Python 中的函式過載
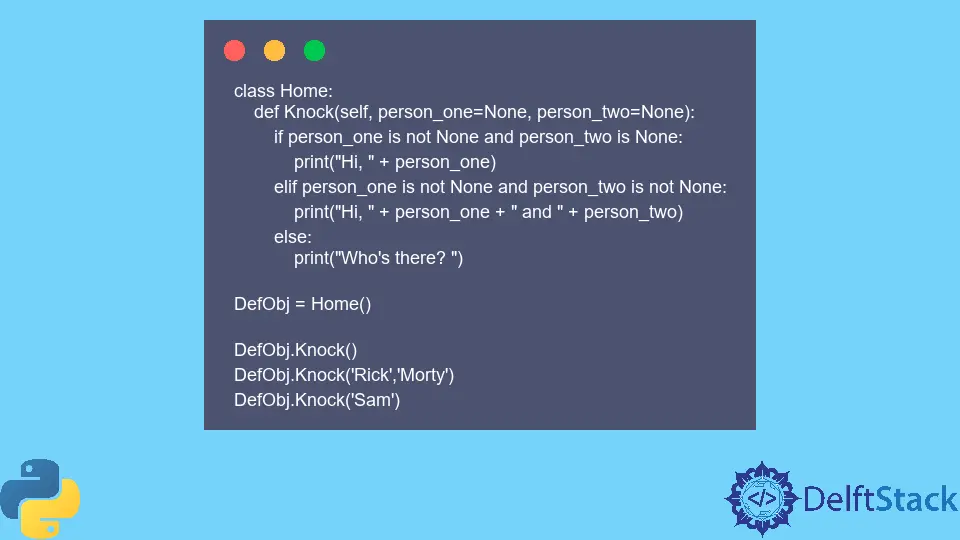
本文討論 Python 函式過載以及如何執行它。我們在下面提供了方法和示例程式供你遵循。
過載定義
在程式設計中,術語過載幫助函式根據儲存在其中的引數和引數以各種方式執行。過載允許重複重用程式;例如,不是傳遞彼此相差不大的多個方法,而是隻能傳遞一個方法並且可以過載。過載函式還可以使程式更清晰、更簡單。
你不應過度使用此過程,因為它在管理多個過載函式時會造成混亂。
Python 中的函式過載
在 Python 中,可以通過在其中傳遞許多引數或引數來多次建立和呼叫函式。使用不同的引數或引數一次又一次地呼叫同一個函式的過程稱為函式過載。
class Home:
def Knock(self, person_one=None, person_two=None):
if person_one is not None and person_two is None:
print("Hi, " + person_one)
elif person_one is not None and person_two is not None:
print("Hi, " + person_one + " and " + person_two)
else:
print("Who's there? ")
DefObj = Home()
DefObj.Knock()
DefObj.Knock("Rick", "Morty")
DefObj.Knock("Sam")
在這個程式中,我們最初定義了一個名為 Home
的類,我們在其中定義了一個函式 Knock
。之後,if-else
條件語句根據不同的輸入給出不同的條件。然後我們建立一個名為 DefObj
的物件,主類 Home
使用它來呼叫函式 Knock
。最後,我們使用不同的引數不止一次呼叫了函式 Knock
。因此,我們在這個程式中使用了函式過載。
在 Python 中使用 Multiple Dispatch Decorator
執行函式過載
Python 中的裝飾器是幫助修改程式中特定類或函式行為的工具。它們用於新增其他功能以修改現有功能,而無需實際更改它。
Dispatch Decorator
可幫助你根據給定的型別列表從同一抽象函式的多個執行中選擇實現。
看看下面的示例程式:
from multipledispatch import dispatch
@dispatch(float, float, float)
def summation(float_1, float_2, float_3):
total = float_1 + float_2 + float_3
print(total)
@dispatch(int, int, int)
def difference(int_1, int_2, int_3):
diff = int_1 - int_2 - int_3
print(diff)
@dispatch(int, int, int)
def multiply(int_1, int_2, int_3):
product = int_1 * int_2 * int_3
print(product)
summation(6.9, 3.14, 7.12)
difference(9, 6, 3)
product(3, 3, 3)
輸出:
17.16
0
27
在這裡,你可以將任何資料型別傳遞給排程程式。排程器用於建立具有不同實現的物件。在執行過程中,僅通過使用函式名稱、引數數量和引數的資料型別來選擇合適的函式。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn