How to Concatenate Lists in Java
-
List Concatenation Using the
cacat()
Method in Java 8 -
List Concatenation Using the
Stream.of()
Method in Java 8
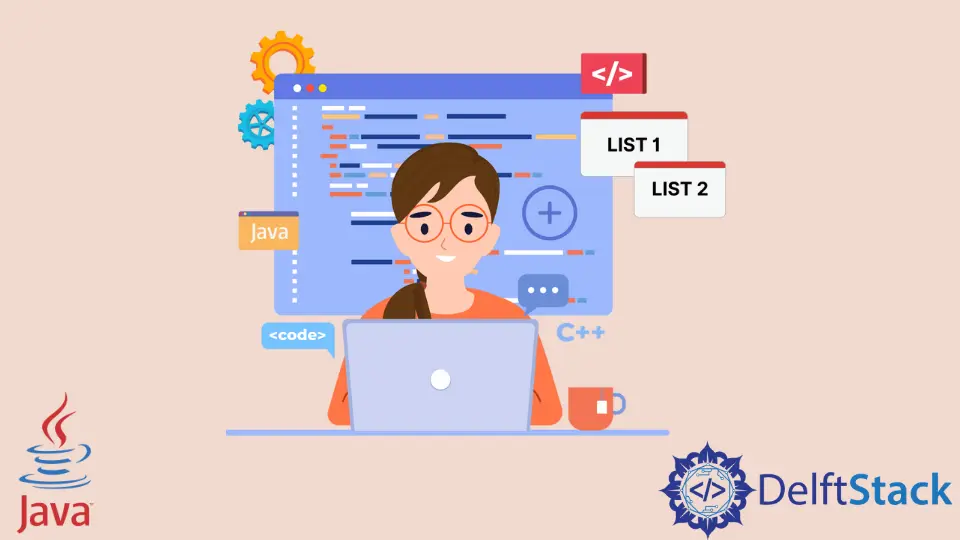
An ordered collection of elements that can increase dynamically is known as the List
collection. It gets represented as a node
element, and each node contains a reference
to the next node and the element. The operations we can perform over a list collection include element traversal, insertion, updating, and deleting using the indexes.
Java provides a List
interface that offers various methods for its functioning. The multiple implementations of the list include ArrayList
,LinkedList
, and SortedList
. There are different properties of the list that can:
- Become duplicate values in the list.
- Store null elements.
- Grow dynamically, unlike arrays whose size is definite.
The code below illustrates how you can create a Java concatenate list:
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class ListConcatenation {
public static void main(String[] args) {
List<String> birds_list =
Stream.of("pigeon", "crow", "squirrel").collect(Collectors.toList()); // Java 8
List<String> animal_list = List.of("cat", "dog", "rabbit"); // Java 9
System.out.println("Way 1");
List<String> stringArrayList = new ArrayList<String>(); // Java7
stringArrayList.addAll(birds_list);
stringArrayList.addAll(animal_list);
System.out.println(stringArrayList);
System.out.println("Way 2");
List<String> newList =
Stream.concat(birds_list.stream(), animal_list.stream()).collect(Collectors.toList());
System.out.println(newList);
System.out.println("Way 3");
List<String> newList2 =
Stream.of(birds_list, animal_list).flatMap(Collection::stream).collect(Collectors.toList());
System.out.println(newList2);
}
}
In the code block above, the first two lines initialize the lists that we want to concatenate.
The first way of list initialization is to give elements at the time of declaration itself. So as per the Java 8
methodology, the Stream
interface gets used. Streams act as a wrapper to the data source and provide methods to operate and process the data. The of
static method takes and creates the sequential stream of provided elements as parameters and returns the element stream. The chain of operations that we perform is in Java 8. The collect
method is a terminal operation that collects the stream
provided after reduction.
Another way of initializing the list is using the Java 9
version. It provides a convenient way of initializing a list using the List.of
static factory method that creates Immutable Lists.
Finally, a combined List
object gets initialized traditionally using the Java 7
version. The addAll()
method gets invoked using the newList
instance. The method appends the specified collection to the identified collection at its end. It returns true
when appending the list is successful. The method can throw NullPointerException
when the passed list collection is null.
The combined list instance now contains the birds_list
collection. Similarly the animal_list
instance is added to stringArrayList
. And finally, after printing the list, it creates a sequential collection appended one after another.
Below is the console output of the code block:
Way 1 [pigeon, crow, squirrel, cat, dog, rabbit] Way 2 [pigeon, crow, squirrel, cat, dog,
rabbit] Way 3 [pigeon, crow, squirrel, cat, dog, rabbit]
List Concatenation Using the cacat()
Method in Java 8
The illustration uses the same code block to show the concat()
method - it’s static in the Stream
interface. It is considered an intermediate
operation or lazy initialization as it operates when a terminal operation gets applied over the function. The concat()
method takes two streams that are to concatenate. And it returns the combined list from the two streams that we pass as parameters. Over this method, the collect
function gets invoked to convert the stream in the desired format. The function takes the collector as an argument, and in our case, the Collectors.toList()
function is passed to reduce the stream in the List
type.
The output would be similar to the first code block and gets printed in the above console log under the Way 2
statement.
List Concatenation Using the Stream.of()
Method in Java 8
As mentioned above, of
is a static method in the Stream
interface that takes elements. So these elements can be primitive datatypes or collections
classes. The collections of birds and animals pass as arguments. Now, the flatMap
method gets invoked in the chain of calls, and the use is to perform processing and transform the stream passed.
The flatMap
method takes a Function
as its parameter. The method applies transformations over the elements and flattens the result. In this case, Collection::stream
is passed to convert the elements in a single stream, which gets returned after processing. The stream is now collected using the collect
method.
The output is the same as the console block above with the heading as Way 3
.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn