TypeScript の Date オブジェクト
- TypeScript の Date オブジェクト
-
TypeScript で
new Date()
関数を使用する -
TypeScript で
new Date(milliseconds)
関数を使用する -
TypeScript で
new Date(datestring)
関数を使用する -
TypeScript で
new Date (yrs, mth, dy[, h, m, s, millisecond ])
関数を使用する - TypeScript の Date オブジェクトのプロパティ
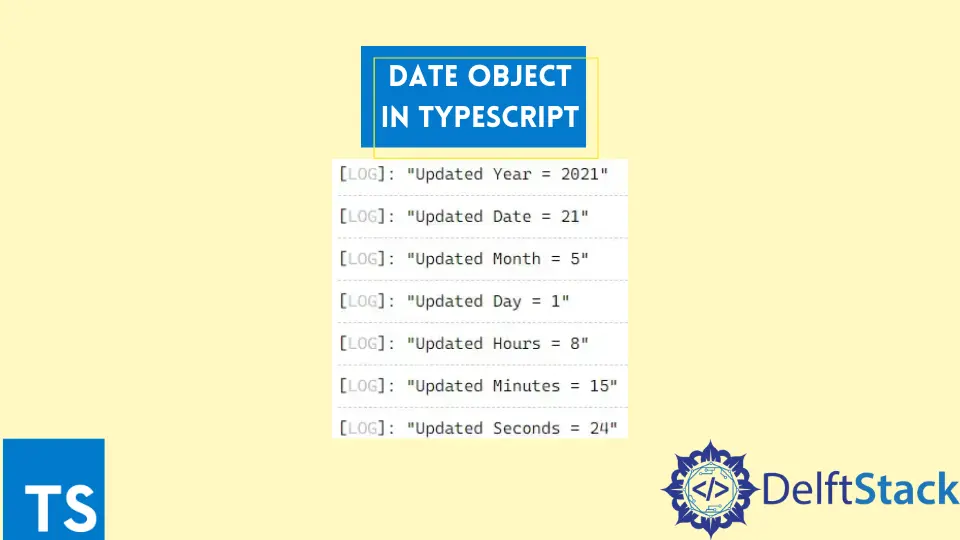
この記事では、いくつかの例を使用して、TypeScript の Date オブジェクトについて説明します。 Date オブジェクトのメソッドについても説明します。
TypeScript の Date オブジェクト
Date オブジェクトは、TypeScript で日付と時刻の機能を表すために使用されます。 年、月、日、時、分、秒、ミリ秒を取得または設定するのに役立ちます。
デフォルトでは、コンストラクターにクラッシュを渡さずに日付を作成すると、日付と時刻が取得されます。 TypeScript の Date オブジェクトは、グリニッジ標準時 (GMT) とも呼ばれる協定世界時 (UTC) 時刻を処理する関数に役立ちます。
新しい日付オブジェクトを作成する方法について説明しましょう。
TypeScript で new Date()
関数を使用する
TypeScript 関数を使用して、現在の日付と時刻で新しい日付オブジェクトを作成できます。
コード例:
# Typescript
let Newdate: Date = new Date();
console.log ("New Date = " + Newdate);
出力:
TypeScript で new Date(milliseconds)
関数を使用する
オブジェクトに渡すミリ秒の値と時間で新しい日付オブジェクトを作成できます。
構文:
# Typescript
New Date (milliseconds)
コード例:
# Typescript
let Newdate: Date = new Date(400);
console.log("Date After 400 = " + Newdate);
出力:
TypeScript で new Date(datestring)
関数を使用する
TypeScript 関数を使用して日付文字列の値をオブジェクトに渡すことで、新しい日付オブジェクトを作成できます。
構文:
# Typescript
New Date (datestring)
コード例:
# Typescript
let Newdate: Date = new Date("2022-05-13");
console.log("Date = " + Newdate);
出力:
TypeScript で new Date (yrs, mth, dy[, h, m, s, millisecond ])
関数を使用する
それぞれのパラメーターの値をオブジェクトに渡すことで、年、月、日、時、分、秒、およびミリ秒で新しい日付オブジェクトを作成できます。
構文:
# Typescript
New Date (yrs, mth, dy[, h, m, s, millisecond ])
コード例:
# Typescript
let Newdate: Date = new Date("2022-05-13");
console.log("Date = " + Newdate);
出力:
TypeScript の Date オブジェクトのプロパティ
Date オブジェクトには、コンストラクターとプロトタイプの 2つのプロパティがあります。 Date Object では、コンストラクターはオブジェクトのプロトタイプを作成する関数を指定するのに役立ちますが、プロトタイプはオブジェクトにプロパティとメソッドを追加することを可能にします。
date()
関数を使用する例を見てみましょう。
コード例:
# Typescript
let Newdate: Date = new Date("2022-05-13");
Newdate.setDate(21);
Newdate.setMonth(5);
Newdate.setFullYear(2021);
Newdate.setHours(8);
Newdate.setMinutes(15);
Newdate.setSeconds(24);
console.log("Updated Year = " + Newdate.getFullYear());
console.log("Updated Date = " + Newdate.getDate());
console.log("Updated Month = " + Newdate.getMonth());
console.log("Updated Day = " + Newdate.getDay());
console.log("Updated Hours = " + Newdate.getHours());
console.log("Updated Minutes = " + Newdate.getMinutes());
console.log("Updated Seconds = " + Newdate.getSeconds());
出力:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn