How to Log Message to File and Console in Python
-
Use the
logging
Module to Print the Log Message to File and Console in Python -
Use the
logging
Module to Print the Log Message to Console in Python -
Use
logging
Module to Print Log Message to a File in Python
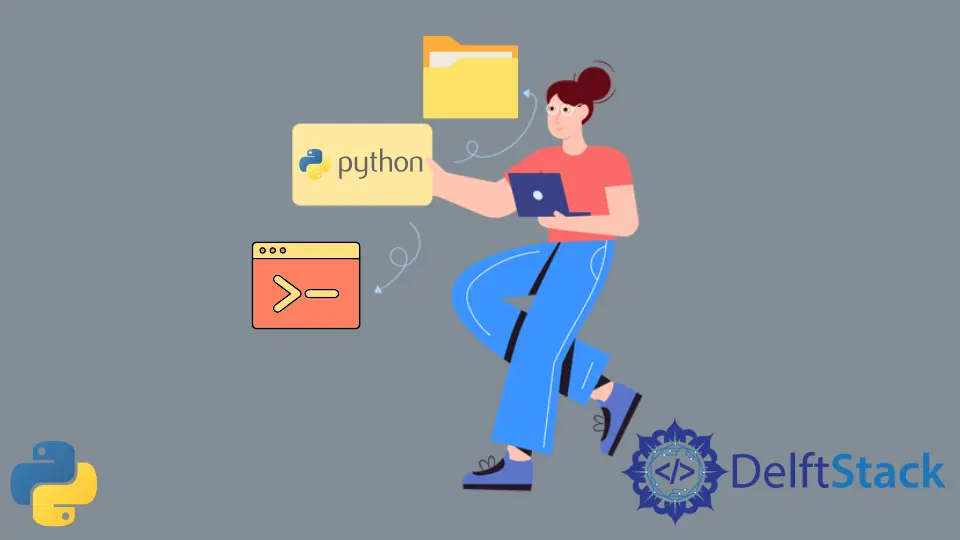
This tutorial will introduce some methods to log debug strings into a file and console in Python.
Use the logging
Module to Print the Log Message to File and Console in Python
Logging is the process of keeping records of various events happening in a system. Those events could be input data, processes, threads, output data, processed information, errors, warnings, notices. The various events are tracked and stored in a log file. The logging
module is provided by Python that facilitates the programmers to log the various events occurred. The module has specific classes and methods. Loggers
, Handlers
, Filters
and Formatters
are the basic classes that the logging
module defines.
The basicConfig()
method performs the basic configuration. It creates a StreamHandler
using a default Formatter
. Then, it adds to the root logger. The logging levels are represented in numeric values. There are six logging levels: CRITICAL
, ERROR
, WARNING
, INFO
, DEBUG
, and NOTSET
. We have set the logging level to INFO
down below. Hence, INFO
, WARNING
, ERROR
, and CRITICAL
are displayed or stored.
To write to console and file, we will use logging.basicConfig()
, which can take argument handlers and simplify logging setup a lot, especially when setting up multiple handlers with the same formatter. We use the FileHandler()
method to write to a file and use debug.log
to log all the information. Similarly, to write to a console, we use StreamHandler()
. By default StreamHandler()
writes to stderr. To write to stdout, we import the sys
module and pass sys.stdout
explicitly to StreamHandler()
.
In the example below, the logs are printed in the console and the file debug.log
.
# python 3.x
import logging
import sys
logging.basicConfig(
level=logging.INFO,
format="%(asctime)s [%(levelname)s] %(message)s",
handlers=[logging.FileHandler("debug.log"), logging.StreamHandler(sys.stdout)],
)
logging.debug("This message is skipped as a level is set as INFO")
logging.info("So should this")
logging.warning("And this, too")
logging.error("Testing non-ASCII character, Ø and ö")
Output:
2021-07-28 14:50:01,348 [INFO] So should this
2021-07-28 14:50:01,349 [WARNING] And this, too
2021-07-28 14:50:01,349 [ERROR] Testing non-ASCII character, Ø and ö
Use the logging
Module to Print the Log Message to Console in Python
To use logging and set up the basic configuration, we use logging.basicConfig()
. Then instead of print()
, we call logging.{level}(message)
to show the message in the console. Since we configured level as INFO in the basicConfig()
setting, we called logging.info()
later in the program. And the whole message in the string is passed to logging.info()
, which is then displayed to the console.
Example Code:
# python 3.x
import logging
logging.basicConfig(level=logging.INFO)
def area(l, b):
"""Compute the area of a rectangle"""
return l * b
logging.info(
"Area of length: {l} and breadth: {b} is {c}".format(l=3, b=4, c=area(l=3, b=4))
)
Output:
INFO:root:Area of length: 3 and breadth: 4 is 12
Use logging
Module to Print Log Message to a File in Python
If we are using multiple modules in a program, then using the root module has severe limitations. That’s why we need to create a new logger using the logger.getLogger(name)
method.
There is a convention to use __name__
variable as the name of the logger. Once we have created a new logger, we should remember to log all our messages using the new logger.info()
instead of the root’s logging.info()
method. A FileHandler()
is used to make our custom logger log in to a different file. Here we logged our information to logfile.log
. Likewise, a Formatter()
is used to change the format of our logged messages. And the Formatter
is set on the FileHandler
object and not directly on the logger.
For example, use getLogger()
to create or get a logger. Then, use the setLogger()
method to set the log level. Set the level to logging.WARNING
. Next, use the FileHandler()
method to define the handler and the Formatter()
method to set the formatter. Then, use the addHandler()
method to add the handler to the logger. Finally, write some messages to test. We can see the logs in the file log_file.log
.
Example Code:
# python 3.x
import logging
logger = logging.getLogger(__name__)
logger.setLevel(logging.WARNING)
handler = logging.FileHandler("log_file.log")
formatter = logging.Formatter(
"%(asctime)s : %(name)s : %(funcName)s : %(levelname)s : %(message)s"
)
handler.setFormatter(formatter)
logger.addHandler(handler)
logger.debug("A debug message")
logger.info("An info message")
logger.warning("There is something wrong")
logger.error("An error has happened.")
logger.critical("Fatal error occured. Cannot continue")
Output:
2021-07-30 11:55:31,047 : __main__ : <module> : WARNING : There is something wrong
2021-07-30 11:55:31,047 : __main__ : <module> : ERROR : An error has happened.
2021-07-30 11:55:31,047 : __main__ : <module> : CRITICAL : Fatal error occured. Cannot continue