How to Format Date and Time in TypeScript
- Date Object With No Parameters in TypeScript
- Date Object With One Parameter in TypeScript
- Date Object With a String Parameter in TypeScript
- Date Object With Multiple Parameters in TypeScript
-
Use the
get
Methods to Get the Date and Time in TypeScript -
Use the
set
Methods to Set the Date and Time in TypeScript - Methods to Format Date and Time in TypeScript
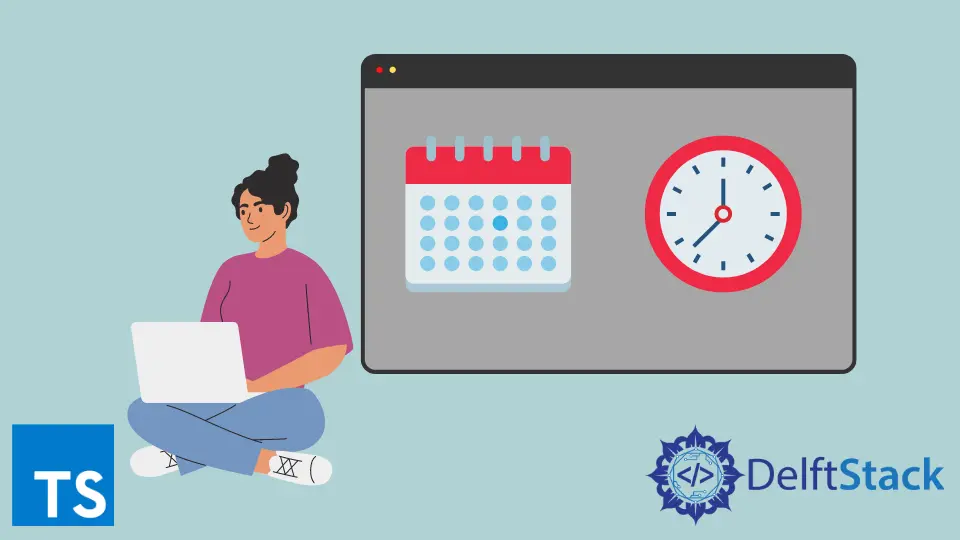
This tutorial will introduce the built-in object Date()
and discuss the various methods to get, set, and format the date and time in Typescript.
Date Object With No Parameters in TypeScript
Whenever the constructor Date()
is called without any parameters, it returns the date and time of the current device the compiler is running.
Example:
let myDate: Date = new Date();
console.log('My date and time is = ' + myDate);
Output:
My date and time is = Fri Feb 18 2022 19:45:32 GMT+0000 (Country Standard Time)
Date Object With One Parameter in TypeScript
Example:
let myDate: Date = new Date(600000000000);
console.log('My date and time is = ' + myDate);
Output:
My date and time is = Thu Jan 05 1989 15:40:00 GMT+0000 (Country Standard Time)
In the above example, the format is the same as the previous one, but the time and date are different. The Date()
constructor now has a parameter milliseconds
added to the compiler starting time and date.
In general, the compiler starting date and time is Thu Jan 01 1970 00:00:00
. Then 600000000000 milliseconds is equal to 166666.667 hours.
So, these hours added to the compiler starting date and time gives the output Thu Jan 05 1989 15:40:00
.
Date Object With a String Parameter in TypeScript
Example 1:
let myDate: Date = new Date("2018-02-08T10:30:35");
console.log('My date and time is = ' + myDate);
Output:
My date and time is = Thu Feb 08 2018 10:30:35 GMT+0000 (Country Standard Time)
In the output, the date and time set by the user are displayed. Notice the parameter passed in the Date()
constructor is a string.
The syntax for the string parameter:
(year-month-date T hours: minutes: seconds)
Here, the T
separates the date from the time.
The compiler will take the default date and month if the user only mentions the year.
Example 2:
let myDate: Date = new Date("2018T10:30:35");
console.log('My date and time is = ' + myDate);
Output:
My date and time is = Thu Jan 01 2018 10:30:35 GMT+0000 (Country Standard Time)
Date Object With Multiple Parameters in TypeScript
Example 1:
let myDate: Date = new Date(2018, 9, 2, 12, 15, 45, 2000);
console.log('My date and time is = ' + myDate);
Output:
My date and time is = Tue Oct 02 2018 12:15:47 GMT+0000 (Country Standard Time)
The format for date and time is still the same as the above example, but now, the Date()
constructors have multiple parameters.
The syntax for multiple parameters:
(year, month, date, hours, minutes, seconds, milliseconds)
In the above example, notice that seconds
is given a value 45
, and milliseconds
is given 2000
. That’s why the time calculated is 12 : 15 : 47
.
If the user only enters four parameters, these values will be assigned sequentially, and the rest will be given the default values.
Example 2:
let myDate: Date = new Date(2018, 9, 2, 12);
console.log('My date and time is = ' + myDate);
Output:
My date and time is = Tue Oct 02 2018 12:00:00 GMT+0000 (Country Standard Time)
Use the get
Methods to Get the Date and Time in TypeScript
As seen above, the Date()
object gives us all the details about the date and time. If the user wants to get only one value from that entire string, the user has to use the built-in get
methods to get the respective value.
Example:
let myDate: Date = new Date(2018, 9, 2, 12, 15, 45);
console.log('My date and time is = ' + myDate);
console.log('The year is = ' + myDate.getFullYear());
console.log('The month is = ' + myDate.getMonth());
console.log('The date is = ' + myDate.getDate());
console.log('The hours are = ' + myDate.getHours());
console.log('The minutes are = ' + myDate.getMinutes());
console.log('The seconds are = ' + myDate.getSeconds());
Output:
My date and time is = Tue Oct 02 2018 12:15:45 GMT+0000 (Country Standard Time)
The year is = 2018
The month is = 9
The date is = 2
The hours are = 12
The minutes are = 15
The seconds are = 45
In the code above, many built-in get
methods are used. As the name describes their functionality, each method returns the value accordingly.
Again, the default value will be printed in the output if any value is missing. There are many other built-in methods for getting the date and time.
Use the set
Methods to Set the Date and Time in TypeScript
If the user wants to set a new value for a specific entity, instead of instantiating the Date
object again, the set
methods can help.
Example:
let myDate: Date = new Date(2018, 9, 2, 12, 15, 45);
console.log('My date and time is = ' + myDate);
myDate.setFullYear(2020);
myDate.setMonth(7);
myDate.setDate(19);
myDate.setHours(16);
myDate.setMinutes(33);
myDate.setSeconds(12);
console.log('My updated date and time is = ' + myDate);
Output:
My date and time is = Tue Oct 02 2018 12:15:45 GMT+0000 (Country Standard Time)
My updated date and time is = Wed Aug 19 2020 16:33:12 GMT+0000 (Country Standard Time)
Notice the difference between the previous and updated date and time in the above example. There are many other built-in methods for setting the date and time that we can use.
Methods to Format Date and Time in TypeScript
We have many built-in methods for formatting date and time, and each performs a specific action. Below are the most commonly used methods.
Example:
let myDate: Date = new Date(2018, 9, 2, 12, 15, 45);
console.log('My date and time is = ' + myDate);
console.log('The ISO 8601 formatted date and time is = ' + myDate.toISOString());
console.log('The Britian formatted date and time is = ' + myDate.toLocaleString("en-GB"));
console.log('The American formatted date and time is = ' + myDate.toLocaleString("en-US"));
console.log(JSON.stringify({
myJSONDate: myDate
}));
Output:
My date and time is = Tue Oct 02 2018 12:15:45 GMT+0000 (Country Standard Time)
The ISO 8601 formatted date and time is = 2018-10-02T07:15:45.000Z
The Britian formatted date and time is = 02/10/2018, 12:15:45
The American formatted date and time is = 10/2/2018, 12:15:45 PM
{"myJSONDate":"2018-10-02T07:15:45.000Z"}
Notice how the different methods format the date and time in the above example. It is up to the user in which format he wants to display the date and time.
The toJSON()
method is used during API references.
The toLocaleString()
is used to format the date and time as per the region.
The toISOString()
is used to format the date and time based on the ISO 8601 formation.
There are many other methods for formatting the date and time.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn