How to Format String in TypeScript
- Using Template Literals
- String Concatenation
-
String Formatting with
String.prototype.replace()
-
Using the
Intl
API for Internationalization - Conclusion
- FAQ
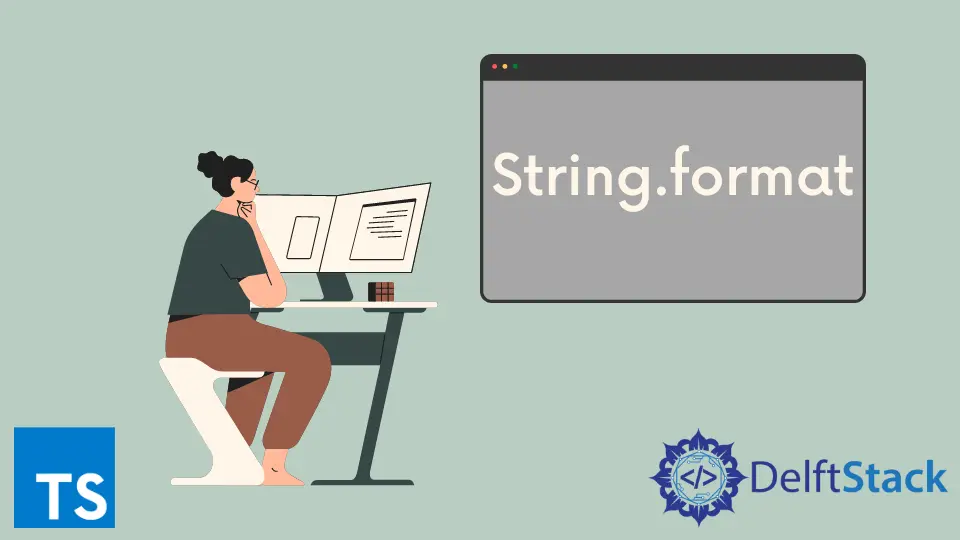
String formatting is a crucial aspect of programming, allowing developers to create dynamic and readable text outputs. In TypeScript, a superset of JavaScript, you have several methods to format strings effectively.
This tutorial demonstrates how to format strings in TypeScript by exploring different techniques. Whether you’re constructing messages, displaying user information, or managing data outputs, understanding string formatting can significantly enhance your code’s clarity and functionality. Let’s dive into the various methods available for formatting strings in TypeScript, ensuring that your code is not only functional but also elegant.
Using Template Literals
One of the most popular and modern ways to format strings in TypeScript is through template literals. Template literals are enclosed by backticks (`
) and can include embedded expressions, making string interpolation straightforward and intuitive.
Here’s a simple example:
const name = "John";
const age = 30;
const greeting = `Hello, my name is ${name} and I am ${age} years old.`;
console.log(greeting);
Output:
Hello, my name is John and I am 30 years old.
In this example, we define two variables: name
and age
. By using template literals, we can seamlessly embed these variables within a string. This method not only enhances readability but also reduces the likelihood of errors compared to traditional string concatenation. You can even include expressions within the curly braces, making it versatile for various scenarios.
String Concatenation
Another method for formatting strings in TypeScript is string concatenation. This approach involves using the +
operator to join multiple strings together. While it may seem straightforward, it can become cumbersome, especially with longer strings or multiple variables.
Here’s how you can use string concatenation:
const firstName = "Jane";
const lastName = "Doe";
const fullName = firstName + " " + lastName;
console.log("Full Name: " + fullName);
Output:
Full Name: Jane Doe
In this example, we concatenate the firstName
and lastName
variables to create a fullName
. Although this method works perfectly, it can lead to less readable code, particularly when dealing with more complex strings. It’s essential to keep this in mind as your projects grow in size and complexity.
String Formatting with String.prototype.replace()
For more advanced string formatting, you can utilize the replace()
method available on string instances. This method allows you to replace parts of a string with new content, making it useful for dynamic text generation.
Here’s an example of how to use replace()
for string formatting:
const template = "Hello, {name}. Welcome to {place}.";
const formattedString = template
.replace("{name}", "Alice")
.replace("{place}", "Wonderland");
console.log(formattedString);
Output:
Hello, Alice. Welcome to Wonderland.
In this snippet, we define a template string that includes placeholders. By using the replace()
method, we can substitute the placeholders with actual values. This method is particularly beneficial when you have a template that needs to be reused with different data, enhancing maintainability and reducing redundancy.
Using the Intl
API for Internationalization
If you are working on applications that require internationalization, the Intl
API in TypeScript provides powerful options for formatting strings. This API allows you to format numbers, dates, and currencies according to the user’s locale, making it an essential tool for global applications.
Here’s a brief example of how to format a number using the Intl.NumberFormat
class:
const number = 1234567.89;
const formattedNumber = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD',
}).format(number);
console.log(formattedNumber);
Output:
$1,234,567.89
In this code, we format a number as currency using the Intl.NumberFormat
class. By specifying the locale and options, we can customize how the number appears. This method is invaluable for applications that cater to users from different regions, ensuring that your output is both accurate and culturally appropriate.
Conclusion
Formatting strings in TypeScript can greatly enhance the readability and maintainability of your code. From using template literals for straightforward interpolation to leveraging the Intl
API for internationalization, there are various methods available to meet your formatting needs. By incorporating these techniques into your TypeScript projects, you can create dynamic and user-friendly outputs that resonate with your audience. Remember, the right method often depends on the context of your application, so choose wisely.
FAQ
-
What are template literals in TypeScript?
Template literals are string literals enclosed by backticks that allow for embedded expressions, making string interpolation easier. -
How does string concatenation work in TypeScript?
String concatenation in TypeScript involves using the+
operator to join multiple strings together. -
Can I use the
replace()
method for string formatting?
Yes, thereplace()
method can be used to substitute parts of a string with new content, making it useful for dynamic text generation. -
What is the
Intl
API in TypeScript?
TheIntl
API provides options for formatting numbers, dates, and currencies according to the user’s locale, which is essential for internationalization. -
Which string formatting method is the best?
The best method depends on your specific use case. For simple cases, template literals or concatenation may suffice, while more complex scenarios may benefit from thereplace()
method or theIntl
API.