How to Convert 3D Array to 2D Array in Python
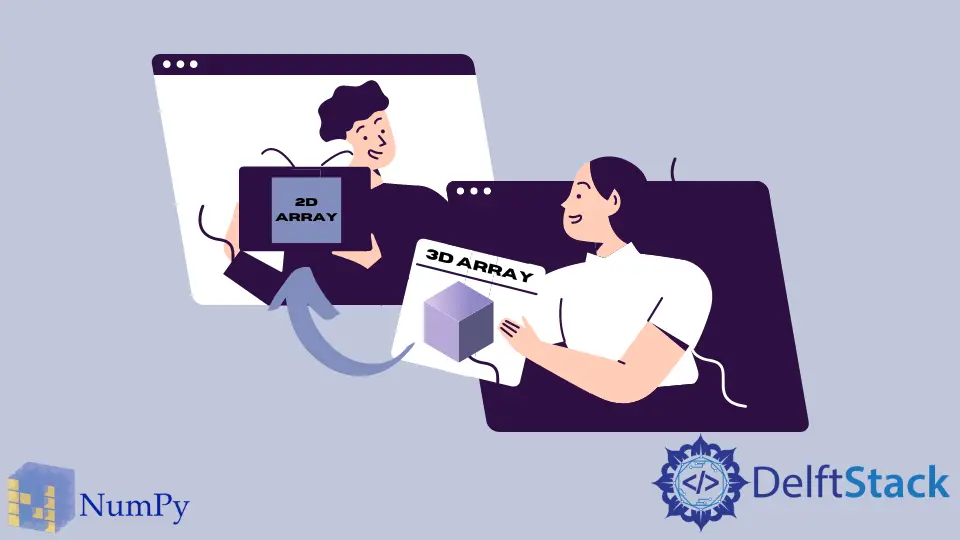
In this tutorial, we will discuss converting a 3D array to a 2D array in Python.
Convert a 3D Array to a 2D Array With the numpy.reshape()
Function in Python
The numpy.reshape()
function changes the shape of an array without changing its data. numpy.reshape()
returns an array with the specified dimensions. For example, if we have a 3D array with dimensions (4, 2, 2)
and we want to convert it to a 2D array with dimensions (4, 4)
.
The following code example shows us how we can use the numpy.reshape()
function to convert a 3D array with dimensions (4, 2, 2)
to a 2D array with dimensions (4, 4)
in Python.
import numpy
arr = numpy.array(
[[[0, 1], [2, 3]], [[4, 5], [6, 7]], [[8, 9], [10, 11]], [[12, 13], [14, 15]]]
)
newarr = arr.reshape(4, 2 * 2)
print(newarr)
Output:
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]]
In the above code, we first initialize a 3D array arr
using numpy.array()
function and then convert it into a 2D array newarr
with numpy.reshape()
function.
The following code example shows another way of doing the same thing if, for some reason, we do not know the exact dimensions of the 3D array.
import numpy
arr = numpy.array(
[[[0, 1], [2, 3]], [[4, 5], [6, 7]], [[8, 9], [10, 11]], [[12, 13], [14, 15]]]
)
newarr = arr.reshape(arr.shape[0], (arr.shape[1] * arr.shape[2]))
print(newarr)
Output:
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]]
In the above code, we use the numpy.shape()
function to specify the dimensions of the newarr
. The numpy.shape()
function returns a tuple that contains the number of elements in each dimension of an array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn