在 Python 中將 NumPy 陣列寫入 CSV
-
使用
pandas
資料框將 NumPy 陣列儲存在 CSV 檔案中 -
使用
numpy.savetxt()
函式將 NumPy 陣列儲存到 CSV 檔案中 -
使用
tofile()
函式將 NumPy 陣列儲存到 CSV 檔案中 - 使用檔案處理方法將 NumPy 陣列儲存在 CSV 檔案中
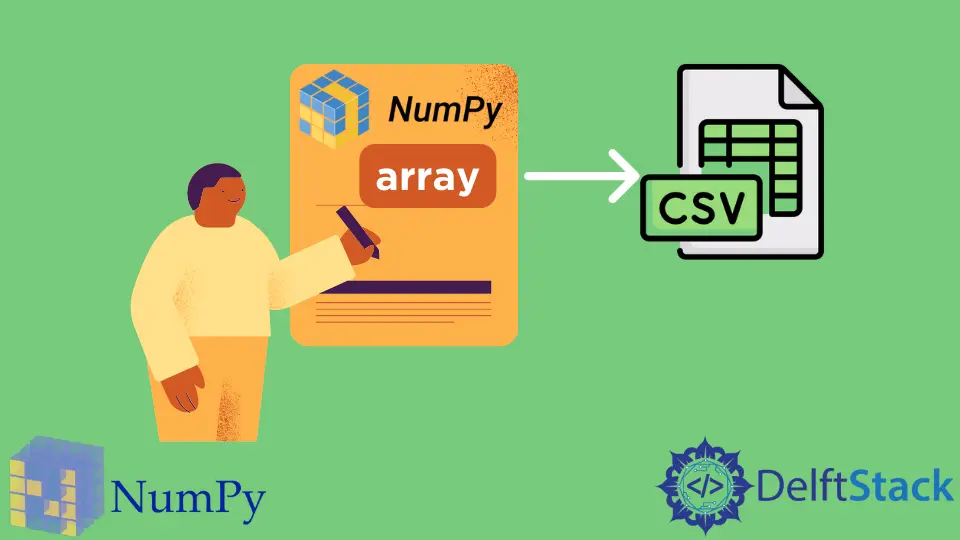
在本教程中,我們將討論如何將 numpy 陣列儲存在 CSV 檔案中。
使用 pandas
資料框將 NumPy 陣列儲存在 CSV 檔案中
在這種方法中,我們將首先將陣列儲存在 pandas
DataFrame 中,然後將其轉換為 CSV 檔案。
以下程式碼顯示了我們如何實現這一目標。
import pandas as pd
import numpy as np
a = np.asarray([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
pd.DataFrame(a).to_csv("sample.csv")
pd.DataFrame
函式將陣列儲存在 DataFrame 中,我們只需使用 to_csv()
函式將其匯出到 CSV 檔案即可。
使用 numpy.savetxt()
函式將 NumPy 陣列儲存到 CSV 檔案中
numpy 模組中的 savetxt()
函式可以將陣列儲存到文字檔案。我們可以指定檔案格式,定界符和許多其他引數,以獲得所需格式的最終結果。
在下面的程式碼中,我們使用此函式將陣列儲存在 CSV 檔案中。
import numpy as np
a = np.asarray([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
np.savetxt("sample.csv", a, delimiter=",")
使用 tofile()
函式將 NumPy 陣列儲存到 CSV 檔案中
tofile()
函式允許我們將陣列寫入文字或二進位制檔案。但是,這種方法有許多缺點。它更像是一種便捷功能,用於快速儲存陣列資料。資訊的準確性會丟失,因為它會將所有內容儲存在一行中,因此對於打算用於存檔資料的檔案來說,此方法不是一個好的選擇。這些問題中的一些可以通過將資料作為文字檔案輸出而以速度和檔案大小為代價來克服。
以下程式碼演示了此函式的用法。
import numpy as np
a = np.asarray([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
a.tofile("sample.csv", sep=",")
使用檔案處理方法將 NumPy 陣列儲存在 CSV 檔案中
我們可以使用傳統的檔案處理方法,但是不建議使用它們,因為此類方法需要根據陣列的形狀進行許多修改,並且會佔用大量記憶體。
以下程式碼顯示了此方法的示例。
a = np.asarray([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
csv_rows = ["{},{},{}".format(i, j, k) for i, j, k in a]
csv_text = "\n".join(csv_rows)
with open("sample.csv", "w") as f:
f.write(csv_text)
我們將陣列解壓縮為一個行列表,然後通過使用 join()
函式加入該列表來返回單個字串。然後,我們將此字串寫入 CSV 檔案。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn