How to Overload Function in Python
- Overloading Definition
- Function Overloading in Python
- Use Multiple Dispatch Decorator to Perform Function Overloading in Python
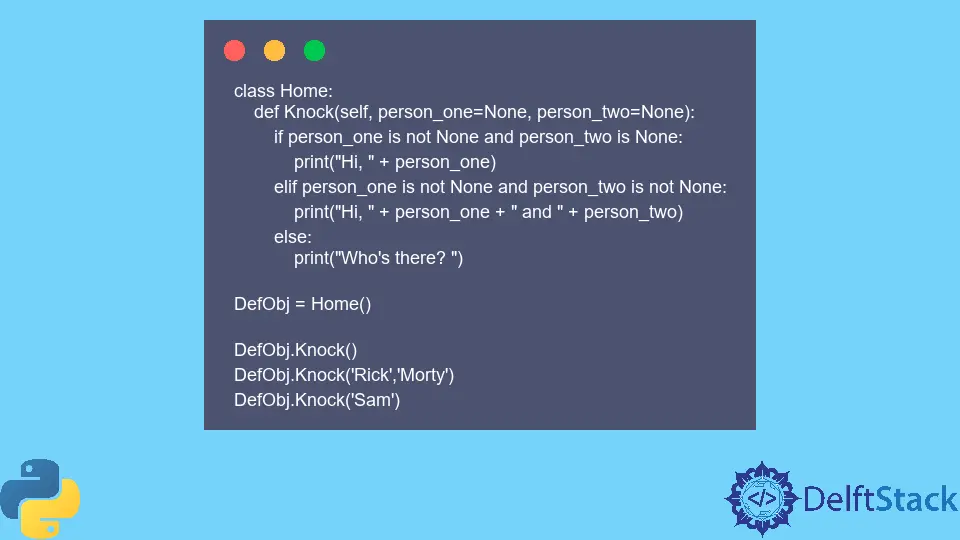
This article talks about Python function overloading and how you can perform it. We’ve included methods and sample programs for you to follow below.
Overloading Definition
In programming, the term overloading helps a function act in various ways based on the arguments and parameters stored in it. Overloading allows the reuse of a program repeatedly; for example, instead of passing multiple methods that are not too different from each other, only one method can be passed and can be overloaded. Overloading a function also makes a program clearer and less complex.
You should not use this process excessively because it creates confusion while managing several overloaded functions.
Function Overloading in Python
In Python, a function can be created and called several times by passing many arguments or parameters in it. This process of calling the same function, again and again, using different arguments or parameters is called Function Overloading.
class Home:
def Knock(self, person_one=None, person_two=None):
if person_one is not None and person_two is None:
print("Hi, " + person_one)
elif person_one is not None and person_two is not None:
print("Hi, " + person_one + " and " + person_two)
else:
print("Who's there? ")
DefObj = Home()
DefObj.Knock()
DefObj.Knock("Rick", "Morty")
DefObj.Knock("Sam")
In this program, we initially define a class called Home
, in which we define a function Knock
. After that, the if-else
conditional statement gives different conditions based on different inputs. Then we create an object called DefObj
that the main class Home
uses to call the function Knock
. Finally, we have called the function Knock
more than once using different arguments. Therefore, we have used function overloading in this program.
Use Multiple Dispatch Decorator to Perform Function Overloading in Python
Decorators in Python are the tools that help in modifying the behavior of a particular class or function in the program. They are used to add other functions to modify the existing function without actually changing it.
A dispatch decorator helps you select implementations from a number of executions from the same abstract function based on the given list of types.
Take a look at the example program below:
from multipledispatch import dispatch
@dispatch(float, float, float)
def summation(float_1, float_2, float_3):
total = float_1 + float_2 + float_3
print(total)
@dispatch(int, int, int)
def difference(int_1, int_2, int_3):
diff = int_1 - int_2 - int_3
print(diff)
@dispatch(int, int, int)
def multiply(int_1, int_2, int_3):
product = int_1 * int_2 * int_3
print(product)
summation(6.9, 3.14, 7.12)
difference(9, 6, 3)
product(3, 3, 3)
Output:
17.16
0
27
Here, you can pass any data type to the dispatcher. The dispatcher is used to create an object with different implementations. During execution, the appropriate function is selected by just using the function name, the number of arguments, and the data type of the arguments.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn