critical()
method, and info
log using the info()
method as shown below.
import logging
logging.debug("This is a sample debug message")
logging.info("This is a sample info message")
logging.warning("This is a sample warning message")
logging.error("This is a sample error message")
logging.critical("This is a sample critical message")
Output:
WARNING:root:This is a sample warning message
ERROR:root:This is a sample error message
CRITICAL:root:This is a sample critical message
In the output, root
is the name of the current logger. We can also define loggers using the getLogger()
method. Also, you can observe that only the critical
, error
, and warning
logs are printed while the debug
and the info
log are not printed.
It is due to the reason that the logging level is set at the warning
level. Any log below the warning
level i.e. info
and debug
logs will not be printed. To print these logs, we will have to set the logging level to info
. For this, we use the setLevel()
method defined in the logging
module.
The setLevel()
method is invoked on a logger
object. The logger
object is created using the getLogger()
method defined in the logging
module. The getLogger()
method takes a string as input. The string is assigned as the name of the logger. After setting the logging level to a definite level, all the logs having priority more than this level will be printed. The following example shows how it works.
import logging
logger = logging.getLogger("myLogger")
logger.setLevel(logging.CRITICAL)
logger.debug("This is a sample debug message")
logger.info("This is a sample info message")
logger.warning("This is a sample warning message")
logger.error("This is a sample error message")
logger.critical("This is a sample critical message")
Output:
This is a sample critical message
Here, only the critical
log is printed as we have defined the logging level at critical
. Also, you can see that only the message is printed and not the log type and the root
keyword. It is because we have defined a custom logger named myLogger
using the getLogger()
function. To print the log type and the logger name, we need to use a python log formatter.
What Is a Python Log Handler?
Simply printing a message to the log file will yield us no information about the errors. So, we need to format the log messages to get the required information from the log files. For this, we use different log formatters and handlers.
You can think of the handler objects as channels for sending log messages to their specific destination. There are different types of handler objects, such as FileHandler
and StreamHandler
objects. The FileHandler
object is created using the FileHandler()
method. As shown below, it takes a file name as input and returns a FileHandler
object.
fileHandler = logging.FileHandler("test_file.log")
logger.addHandler(fileHandler)
Similarly, a StreamHandler
object is created using the StreamHandler()
method. While the FileHandler
objects direct the logs to a specific file, the StreamHandler
object directs the logs to a specific stream. When we don’t pass any input argument to the StreamHandler()
method, it directs the logs to the standard output stream. You can create a StreamHandler
, as shown below.
streamHandler = logging.StreamHandler()
logger.addHandler(streamHandler)
After creating a handler object, we add the handler to the logger using the addHandler()
method. The addHandler()
method is invoked on a logger
object, and it takes a handler object as an input argument. After execution of the addHandler()
method, the handler is added to the logger
.
What Is Python Log Formatter?
A log formatter in Python is used to configure the final structure and content of the logs. Using a python log formatter, we can include log name
, time
, date
, severity
, and other information along with the log message using the % operator.
To define the format of a log, we use the Formatter()
method. The Formatter()
method takes a string containing different attributes such as asctime
, name
, levelname
, etc as an input argument. After execution, the Formatter()
method returns a Formatter
object.
formatter = logging.Formatter("%(asctime)s %(name)s %(levelname)s: %(message)s")
Here,
- The
asctime
attribute denotes the time when the log record is created. - The
name
attribute denotes the name of the logger used for logging the call. - The
levelname
attribute denotes the logging level of the message such as debug, info, warning, error, or critical. You can read more about other log attributes here.
After creating a Formatter
object, we set the format of the log using the setFormatter()
method. The setFormatter()
method is invoked on a handler object. We have used a StreamHandler
to print the logs to the standard output in our program. When invoked on a handler object, the setFormatter()
function takes a Formatter
object as an input argument and sets the log format in the handler.
streamHandler.setFormatter(formatter)
After setting the format of the log messages, you can log the messages as you do normally, and they will be sent to the output stream in the defined format.
import logging
logger = logging.getLogger("myLogger")
streamHandler = logging.StreamHandler()
logger.addHandler(streamHandler)
formatter = logging.Formatter("%(asctime)s %(name)s %(levelname)s: %(message)s")
streamHandler.setFormatter(formatter)
logger.debug("This is a sample debug message")
logger.info("This is a sample info message")
logger.warning("This is a sample warning message")
logger.error("This is a sample error message")
logger.critical("This is a sample critical message")
Output:
2021-12-28 02:33:42,933 myLogger WARNING: This is a sample warning message
2021-12-28 02:33:42,933 myLogger ERROR: This is a sample error message
2021-12-28 02:33:42,933 myLogger CRITICAL: This is a sample critical message
Here, you can see that we have only logged the message using the warning()
method. The log has been printed in the format we have defined using the Formatter()
method. It contains all the details about the log, like the date and time of log creation, the name of the logger, and the log type. Now that we have learned the entire process in chunks, I have mentioned the step-by-step process to create a python log formatter to format log outputs below.
Steps to Create a Python Log Formatter
-
Create a logger object using the
getLogger()
method. -
Create a
FileHandler
orStreamHandler
object using theFileHandler()
method or theStreamHandler()
method. -
Add the
FileHandler
or theStreamHandler
object to the logger using theaddHandler()
method. -
Create a python Log Formatter using the
Formatter()
method. -
Apply the formatter using the
setFormatter()
method on theFileHandler
or theStreamHandler
object.
Conclusion
In this article, we have discussed how logging works in Python. We also discussed handlers and log formatters in Python. In this article, we used the StreamHandler
object and the log formatter to demonstrate how the formatting works. However, you should always use the FileHandler
object to create logs because we should always store logs in files to be examined for errors if anything unexpected happens. Also, you can use many other log attributes given in this article to format the log messages for better understanding.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub