How to Iterate Over Rows of a Numpy Array in Python
-
Use a Nested
for
Loop to Iterate Over Rows of a Numpy Array in Python -
Use a
for
Loop and theflatten()
Function to Iterate Over Rows of a Numpy Array in Python -
Use the
apply_along_axis()
Function to Iterate Over Rows of a Numpy Array in Python -
Iterate Over Rows of a NumPy Array in Python Using
nditer
-
Iterate Over Rows of a NumPy Array in Python Using
enumerate
andndenumerate
- Conclusion
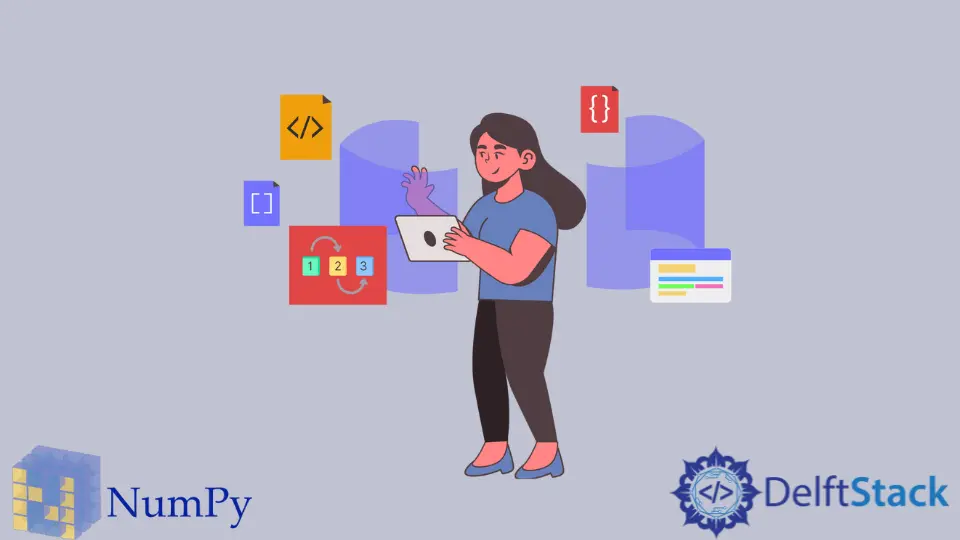
NumPy, a cornerstone library in Python for numerical operations, empowers developers to work seamlessly with arrays, especially in the realm of scientific computing and data manipulation. When dealing with multi-dimensional arrays, the ability to iterate over rows is a fundamental skill.
In this article, we will explore various methods and techniques for efficiently iterating over rows of a NumPy array in Python.
Use a Nested for
Loop to Iterate Over Rows of a Numpy Array in Python
To start, we import the NumPy library as np
and create a 2D NumPy array named my_array
using the np.array
function. This array, denoted by square brackets, represents a matrix with three rows and three columns.
import numpy as np
# Create a 2D NumPy array
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
Iterating over rows of a NumPy array using a for
loop is a common and intuitive approach. The for
loop allows us to iterate over each row effortlessly.
In the provided example, we use a for
loop to iterate over each row of the my_array
and print the contents of each row.
import numpy as np
# Create a 2D NumPy array
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Iterate over rows using a for loop
for row in my_array:
print(row)
The for
loop iterates over each row of the array, and at each iteration, the variable row
represents a single row of the array. The print(row)
statement outputs the contents of each row.
The example output demonstrates the simplicity of using a for
loop for iteration.
[1 2 3]
[4 5 6]
[7 8 9]
Use a for
Loop and the flatten()
Function to Iterate Over Rows of a Numpy Array in Python
Combining a for
loop with the flatten()
function offers an alternative approach to iterating over rows of a NumPy array.
The flatten()
function in NumPy is a method that returns a one-dimensional copy of an array, collapsing all dimensions. This is particularly useful when you want to iterate over the rows of a 2D array using a for
loop.
Here is the syntax for the flatten()
function:
numpy_array.flatten(order="C")
numpy_array
: The array to be flattened.order
: (Optional) The order in which the array elements are flattened. It can beC
(row-major, the default) orF
(column-major).
Let’s see an example:
import numpy as np
# Create a 2D NumPy array
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Iterate over rows using a for loop and flatten()
for cell in my_array.flatten():
print(cell, end=" ")
Output:
1 2 3 4 5 6 7 8 9
First off, we create a 2D array called my_array
using the np.array()
function. This array contains values organized in a matrix with three rows and three columns.
We want to go through each element in the array but in a more sequential way. So, we use a for
loop to iterate over the elements of the array after applying the flatten()
function to it.
The flatten()
function essentially transforms our 2D array into a simpler, one-dimensional array, making it easier to loop through.
Inside the loop, for each iteration, the variable cell
takes on the value of the current element. Then, we print out that element, followed by a space.
The end=" "
part in the print
statement ensures that the elements are printed on the same line with a space in between. So, when we run this code, we get a neat line of all the elements from our 2D array, making it a handy way to iterate over the rows using a for loop and the flatten()
function.
Use the apply_along_axis()
Function to Iterate Over Rows of a Numpy Array in Python
The apply_along_axis()
function in NumPy provides another convenient way to apply a function along the specified axis of an array, making it an effective tool for row-wise operations.
Its syntax is as follows:
numpy.apply_along_axis(func1d, axis, arr, *args, **kwargs)
Parameters:
func1d
: The function to be applied along the specified axis.axis
: The axis along which the function will be applied (0
for columns,1
for rows).arr
: The input array.*args
and**kwargs
: Additional arguments and keyword arguments to be passed to the function.
Now, let’s dive into an example to demonstrate how to use apply_along_axis()
for iterating over rows in a NumPy array:
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
def row_operation(row):
return row.sum()
result = np.apply_along_axis(row_operation, axis=1, arr=my_array)
print("Result of row-wise operation:", result)
Output:
Result of row-wise operation: [ 6 15 24]
In this example, we create a 2D NumPy array named my_array
. We then define a function row_operation()
that takes a row as input and performs a specific operation on that row.
In this case, it calculates the sum of the elements in each row.
Next, we use the apply_along_axis()
function, specifying the row_operation
function, setting axis=1
to indicate row-wise application, and providing our array my_array
as the input.
The result is an array containing the output of the row_operation
function applied to each row of my_array
. Finally, we print the result, showcasing how apply_along_axis()
efficiently iterates over rows.
Iterate Over Rows of a NumPy Array in Python Using nditer
The nditer
function is a part of the NumPy library and is designed to efficiently iterate over elements in an array. It offers a variety of methods and options to customize the iteration process.
The syntax of nditer
is as follows:
numpy.nditer(
op,
flags=["readwrite"],
op_flags=None,
op_dtypes=None,
order="K",
casting="safe",
buffersize=0,
)
Parameters:
op
: The array to be iterated.flags
: A list of flags modifying the behavior of the iterator (default isreadwrite
).op_flags
: Additional flags for each operand (if applicable).op_dtypes
: A list of operand data types (if applicable).order
: The order in which the iterator should traverse the array (C
for C-style,F
for Fortran-style,K
for keep order).casting
: Specifies the casting rule for implicit type casting.buffersize
: Size of the buffer for optimization.
Now, let’s illustrate how to use nditer
to iterate over rows in a NumPy array with a practical example:
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
with np.nditer(my_array, flags=["multi_index"], op_flags=["readwrite"]) as it:
for row_index in it:
row_data = my_array[it.multi_index[0], :]
print(f"Row {it.multi_index[0] + 1}: {row_data}")
Output:
Row 1: [1 2 3]
Row 1: [1 2 3]
Row 1: [1 2 3]
Row 2: [4 5 6]
Row 2: [4 5 6]
Row 2: [4 5 6]
Row 3: [7 8 9]
Row 3: [7 8 9]
Row 3: [7 8 9]
In this example, we first create a 2D NumPy array named my_array
. To iterate over rows, we use the nditer
function with the 'multi_index'
flag, indicating that we want to track the multi-index during iteration.
Inside the loop, the it.multi_index[0]
gives us the current row index, and we use this index to access the elements of the array along that row.
In this case, row_data
represents the elements of the current row.
You can perform any row-wise operation within the loop, making nditer
a versatile tool for customizing the iteration process based on your specific needs.
Iterate Over Rows of a NumPy Array in Python Using enumerate
and ndenumerate
In addition to the methods discussed above, the built-in enumerate
and ndenumerate
functions, in combination with a for
loop, can also be used for iterating over rows in a NumPy array.
Using enumerate
With a for
Loop
The enumerate
function in Python is a versatile tool for obtaining both the index and value of elements in an iterable. When combined with a for
loop, it becomes a handy way to iterate over rows in a NumPy array.
Here’s an example:
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
for row_index, row_data in enumerate(my_array):
print(f"Row {row_index + 1}: {row_data}")
Output:
Row 1: [1 2 3]
Row 2: [4 5 6]
Row 3: [7 8 9]
In this example, enumerate(my_array)
returns pairs of row indices (row_index
) and corresponding row data (row_data
). The for
loop then iterates over these pairs, allowing us to access and print each row along with its index.
Using ndenumerate
With a for
Loop
NumPy provides the ndenumerate
function, specifically designed for iterating over elements and their indices in multi-dimensional arrays. Here’s how you can use it to iterate over rows:
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
for index, value in np.ndenumerate(my_array):
row_index = index[0]
if index[1] == 0:
print(f"Row {row_index + 1}: {my_array[row_index, :]}", end=" ")
Output:
Output:
Row 1: [1 2 3] Row 2: [4 5 6] Row 3: [7 8 9]
In this example, np.ndenumerate(my_array)
returns pairs of indices (index
) and corresponding values (value
). The loop iterates over these pairs, and we use the first index (index[0]
) to identify the row index.
We print the value, ensuring it’s in the values of each row by checking if the second index (index[1]
) is 0
.
Both methods offer effective ways to iterate over rows in a NumPy array. The enumerate
method provides a concise and Pythonic approach, while ndenumerate
from NumPy provides more control over the iteration process, especially in multi-dimensional arrays.
Conclusion
Iterating over rows of a NumPy array is a fundamental skill for anyone working with data in Python. The methods discussed in this article provide a comprehensive toolkit for you to choose from based on your specific needs.
Whether it’s the simplicity of a for
loop, the efficiency of nditer
, or the flexibility of enumerate
and ndenumerate
, mastering these techniques will undoubtedly enhance your proficiency in array manipulation and contribute to the development of robust Python applications.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn