How to Remove an Array Item in TypeScript
-
Use
splice()
to Remove an Array Item in TypeScript -
Use
shift()
to Remove an Array Item in TypeScript -
Use
pop()
to Remove an Array Item in TypeScript -
Use
delete
Operator to Remove an Array Item in TypeScript
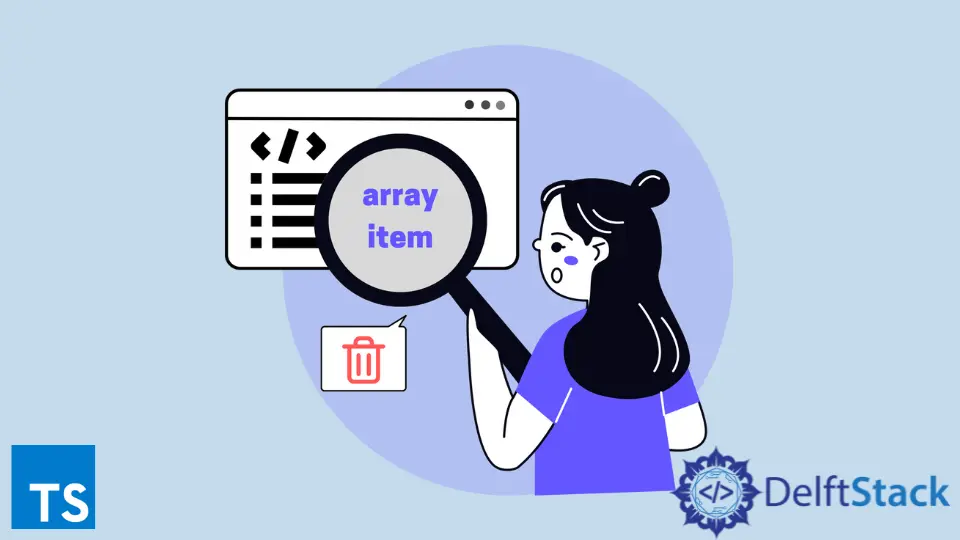
Removing an array item can be achieved using multiple methods in TypeScript. The methods that are used to achieve the above functionalities are splice()
, shift()
, pop()
, and delete
operator.
In this post, we’ll look at several different methods for removing an array item using TypeScript.
Use splice()
to Remove an Array Item in TypeScript
When removing and adding elements in TypeScript, splice()
is the best option. There are two main reasons why splice()
is the best option.
- It does not create a new object, and it removes the item instantly.
- After removing an element, it does not leave the array index as null but rather appropriately updates the array.
Syntax:
array.splice(array_index, no_of_elements, [element1][, ..., elementN]);
array_index
: Specifies where the alteration should begin.no_of_elements
: Specifies the number of elements that should be removed after the specifiedarray_index
.element1
: The element/elements that should be added to the array, if there is/are any.
Example:
let array = ["white", "yellow", "black", "green", "blue"];
let removed = array.splice(3, 1, "red");
console.log("The new array is : " + array );
console.log("The color that was removed is : " + removed);
Output:
The new array is : white,yellow,black,red,blue
The color that was removed is: green
Use shift()
to Remove an Array Item in TypeScript
The shift()
method can delete an element from an array in TypeScript, but its capacity is limited. Using shift()
is only possible to remove the first element of a particular array and return it.
Furthermore, it has no arguments that need to be passed to the function as it only does one task.
Syntax:
array.shift();
Example:
let array = ["white", "yellow", "black", "green", "blue"].shift();
console.log("The removed color is : " + array );
Output:
The removed color is : white
Use pop()
to Remove an Array Item in TypeScript
pop()
has similar functionality as shift()
, but the difference between the two functions is that while shift()
removes the first element of an array, pop()
removes the last element of an array and returns it.
Syntax:
array.pop();
Example:
let array = ["white", "yellow", "black", "green", "blue"].pop();
console.log("The removed color is : " + array );
Output:
The removed color is : blue
Use delete
Operator to Remove an Array Item in TypeScript
The delete
operator in TypeScript completely deletes the value of the property and the object’s property, but we can still achieve the functionality of removing elements using the operator. The property cannot be used again when deleted unless it is added back again.
Using the delete
operator is not recommended as we can achieve the same result in a much more efficient and safe way by using the methods of splice()
, shift(),
and pop()
.
Example:
let array = ["white", "yellow", "black", "green", "blue"];
delete array[1];
console.log("The array after deletion : " + array);
Output:
The array after deletion : white,,black,green,blue
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.