How to List Subdirectories in Python
-
List Subdirectories With the
glob
Module in Python -
List Subdirectories With the
os.scandir()
Function in Python -
List Subdirectories With the
os.walk()
Function in Python
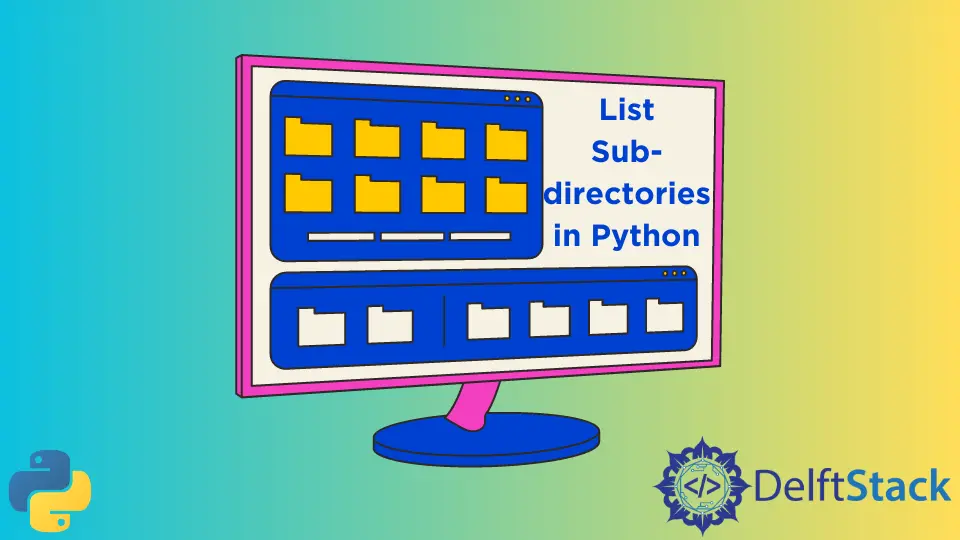
There are three effective methods that you can use to list all the subdirectories inside a specified directory in Python: the glob.glob()
function, the os.scandir() function, and the os.walk()
function.
This tutorial will demonstrate how you can work with these functions to list subdirectories in Python.
List Subdirectories With the glob
Module in Python
The glob
module is used to get path names that match a specific pattern. We can use the glob()
function inside the glob
module of Python to list all the subdirectories of a specified main directory by specifying /*/
at the end of the directory path. The following program snippet shows us how to list all the subdirectories inside the main directory using the glob
module:
import os
from glob import glob
glob(os.getcwd() + "/*/", recursive=True)
Output:
['/content/sample_data/', '/content/subdirectory/']
We listed all the subdirectories of our current working directory with the glob
module in the code above. This method’s disadvantage is that it doesn’t display any hidden directory or directory where the directory name starts with a period .
; it only shows the immediate subdirectories.
List Subdirectories With the os.scandir()
Function in Python
os
is a built-in module in Python that can be used for many Operating System-related functions like file storage. The os.scandir()
function can be used to list all the subdirectories of a specified main directory. This function takes the directory path as an input parameter and returns an iterator that can be used to iterate through files and directories inside the path.
The following code snippet shows us how to list all the subdirectories inside the main directory with the os.scandir()
function:
import os
[f.path for f in os.scandir(os.getcwd()) if f.is_dir()]
Output:
['/content/.config',
'/content/.ipynb_checkpoints',
'/content/sample_data',
'/content/subdirectory']
We listed all the subdirectories of our current working directory with the os.scandir()
function in the code above. This process is an improvement over the previous approach because it also lists the hidden directories or the directories where the directory name starts with a period .
. This method’s only disadvantage is it only shows the immediate child directories of the main parent directory. It does not get the subsubdirectories inside the main directory.
List Subdirectories With the os.walk()
Function in Python
The os.walk()
function is another method that can be used to list all the subdirectories inside a single main directory in Python. The os.walk()
function takes the path of the main directory as an input parameter and returns a set of tuples, where the first element of each tuple is the path of a subdirectory inside the directory tree. This includes all the subdirectories and their subsubdirectories until the directory tree ends.
The os.walk()
function also gives us the hidden directories or the directories where the directory name starts with a period .
. The following code snippet shows us how to list all the subdirectories inside a main directory with the os.walk()
function:
import os
[x[0] for x in os.walk(os.getcwd())]
Output:
['/content',
'/content/.config',
'/content/.config/logs',
'/content/.config/logs/2021.05.06',
'/content/.config/configurations',
'/content/.ipynb_checkpoints',
'/content/sample_data',
'/content/sample_data/.ipynb_checkpoints',
'/content/sample_data/subsubdirectory',
'/content/subdirectory']
We listed all the subdirectories of our current working directory with the os.scandir()
function in the program above. As we can see, the output shows all the directories inside the directory tree. The result also includes all the hidden directories.
Even though the os.walk()
approach shows us all the subdirectories and the subsubdirectories with all the hidden directories, all the methods discussed above have their own unique use cases and are helpful in different scenarios. The best approach among the ones we discussed depends on the specific problem you’re trying to solve.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python