Python 中的函数重载
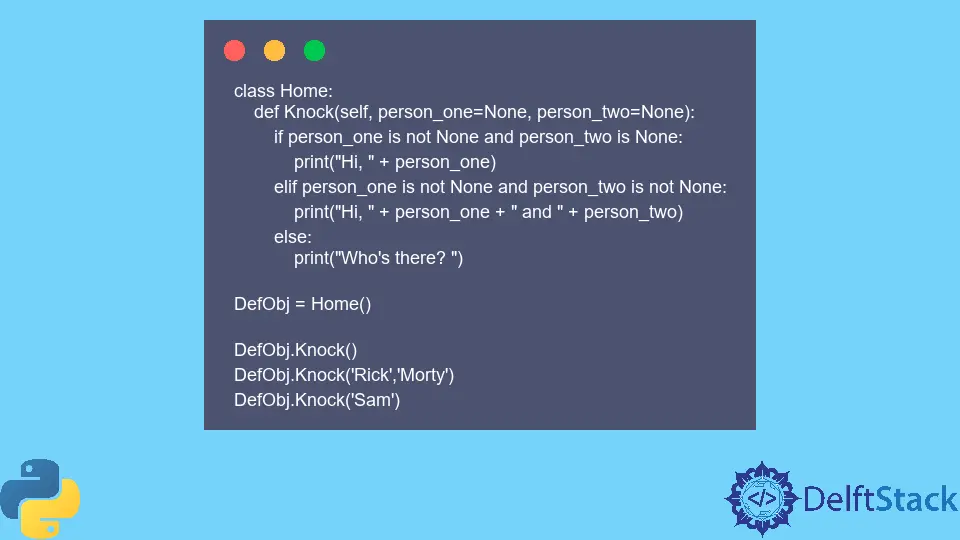
本文讨论 Python 函数重载以及如何执行它。我们在下面提供了方法和示例程序供你遵循。
重载定义
在编程中,术语重载帮助函数根据存储在其中的参数和参数以各种方式运行。重载允许重复重用程序;例如,不是传递彼此相差不大的多个方法,而是只能传递一个方法并且可以重载。重载函数还可以使程序更清晰、更简单。
你不应过度使用此过程,因为它在管理多个重载函数时会造成混乱。
Python 中的函数重载
在 Python 中,可以通过在其中传递许多参数或参数来多次创建和调用函数。使用不同的参数或参数一次又一次地调用同一个函数的过程称为函数重载。
class Home:
def Knock(self, person_one=None, person_two=None):
if person_one is not None and person_two is None:
print("Hi, " + person_one)
elif person_one is not None and person_two is not None:
print("Hi, " + person_one + " and " + person_two)
else:
print("Who's there? ")
DefObj = Home()
DefObj.Knock()
DefObj.Knock("Rick", "Morty")
DefObj.Knock("Sam")
在这个程序中,我们最初定义了一个名为 Home
的类,我们在其中定义了一个函数 Knock
。之后,if-else
条件语句根据不同的输入给出不同的条件。然后我们创建一个名为 DefObj
的对象,主类 Home
使用它来调用函数 Knock
。最后,我们使用不同的参数不止一次调用了函数 Knock
。因此,我们在这个程序中使用了函数重载。
在 Python 中使用 Multiple Dispatch Decorator
执行函数重载
Python 中的装饰器是帮助修改程序中特定类或函数行为的工具。它们用于添加其他功能以修改现有功能,而无需实际更改它。
Dispatch Decorator
可帮助你根据给定的类型列表从同一抽象函数的多个执行中选择实现。
看看下面的示例程序:
from multipledispatch import dispatch
@dispatch(float, float, float)
def summation(float_1, float_2, float_3):
total = float_1 + float_2 + float_3
print(total)
@dispatch(int, int, int)
def difference(int_1, int_2, int_3):
diff = int_1 - int_2 - int_3
print(diff)
@dispatch(int, int, int)
def multiply(int_1, int_2, int_3):
product = int_1 * int_2 * int_3
print(product)
summation(6.9, 3.14, 7.12)
difference(9, 6, 3)
product(3, 3, 3)
输出:
17.16
0
27
在这里,你可以将任何数据类型传递给调度程序。调度器用于创建具有不同实现的对象。在执行过程中,仅通过使用函数名称、参数数量和参数的数据类型来选择合适的函数。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn