Java Optional Parameters
- Use Method Overloading to Have Optional Parameters in Java
-
Use the
Optional
Container Object to Have Optional Parameters in Java -
Use
Build Pattern
to Have Optional Parameters in Java -
Use
Varargs
to Have Optional Parameters in Java
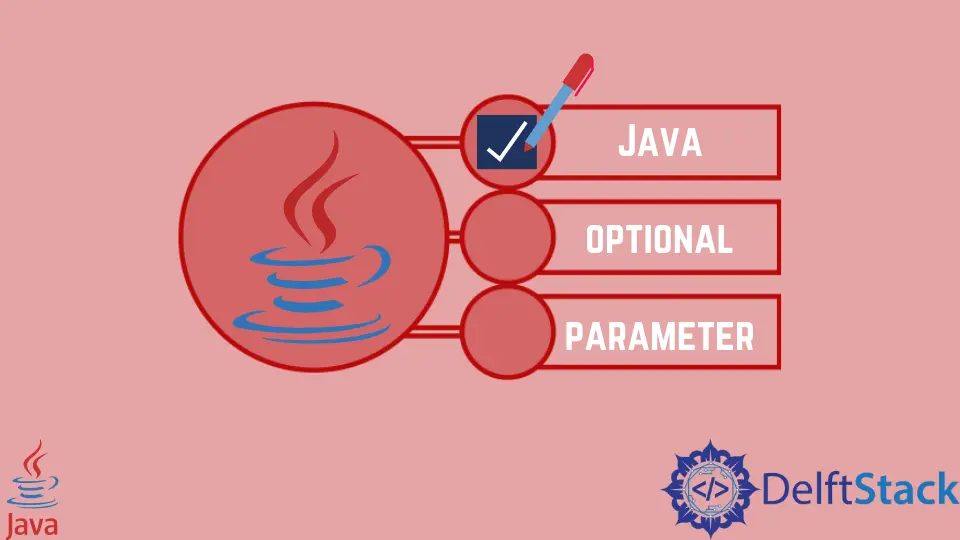
In Java class, when we design a method or function, some arguments may be optional for its execution. There are no optional parameters in Java, but we can simulate optional parameters using the following techniques discussed in this article.
Use Method Overloading to Have Optional Parameters in Java
If a class has multiple methods that have the same name but takes different parameters, it is called method Overloading. Here we have a class, Multiply
, which has two methods with the same name mul
. The arguments passed to the methods are different one takes two int type arguments while the other takes three.
class Multiply {
static int mul(int a, int b) {
return a * b;
}
static int mul(int a, int b, int c) {
return a * b * c;
}
}
class TestOverloading {
public static void main(String[] args) {
System.out.println(Multiply.mul(2, 11));
System.out.println(Multiply.mul(2, 2, 11));
}
}
Output:
22
44
Use the Optional
Container Object to Have Optional Parameters in Java
In Java, Optional
is a container object that may or may not contain a non-null value. If a value is present, then the isPresent()
method will return true, and the get()
method will return the value.
In case if we expect some null values, we can use the ofNullable()
method of this class. It returns an empty Optional
object and does not throw an exception. Here the variable lastName
is passed null for the Student
class object, so we created an Optional
object that may contain a null value.
The method isPresent()
checks if the value is present; otherwise, the default string is assigned to it, as shown in the code below.
import java.util.Optional;
public class Main {
public static void main(String args[]) {
Student("John", null, 25);
}
private static void Student(String name, String lastName, int age) {
Optional<String> ln = Optional.ofNullable(lastName);
String a = ln.isPresent() ? ln.get() : "Not Given";
System.out.println("name : " + name + ", lastname : " + a + ", age : " + age);
}
}
Output:
name : John, lastname : Not Given, age : 25
Use Build Pattern
to Have Optional Parameters in Java
The purpose of the Build Pattern
is to separate the complex object construction from its representation. The logic and default configuration values required to create an object are enclosed in the builder
class.
Here in the code, we use an additional class, UserBuilder
, which encloses all necessary properties and a combination of optional parameters for building a User
object without losing immutability. In the User
class, there are only getters and no setters to preserve immutability.
public class User {
private final String firstName; // required
private final String lastName; // required
private final int age; // optional
private final String email; // optional
private User(UserBuilder builder) {
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.age = builder.age;
this.email = builder.email;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public int getAge() {
return age;
}
public String getEmail() {
return email;
}
@Override
public String toString() {
return "User: " + this.firstName + ", " + this.lastName + ", " + this.age + ", " + this.email;
}
public static class UserBuilder {
private final String firstName;
private final String lastName;
private int age;
private String email;
public UserBuilder(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public UserBuilder age(int age) {
this.age = age;
return this;
}
public UserBuilder email(String email) {
this.email = email;
return this;
}
public User build() {
User user = new User(this);
return user;
}
}
}
In the TestUser
class, we utilize the UserBuilder
to create the User
class object. For the user1
object, we pass all attributes, while for the user2
object, we don’t pass the age
and email
arguments. Thus it takes default values for optional parameters. The build()
method constructs the User
object and returns it.
public class TestUser {
public static void main(String args[]) {
User user1 = new User.UserBuilder("John", "Doe").age(24).email("john.doe@dex.com").build();
System.out.println(user1);
User user2 = new User.UserBuilder("Jack", "Luther").build();
System.out.println(user2);
}
}
Output:
User: John, Doe, 24, john.doe@dex.com
User: Jack, Luther, 0, null
Use Varargs
to Have Optional Parameters in Java
In Java, Varargs
(variable-length arguments
) allows the method to accept zero or multiple arguments. The use of this particular approach is not recommended as it creates maintenance problems.
Here in the code below, we have the VarargsExample
class that has a method display()
. This method takes an arbitrary number of parameters of the String
type. Few rules to keep in mind while using varargs
is that each method can have only one varargs
parameter, and it must be the last parameter.
public class VarArgsCheck {
public static void main(String args[]) {
VarargsExample.display();
VarargsExample.display("here", "are", "the", "varargs", "to", "check");
}
}
class VarargsExample {
static void display(String... values) {
String arr[] = values;
System.out.println("display method invoked ");
System.out.println("Value length " + arr.length);
}
}
Output:
display method invoked
Value length 0
display method invoked
Value length 6
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn