The Date Object in TypeScript
- Date Object in TypeScript
-
Use the
new Date()
Function in TypeScript -
Use the
new Date(milliseconds)
Function in TypeScript -
Use the
new Date(datestring)
Function in TypeScript -
Use the
new Date (yrs, mth, dy[, h, m, s, millisecond ])
Function in TypeScript - Date Object Properties in TypeScript
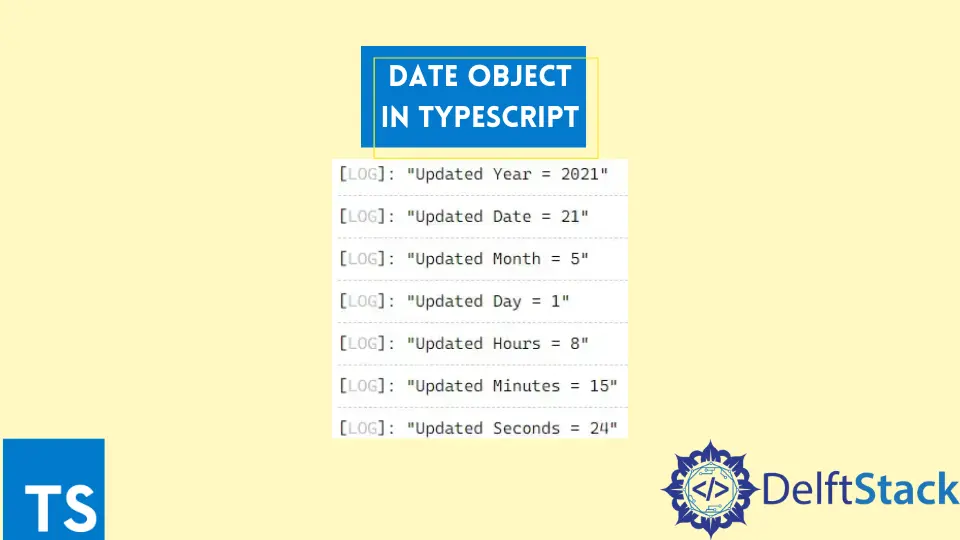
In this article, we’ll discuss what a Date Object in TypeScript is with the help of some examples. We will discuss the methods of Date objects as well.
Date Object in TypeScript
The Date object is used to represent date and time functionality in TypeScript. It helps us get or set the year, month and day, hour, minute, second, and millisecond.
By default, if we create a date without any clash passed to its constructor, that will get the date and time. The Date object in TypeScript helps the functions which deal with Coordinated Universal Time (UTC) time, also known as Greenwich Mean Time (GMT).
Let’s discuss the ways to create a new date object.
Use the new Date()
Function in TypeScript
We can create a new date object with the current date and time using a TypeScript function.
Example Code:
# Typescript
let Newdate: Date = new Date();
console.log ("New Date = " + Newdate);
Output:
Use the new Date(milliseconds)
Function in TypeScript
We can create a new date object with the time plus the value of the milliseconds we pass to the object.
Syntax:
# Typescript
New Date (milliseconds)
Example Code:
# Typescript
let Newdate: Date = new Date(400);
console.log("Date After 400 = " + Newdate);
Output:
Use the new Date(datestring)
Function in TypeScript
We can make a new date object by passing the value of the date string to the object by using a TypeScript function.
Syntax:
# Typescript
New Date (datestring)
Example Code:
# Typescript
let Newdate: Date = new Date("2022-05-13");
console.log("Date = " + Newdate);
Output:
Use the new Date (yrs, mth, dy[, h, m, s, millisecond ])
Function in TypeScript
We can create a new date object with the year, month, days, hours, minutes, seconds, and milliseconds by passing the values for respective parameters to the object.
Syntax:
# Typescript
New Date (yrs, mth, dy[, h, m, s, millisecond ])
Example Code:
# Typescript
let Newdate: Date = new Date("2022-05-13");
console.log("Date = " + Newdate);
Output:
Date Object Properties in TypeScript
There are two properties in Date Object which are constructor and prototype. In Date Object, the constructor helps us to specify the function that creates an object’s prototype, whereas the prototype allows us to add properties and methods to an object.
Let’s have an example in which we’ll use the date()
function.
Example Code:
# Typescript
let Newdate: Date = new Date("2022-05-13");
Newdate.setDate(21);
Newdate.setMonth(5);
Newdate.setFullYear(2021);
Newdate.setHours(8);
Newdate.setMinutes(15);
Newdate.setSeconds(24);
console.log("Updated Year = " + Newdate.getFullYear());
console.log("Updated Date = " + Newdate.getDate());
console.log("Updated Month = " + Newdate.getMonth());
console.log("Updated Day = " + Newdate.getDay());
console.log("Updated Hours = " + Newdate.getHours());
console.log("Updated Minutes = " + Newdate.getMinutes());
console.log("Updated Seconds = " + Newdate.getSeconds());
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn