How to Remove Last Element From Array in JavaScript
-
Use the
array.prototype.splice()
to Remove the Last Element From an Array JavaScript -
Use the
array.prototype.pop()
to Remove the Last Element From an Array JavaScript -
Use the
array.prototype.slice()
to Remove the Last Element From an Array in JavaScript -
Use the
array.prototype.filter()
to Remove the Last Element From an Array in JavaScript - Use a User-Defined Method to Remove the Last Element From an Array in JavaScript
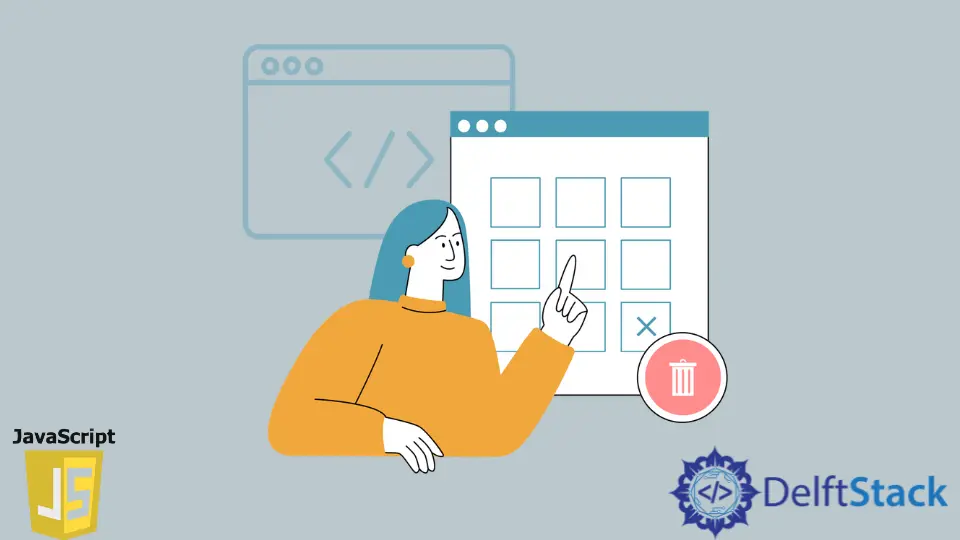
An array stores every element at a particular index. The index for the elements starts from 0 to the array’s length minus 1.
In this tutorial, different methods are discussed for removing the last element from an array in JavaScript.
Use the array.prototype.splice()
to Remove the Last Element From an Array JavaScript
The splice()
method is used to change the array by removing or replacing the elements. If any element is deleted, then it returns the deleted elements. Otherwise, an empty array is returned.
We can use it to remove the last element from an array.
Check the code below.
var array = [10, 11, 12, 13, 14, 15, 16, 17];
array.splice(-1, 1);
console.log(array);
Output:
[10, 11, 12, 13, 14, 15, 16]
Use the array.prototype.pop()
to Remove the Last Element From an Array JavaScript
The pop()
function is the most straightforward way to achieve this. It removes the last element from an array and returns the deleted element.
For example,
var array = [10, 11, 12, 13, 14, 15, 16, 17];
array.pop();
console.log(array)
Output:
[10, 11, 12, 13, 14, 15, 16]
Use the array.prototype.slice()
to Remove the Last Element From an Array in JavaScript
The slice()
function creates a copy of the defined part selected from the start
to the stop
(the end is not included). The start
and stop
values are the indices of the array. It returns the new array.
Check the code below.
var array = [10, 11, 12, 13, 14, 15, 16, 17];
array = array.slice(0, -1);
console.log(array);
Output:
[10, 11, 12, 13, 14, 15, 16]
Use the array.prototype.filter()
to Remove the Last Element From an Array in JavaScript
The filter()
method returns the modified array according to the passed provided function.
To remove the last element using this function, see the code below.
var array = [10, 11, 12, 13, 14, 15, 16, 17];
array = array.filter((element, index) => index < array.length - 1);
console.log(array);
Output:
[10, 11, 12, 13, 14, 15, 16]
Use a User-Defined Method to Remove the Last Element From an Array in JavaScript
We can create a new function that takes the array as an input and reduces the array’s length if the length of the array is greater than zero.
We implement this in the following example.
var array = [10, 11, 12, 13, 14, 15, 16, 17];
function removeElement(arr) {
if (arr.length > 0) arr.length--;
return arr
};
var newArray = removeElement(array);
console.log(newArray);
Output:
[10, 11, 12, 13, 14, 15, 16]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript