How to Shift Array in Python NumPy
-
NumPy Shift Array With the
np.roll()
Method - NumPy Shift Array With the Slicing Method in Python
-
NumPy Shift Array With
shift()
Function Inside thescipy.ndimage.interpolation
Library in Python
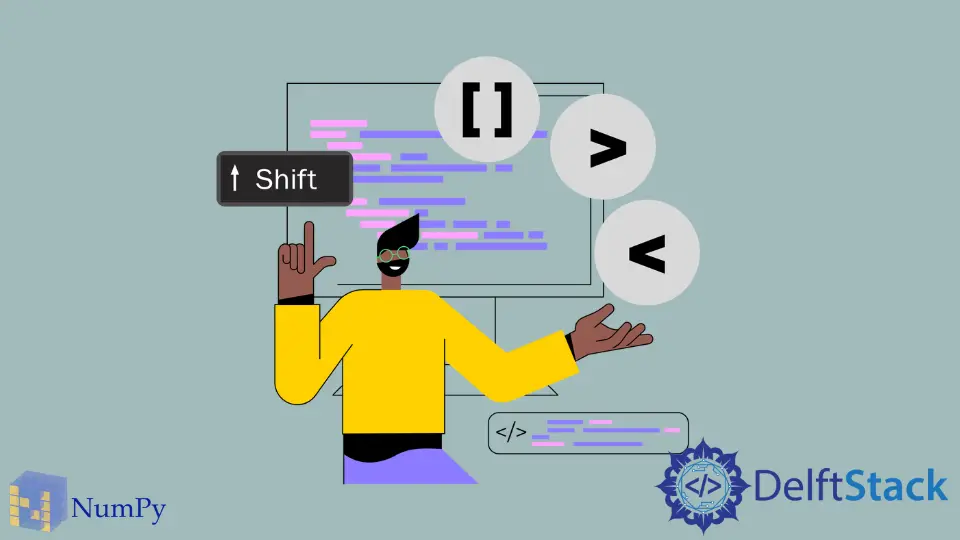
This tutorial will introduce methods to shift a NumPy array.
NumPy Shift Array With the np.roll()
Method
If we want to right-shift or left-shift the elements of a NumPy array, we can use the numpy.roll()
method in Python. The numpy.roll()
method is used to roll array elements along a specified axis. It takes the array and the number of places we want to shift the elements of the array and returns the shifted array. If we want to shift the elements towards the right, we have to use a positive integer as the shift value. If we want to shift the elements towards the left, we have to specify a negative shift value. The following code example shows how to shift elements of an array with the numpy.roll()
method.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
array_new = np.roll(array, 3)
print(array_new)
Output:
[3 4 5 1 2]
We first created the array with the np.array()
function. We then shifted the elements towards the right with the np.roll()
function and stored the resultant array inside array_new
.
NumPy Shift Array With the Slicing Method in Python
If we want to shift the elements toward right or left and replace the shifted indices with a constant value, we have to use the array slicing method in Python. We can create a new empty array just like our original array. If the shift value is positive, we fill the left side of the array with the constant value, and if the shift value is negative, we fill the right side of the array with the constant value. We can then fill the remaining indices of our new array with values from the original array. The following code example shows how to shift elements of an array with the array slicing method.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
num = -3
fill_value = 0
def shift(arr, num, fill_value):
result = np.empty_like(arr)
if num > 0:
result[:num] = fill_value
result[num:] = arr[:-num]
elif num < 0:
result[num:] = fill_value
result[:num] = arr[-num:]
else:
result[:] = arr
print(result)
shift(array, num, fill_value)
Output:
[4 5 0 0 0]
We defined the function shift()
that shifts the elements of our array three places towards the left using the array slicing method in the above code. We first created our original array with the np.array()
method. We then specified the number of indices num
we want to shift our array elements and the constant value fill_value
that we want to replace all the shifted indices with. In the end, we passed these values to the shift()
function that creates a new array result
like our original array with the np.empty_like(arr)
function, stores the shifted elements, and prints the shifted array.
NumPy Shift Array With shift()
Function Inside the scipy.ndimage.interpolation
Library in Python
The shift()
method inside the scipy.ndimage.interpolation
library is used to shift the array using the spline interpolation method in Python. Unlike the numpy.roll()
method, the shift()
method can shift the array and replace the shifted indices with a specified constant value simultaneously. The shift()
function takes the original array, the number of indices we want to shift, and the constant value we want to replace with the shifted indices as input parameters and returns the shifted array where each shifted index is replaced with the specified constant value. The following code example shows us how to shift the elements of an array with the shift()
function.
import numpy as np
from scipy.ndimage.interpolation import shift
array = np.array([1, 2, 3, 4, 5])
result = shift(array, 3, cval=0)
print(result)
Output:
[0 0 0 1 2]
We shifted the elements of array
three places towards the right and replaced the shifted indices with 0
using the shift()
function in the above code. We first created our array with the np.array()
function. We then used the shift function to shift the array
to the right side by 3
places and replace the first three indices of the original array with the constant value 0
. We stored the output of the shift()
function inside the result
array. In the end, we printed the values inside the result
array.
All of these methods work just fine. If we only want to shift the values inside the array and do not want to replace the shifted indices with a constant value, we should use the numpy.roll()
function. On the other hand, if we want to replace the shifted indices with a specific constant value, the array slicing method is the fastest method for this operation. The array slicing method is faster, but it is a little more complicated than using the shift()
function in the scipy
library. If we do not care about the performance of our code, we can use the shift()
function for this task.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn