How to Write NumPy Array to CSV in Python
-
Method 1: Using NumPy’s
savetxt
Function - Method 2: Using Pandas to Write NumPy Array to CSV
- Method 3: Using the CSV Module in Python
- Conclusion
- FAQ
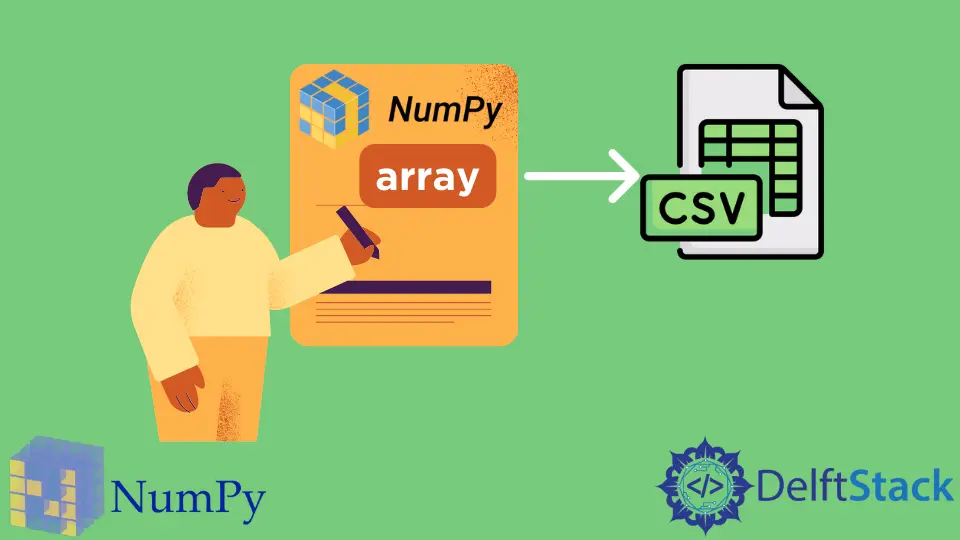
Saving data efficiently is crucial in data science and programming. One common task is exporting data from a NumPy array to a CSV file. CSV (Comma Separated Values) files are widely used for data storage because they are simple and easy to read.
In this tutorial, we will explore how to write a NumPy array to a CSV file using Python. Whether you are a beginner or an experienced programmer, this guide will provide you with clear examples and explanations to help you master this essential skill. So, let’s dive in and learn how to save your NumPy arrays to CSV files seamlessly.
Method 1: Using NumPy’s savetxt
Function
One of the simplest ways to write a NumPy array to a CSV file is by using the savetxt
function provided by the NumPy library. This function is versatile and can handle a variety of array types. Here’s a straightforward example of how to use it.
import numpy as np
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
np.savetxt('output.csv', data, delimiter=',', fmt='%d')
Output:
1,2,3
4,5,6
7,8,9
In this example, we first import the NumPy library and create a 2D NumPy array named data
. The savetxt
function is then called with three parameters: the name of the output file (‘output.csv’), the array we want to save, and the delimiter we want to use (in this case, a comma). The fmt
parameter specifies the format of the data; here, we use %d
for integers. This method is efficient and easy to implement, making it a go-to choice for many developers.
Method 2: Using Pandas to Write NumPy Array to CSV
Another powerful approach to writing a NumPy array to a CSV file is by using the Pandas library. Pandas is designed for data manipulation and analysis, and it provides a convenient way to handle CSV files. Here’s how you can do it.
import numpy as np
import pandas as pd
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
df = pd.DataFrame(data)
df.to_csv('output_pandas.csv', index=False, header=False)
Output:
1,2,3
4,5,6
7,8,9
In this method, we start by importing both NumPy and Pandas. We create a 2D NumPy array similar to the previous example. Next, we convert this array into a Pandas DataFrame, which is a powerful data structure that allows for easy manipulation of data. Finally, we use the to_csv
method to write the DataFrame to a CSV file. The parameters index=False
and header=False
ensure that we do not write the index or header to the CSV file, keeping the output clean and straightforward. This method is particularly useful when you need to perform additional data manipulation before saving your array.
Method 3: Using the CSV Module in Python
If you prefer a more manual approach, you can use Python’s built-in CSV module to write a NumPy array to a CSV file. This method gives you more control over the writing process and is useful if you need to customize the output significantly.
import numpy as np
import csv
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
with open('output_csv_module.csv', mode='w', newline='') as file:
writer = csv.writer(file)
for row in data:
writer.writerow(row)
Output:
1,2,3
4,5,6
7,8,9
In this example, we import both NumPy and the CSV module. We create a 2D NumPy array as before. The open
function is used to create a new CSV file, and we specify the mode as ‘w’ for writing. The csv.writer
function initializes a writer object that we can use to write rows to the file. We then loop through each row of the NumPy array and use the writer.writerow
method to write it to the CSV file. This method is particularly advantageous if you need to customize the output format or include additional processing for each row.
Conclusion
Exporting NumPy arrays to CSV files is a fundamental skill for anyone working with data in Python. Whether you choose to use NumPy’s built-in functions, the Pandas library, or the CSV module, each method has its advantages and can be tailored to suit your specific needs. With the examples and explanations provided in this tutorial, you should now feel confident in your ability to save NumPy arrays to CSV files effectively. Happy coding!
FAQ
-
Can I write a 3D NumPy array to a CSV file?
Writing a 3D array directly to a CSV file is not straightforward since CSV files are inherently 2D. You would need to reshape or flatten the array before saving it. -
What if my NumPy array contains non-numeric data?
If your array contains non-numeric data, you can still use the methods mentioned. Just ensure that the data types are compatible with the CSV format.
-
Is there a way to append data to an existing CSV file?
Yes, you can open the file in append mode (‘a’) in Python and write additional rows without overwriting the existing data. -
What delimiter can I use besides a comma?
While commas are the standard delimiter for CSV files, you can use other delimiters like semicolons or tabs. Just specify the delimiter parameter accordingly. -
Do I need to install any additional libraries to use Pandas?
Yes, you need to install the Pandas library separately if it’s not already included in your Python environment. You can do this using pip: pip install pandas.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn