How to Create a Dice Roll Simulator in Python
- Understanding the Basics of Random Number Generation
- Enhancing the Simulator with Multiple Rolls
- Adding User Interaction and Customization
- Conclusion
- FAQ
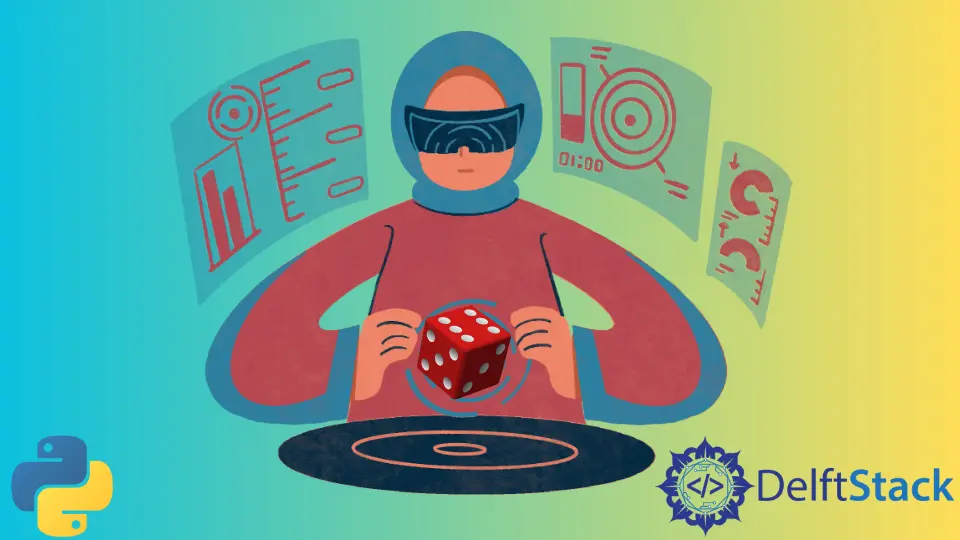
Creating a Dice Roll Simulator in Python can be an exciting project for both beginners and seasoned programmers. This simple yet engaging task allows you to explore Python’s random number generation capabilities while delivering a fun and interactive experience. Whether you’re developing a game, conducting simulations, or just having fun with coding, a dice roll simulator is a great way to practice your skills.
In this article, we’ll guide you through the process of building a Dice Roll Simulator step by step, showcasing the necessary code, explanations, and output. Let’s dive into the world of Python programming and roll some dice!
Understanding the Basics of Random Number Generation
Before we jump into coding, it’s essential to understand how random number generation works in Python. The random
module is a built-in library that provides various functions to generate random numbers. For our dice roll simulator, we will specifically use the randint()
function, which allows us to generate a random integer within a specified range. In the context of a dice roll, this means generating a number between 1 and 6, inclusive.
Here’s a simple code snippet to get started:
import random
def roll_dice():
return random.randint(1, 6)
result = roll_dice()
print("You rolled a:", result)
In this code, we first import the random
module. The roll_dice()
function uses random.randint(1, 6)
to simulate a dice roll. When we call this function, it returns a random integer between 1 and 6. Finally, we print the result to the console.
Output:
You rolled a: 4
This basic structure sets the foundation for our dice roll simulator. By understanding how to generate random numbers, we can build upon this code to add more features and functionality.
Enhancing the Simulator with Multiple Rolls
Now that we have a basic dice roll simulator, let’s enhance it by allowing users to roll the dice multiple times. This feature can be useful for games that require several rolls or for simulations that need to analyze multiple outcomes. We can achieve this by introducing a loop that asks the user how many times they want to roll the dice.
Here’s how we can modify our existing code:
import random
def roll_dice():
return random.randint(1, 6)
def roll_multiple_times(number_of_rolls):
results = []
for _ in range(number_of_rolls):
results.append(roll_dice())
return results
num_rolls = int(input("How many times do you want to roll the dice? "))
results = roll_multiple_times(num_rolls)
print("You rolled:", results)
In this code, we added a new function called roll_multiple_times()
, which takes the number of rolls as an argument. Inside this function, we use a loop to call roll_dice()
multiple times and store the results in a list. Finally, we print the list of results, giving the user a clear view of all the rolls.
Output:
How many times do you want to roll the dice? 3
You rolled: [2, 5, 1]
This enhancement not only makes the simulator more interactive but also allows users to gather more data about their rolls, which can be beneficial for analysis or gameplay.
Adding User Interaction and Customization
To make our Dice Roll Simulator even more engaging, we can add user interaction and customization options. For example, we can allow users to choose the number of sides on their dice. While traditional dice have six sides, we can easily extend our simulator to accommodate any number of sides.
Here’s how we can implement this feature:
import random
def roll_dice(sides):
return random.randint(1, sides)
def roll_multiple_times(sides, number_of_rolls):
results = []
for _ in range(number_of_rolls):
results.append(roll_dice(sides))
return results
sides = int(input("Enter the number of sides on the dice: "))
num_rolls = int(input("How many times do you want to roll the dice? "))
results = roll_multiple_times(sides, num_rolls)
print("You rolled:", results)
In this version, we modified the roll_dice()
function to accept the number of sides as an argument. The user is prompted to enter the number of sides they want on their dice before specifying how many times to roll. This flexibility allows users to simulate various types of dice, enhancing the overall experience.
Output:
Enter the number of sides on the dice: 10
How many times do you want to roll the dice? 5
You rolled: [3, 7, 1, 10, 4]
By adding customization options, we empower users to tailor their experience, making the simulator more versatile and fun.
Conclusion
Creating a Dice Roll Simulator in Python is a fantastic way to hone your programming skills while enjoying the process. We’ve covered the basics of random number generation, enhanced our simulator to allow multiple rolls, and added user interaction for customization. These steps not only make the simulator more engaging but also provide a solid foundation for further exploration in Python programming. Whether you’re building a game or simply learning the ropes, this project is an excellent addition to your coding repertoire. So grab your virtual dice and start rolling!
FAQ
- What is a Dice Roll Simulator?
A Dice Roll Simulator is a program that mimics the action of rolling dice, generating random numbers typically between 1 and 6.
-
Can I customize the number of sides on the dice?
Yes, our simulator allows you to specify the number of sides on the dice, so you can simulate various types of dice. -
Is Python the only language I can use to create a Dice Roll Simulator?
No, you can create a Dice Roll Simulator in many programming languages, but Python is a popular choice due to its simplicity and readability. -
How can I improve my Dice Roll Simulator?
You can add features like statistics tracking, animations, or even a graphical user interface (GUI) to enhance user experience. -
Where can I learn more about Python programming?
There are many online resources, including tutorials, courses, and documentation, that can help you learn Python programming effectively.