How to Write Logs to a File in Python
-
Write Logs to a File With the
logging
Module in Python -
Write Logs to a File With the
logging.FileHandler()
Function in Python
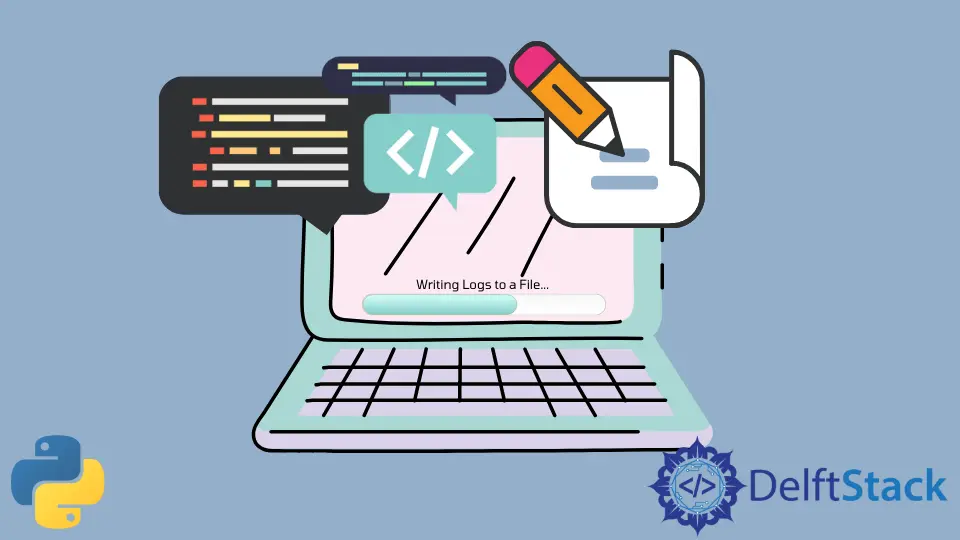
This tutorial will introduce the methods to write logs to a file in Python.
Write Logs to a File With the logging
Module in Python
The logs are used for debugging a program and finding out what went wrong. The logging
module is used for logging data to a file in Python. We can use the logging.basicConfig()
function to configure the logs to be written to a specific file. By default, we can log 5 types of lines with the logging
module. These message types include debug, info, warning, error, and critical. Still, we can increase that number to whatever we need through coding. The following code example shows us how to write logs to a file with the logging.basicConfig()
function.
import logging
# Creating and Configuring Logger
Log_Format = "%(levelname)s %(asctime)s - %(message)s"
logging.basicConfig(
filename="logfile.log", filemode="w", format=Log_Format, level=logging.ERROR
)
logger = logging.getLogger()
# Testing our Logger
logger.error("Our First Log Message")
The file content of logfile.log
:
ERROR 2021-06-13 04:14:29,604 - Our First Log Message
We wrote the log message Our First Log Message
of level error
with the proper date and time of logging by using the logging.basicConfig()
function in the code above. The most important thing while writing the logs is the date and time of their occurrence. So, we first created a format for our logs inside the Log_Format
string. This format includes the level of the log, the date and time of occurrence, and the message to be written.
Then, we use the filename
parameter of the logging.basicConfig()
function to specify the log file. We assigned the value a
to the filemode
parameter of the logging.basicConfig()
function to open the file in append
mode so that the previous logs don’t get deleted every time there’s a new log. We then set the format
parameter to Log_Format
. After that step, we assigned the logging.ERROR
to the level
parameter to specify the minimum level of the logs to error. We created a logger object with logger = logging.getLogger()
to write log messages. In the end, we wrote the error message with logger.error("Our First Log Message")
.
Write Logs to a File With the logging.FileHandler()
Function in Python
We can also use the logging.FileHandler()
function to write logs to a file in Python. This function takes the file path where we want to write our logs. We can then use the addHandler()
function to add this handler to our logger object. The code below demonstrates how to write logs to a file with the logging.FileHandler()
function.
import logging
logger = logging.getLogger()
handler = logging.FileHandler("logfile.log")
logger.addHandler(handler)
logger.error("Our First Log Message")
logfile.log contents:
Our First Log Message
We wrote the log message, Our First Log Message
, to the file logfile.log
with the logging.FileHandler()
function in the code above. We first created a logger object that we will use to write logs with the logging.getLogger()
function. Then, we create a file handler, handler
, and assigned logging.FileHandler('logfile.log')
to it. After that, we added this new handler
to our logger object with logger.addHandler(handler)
. In the end, we wrote the error message to our file with logger.error('Our First Log Message')
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn