How to Create an Object From Interface in TypeScript
- Assign All Fields in the Created Object in TypeScript
-
Use the
as
Keyword to Set an Empty Object in TypeScript -
Use the
as
Keyword to Set Only the Required Attributes in TypeScript -
Use the
Partial
,Omit
, andPick
Types to Create an Object in TypeScript -
Use the
?
Operator to Make Attributes in the Interface Optional in TypeScript
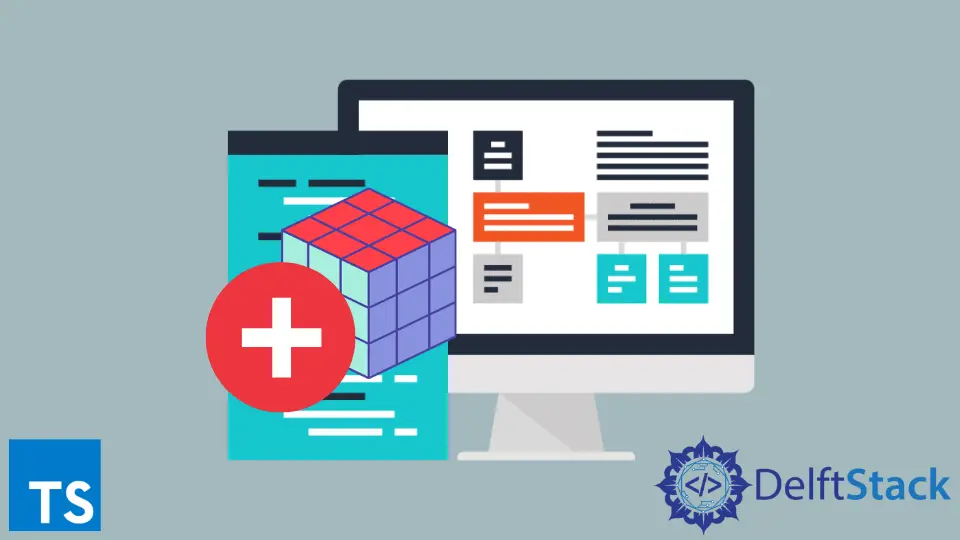
Interfaces in TypeScript provide a construct for strict typing support compared to plain JavaScript. The user may design interfaces or be present in a third-party library imported by the user.
Most of the time, the user wants to create an object based on the interface definition provided in the third-party library to access the methods and functionality provided by the third-party library.
This article will focus on creating an object from an interface definition.
Assign All Fields in the Created Object in TypeScript
All the properties associated with the interface definition can be directly assigned to the newly created object. The following code segment demonstrates this.
interface Animal {
legs : number ;
eyes : number ;
name : string ;
wild : boolean ;
};
const dog : Animal = {
legs : 4,
eyes : 2,
name : 'Dog',
wild : false
};
The properties can now be accessed from the object like dog.name
or dog.wild
.
Use the as
Keyword to Set an Empty Object in TypeScript
An empty object can be initialized using the as
keyword, whose attributes can be set later. The following code segment demonstrates this.
interface Animal {
legs : number ;
eyes : number ;
name : string ;
wild : boolean ;
};
const dog : Animal = {} as Animal;
dog.legs = 4;
dog.name = "Dog";
console.log(dog);
Output:
{
"legs": 4,
"name": "Dog"
}
Thus an empty object was initialized of the type Animal
, and the attributes legs
and name
were set. When the object was printed, it only showed the set attributes.
Use the as
Keyword to Set Only the Required Attributes in TypeScript
The required attributes can be set directly using the as
keyword. The as
keyword forces the compiler to recognize the object is of a certain type even if all the fields in the object have not been set.
interface Animal {
legs : number ;
eyes : number ;
name : string ;
wild : boolean ;
};
const dog : Animal = {
legs : 4,
name : 'Dog',
} as Animal;
Use the Partial
, Omit
, and Pick
Types to Create an Object in TypeScript
The Partial
type is used to make all attributes of an interface optional. The Pick
type is used when only certain interface attributes are required to create the object.
The Omit
type is used as the inverse of the Pick
type - to remove certain attributes from the interface while keeping all the other attributes as required.
interface Animal {
legs : number ;
eyes : number ;
name : string ;
wild : boolean ;
};
const dogOnlyWithName : Pick<Animal, 'name'> = {
name : "Dog"
};
const dogWithoutWildFlagAndEyes : Omit<Animal, "wild" | "eyes"> = {
legs : 4,
name : "Dog"
}
const dogWithAllOptionalTypes : Partial<Animal> = {
eyes: 2
};
Use the ?
Operator to Make Attributes in the Interface Optional in TypeScript
Some of the attributes in the interface can be marked optional by the ?
operator. This is a viable option in the case of user-defined interfaces but cannot be implemented in the case of interfaces imported from third-party libraries.
interface Animal {
legs : number ;
eyes? : number ;
name : string ;
wild? : boolean ;
};
const dog : Animal = {
legs : 4,
name : 'Dog'
};