How to Propagate Logging in Python
- Understanding Python Logging
- Setting Up Basic Logging
- Using LevelFilter for Propagation
- Advanced Logging Configuration
- Conclusion
- FAQ
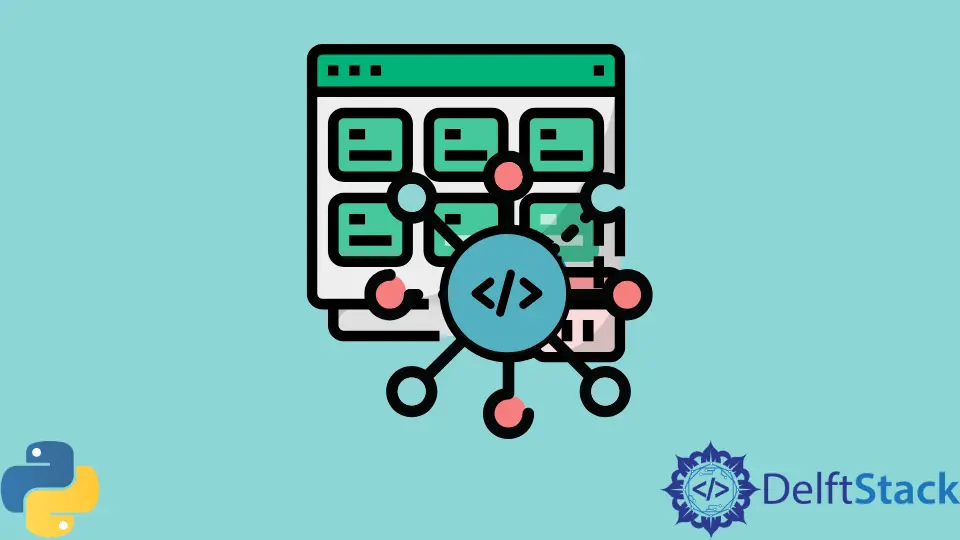
Logging is an essential part of any software development process, providing insights into application behavior and helping to diagnose issues. In Python, the logging module offers a powerful framework for tracking events that happen during runtime. One interesting aspect of this module is the ability to propagate log messages up the hierarchy of loggers, which can be controlled using LevelFilter.
In this tutorial, we’ll delve into how to effectively use LevelFilter for logging propagation in Python, ensuring that your logs are not only informative but also structured in a way that enhances their utility. Whether you are a beginner or an experienced developer, understanding logging propagation can significantly improve your debugging and monitoring capabilities.
Understanding Python Logging
Before we dive into propagation, it’s crucial to grasp what Python logging is. The logging module provides a flexible framework for emitting log messages from Python programs. It allows developers to track events, record errors, and understand the flow of applications. The logging system is hierarchical, meaning loggers can inherit properties from parent loggers. This is where propagation comes into play.
When a logger handles a log message, it can choose to pass that message up to its parent logger. This behavior is controlled by the propagate
attribute of the logger. By default, this attribute is set to True
, meaning messages are propagated. However, you can adjust this behavior using LevelFilter to filter messages based on their severity before they reach the parent logger.
Setting Up Basic Logging
To get started with logging in Python, you first need to set up a basic logging configuration. Here’s how you can do it:
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger('example_logger')
logger.debug('This is a debug message.')
logger.info('This is an info message.')
logger.warning('This is a warning message.')
logger.error('This is an error message.')
logger.critical('This is a critical message.')
Output:
DEBUG:example_logger:This is a debug message.
INFO:example_logger:This is an info message.
WARNING:example_logger:This is a warning message.
ERROR:example_logger:This is an error message.
CRITICAL:example_logger:This is a critical message.
In this code, we first import the logging module and set up a basic configuration that logs messages at the DEBUG level and above. We create a logger named example_logger
and emit various log messages. Each message is tagged with its severity level, which helps in filtering and understanding the log output.
Using LevelFilter for Propagation
Now, let’s explore how to use LevelFilter to control the propagation of log messages. LevelFilter allows you to specify the minimum logging level for messages that should be passed to the parent logger. Here’s an example:
class LevelFilter(logging.Filter):
def __init__(self, level):
super().__init__()
self.level = level
def filter(self, record):
return record.levelno >= self.level
parent_logger = logging.getLogger('parent_logger')
parent_logger.setLevel(logging.DEBUG)
child_logger = logging.getLogger('parent_logger.child')
child_logger.setLevel(logging.INFO)
child_logger.addFilter(LevelFilter(logging.WARNING))
child_logger.debug('This debug message will not be propagated.')
child_logger.info('This info message will not be propagated.')
child_logger.warning('This warning message will be propagated.')
Output:
WARNING:parent_logger.child:This warning message will be propagated.
In this example, we define a custom filter class, LevelFilter
, that checks if the log message level is greater than or equal to a specified threshold. We set up a parent logger and a child logger. The child logger has a filter that allows only messages with a level of WARNING or higher to propagate to the parent logger. As a result, only the warning message is logged.
Advanced Logging Configuration
For more complex applications, you may want to configure your logging in a more advanced way. This includes setting different handlers, formatting, and levels for different loggers. Here’s an example that demonstrates this:
import logging
logger = logging.getLogger('my_app')
logger.setLevel(logging.DEBUG)
handler = logging.FileHandler('app.log')
handler.setLevel(logging.WARNING)
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
handler.setFormatter(formatter)
logger.addHandler(handler)
logger.debug('This debug message will not be logged to file.')
logger.warning('This warning message will be logged to file.')
logger.error('This error message will be logged to file.')
Output:
This warning message will be logged to file.
This error message will be logged to file.
In this setup, we create a file handler that writes log messages to app.log
and is configured to log only WARNING level messages and above. The formatter specifies the output format, including the timestamp, logger name, log level, and message. Debug messages are ignored by the handler, while warning and error messages are recorded in the log file.
Conclusion
In conclusion, understanding how to propagate logging in Python using LevelFilter can greatly enhance your logging strategy. By controlling which log messages are passed to parent loggers, you can create a more organized and useful logging system. This not only aids in debugging but also improves the maintainability of your code. As you continue to develop applications, remember that effective logging is an invaluable tool in your programming toolkit. Take the time to implement and refine your logging strategy to reap the benefits in the long run.
FAQ
-
What is logging in Python?
Logging in Python is a built-in module that provides a way to track events that happen during program execution, allowing developers to debug and monitor applications effectively. -
How does logging propagation work?
Logging propagation in Python allows log messages to be passed from child loggers to parent loggers. This is controlled by thepropagate
attribute of the logger.
-
What is LevelFilter in Python logging?
LevelFilter is a custom logging filter that allows you to specify a minimum log level for messages that should be passed to a parent logger, helping to control the flow of log messages. -
Can I log messages to a file in Python?
Yes, you can log messages to a file using theFileHandler
class in the logging module, allowing you to store log messages for later analysis. -
How can I customize the format of log messages?
You can customize the format of log messages by using theFormatter
class in the logging module, specifying the desired output format for your logs.