How to Read User Input as Integers in Python
- Reading Input in Python 3
- Handling Errors in Python 3
- Reading Input in Python 2
- Handling Errors in Python 2
- Conclusion
- FAQ
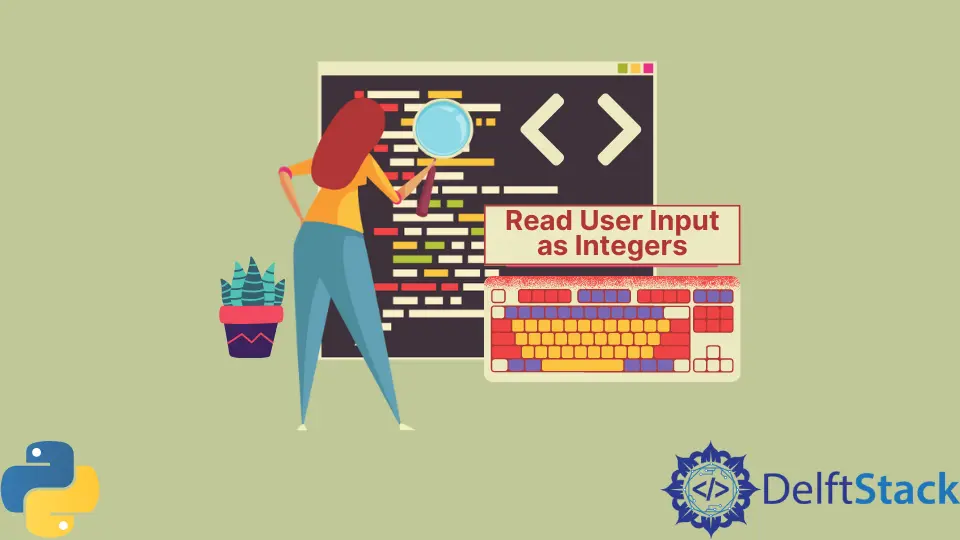
Reading user input is a fundamental aspect of programming in Python. Whether you’re developing a simple script or a complex application, understanding how to handle user input effectively is crucial.
In this article, we will explore how to read user input as integers in both Python 2 and Python 3. While the syntax may differ slightly between the two versions, the core concept remains the same. This guide will provide you with clear examples and explanations to help you grasp this essential skill. So, let’s dive in and learn how to read user input as integers in Python!
Reading Input in Python 3
In Python 3, the input()
function is used to read user input. By default, this function reads input as a string, so you need to convert it to an integer using the int()
function. Here’s how you can do it:
user_input = input("Please enter an integer: ")
integer_value = int(user_input)
print("You entered:", integer_value)
When you run this code, the program prompts the user to enter an integer. After the user inputs a value, the int()
function converts that string into an integer. Finally, the program prints the integer value.
Output:
Please enter an integer: 42
You entered: 42
This method is straightforward and effective. However, keep in mind that if the user inputs a non-integer value, it will raise a ValueError
. To handle such cases gracefully, you can use a try-except block to catch the error and prompt the user again.
Handling Errors in Python 3
To ensure a smooth user experience, it’s essential to handle potential errors when converting input to integers. Here’s an example of how to implement error handling in Python 3:
while True:
user_input = input("Please enter an integer: ")
try:
integer_value = int(user_input)
print("You entered:", integer_value)
break
except ValueError:
print("That's not a valid integer. Please try again.")
In this code, we use a while
loop to repeatedly prompt the user for input until they provide a valid integer. The try
block attempts to convert the input to an integer, while the except
block catches any ValueError
and informs the user of the mistake.
Output:
Please enter an integer: hello
That's not a valid integer. Please try again.
Please enter an integer: 10
You entered: 10
This method enhances user interaction by allowing them to correct their mistakes without crashing the program. It’s a best practice to validate user input, making your application more robust and user-friendly.
Reading Input in Python 2
In Python 2, the approach to reading user input differs slightly. Instead of input()
, you use the raw_input()
function, which reads input as a string. To convert it to an integer, you still use the int()
function. Here’s how it looks:
user_input = raw_input("Please enter an integer: ")
integer_value = int(user_input)
print "You entered:", integer_value
In this example, raw_input()
captures the user input as a string, which is then converted into an integer using int()
. Finally, the program prints out the integer value.
Output:
Please enter an integer: 25
You entered: 25
Just like in Python 3, you need to be cautious about non-integer inputs. If a user enters something that cannot be converted into an integer, it will raise a ValueError
.
Handling Errors in Python 2
To manage errors in Python 2, you can also use a try-except block. Here’s an example that illustrates this:
while True:
user_input = raw_input("Please enter an integer: ")
try:
integer_value = int(user_input)
print "You entered:", integer_value
break
except ValueError:
print "That's not a valid integer. Please try again."
In this code snippet, the loop continues to prompt the user until they enter a valid integer. The try
block attempts to convert the input to an integer, while the except
block catches any ValueError
and asks the user to try again.
Output:
Please enter an integer: abc
That's not a valid integer. Please try again.
Please enter an integer: 7
You entered: 7
This error handling mechanism in Python 2 functions similarly to that in Python 3, allowing for a better user experience by providing feedback on invalid input.
Conclusion
Understanding how to read user input as integers in Python is a vital skill for any programmer. Whether you are using Python 2 or Python 3, the core concepts remain the same, though the syntax varies slightly. By employing error handling techniques, you can create more robust and user-friendly applications. Remember that validating user input is not just a good practice; it’s essential for ensuring that your programs run smoothly. So, go ahead and implement these techniques in your projects!
FAQ
-
How do I read user input as an integer in Python 3?
You can use theinput()
function to read input as a string and then convert it to an integer usingint()
. -
What happens if the user inputs a non-integer value?
If a non-integer value is entered, it raises aValueError
. You can handle this using a try-except block. -
Is there a difference between Python 2 and Python 3 for reading input?
Yes, in Python 2, you useraw_input()
to read input as a string, while in Python 3, you useinput()
.
-
Can I validate multiple types of input?
Yes, you can implement additional checks to validate various types of input, depending on your application’s needs. -
What is the best practice for handling user input?
Always validate user input and provide feedback to the user to enhance their experience and prevent crashes.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook