Mutable List in Scala
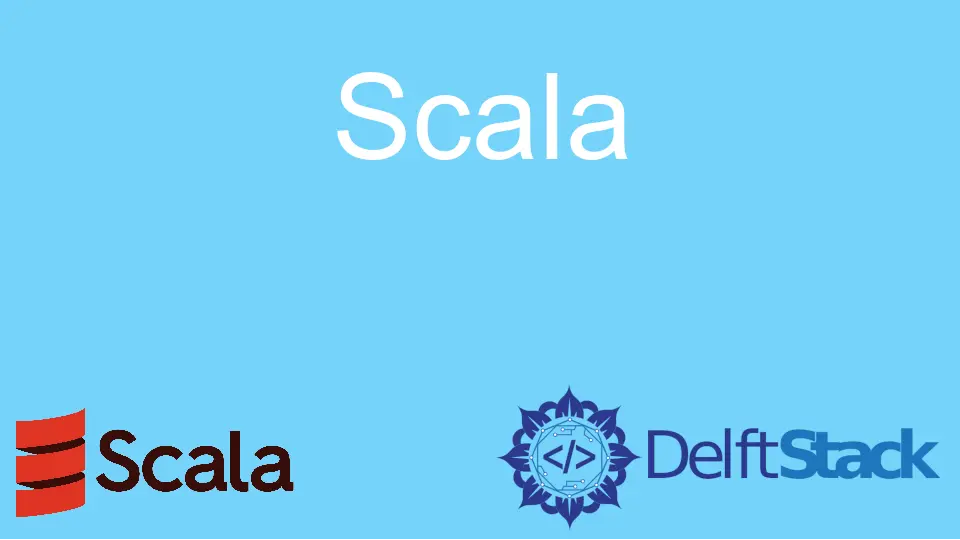
In this article, we will learn about mutable lists in Scala. List by default in Scala are immutable means once initialized, they can’t be updated, but Scala does provide many mutable lists.
Let’s look at them one by one.
DoubleLinkedList
in Scala
DoubleLinkedList
in Scala is a linked list with two references, prev
and next
, to go to the previous node or next node; having two references allows us to traverse the list back and forth.
Let’s look at an example.
object MyClass {
class Node(var data: Int,var prev: Node, var next: Node)
{
def this(data: Int)
{
this(data, null, null)
}
}
class DoublyLinkedList(var head:Node,var tail: Node)
{
def this()
{
this(null, null)
}
def insert(x: Int): Unit = {
var node:Node = new Node(x);
if (this.head == null)
{
this.head = node;
this.tail = node;
return;
}
this.tail.next = node;
node.prev = this.tail;
this.tail = node;
}
def show(): Unit = {
if (this.head == null)
{
println("List is empty");
}
else
{
print("\nPrinting list from front to back :\n");
var first: Node = this.head;
while (first!= null)
{
print(" " + first.data);
first = first.next;
}
print("\nPrinting list from back to front:\n");
var last: Node = this.tail;
while (last!= null)
{
print(" " + last.data);
last = last.prev;
}
}
}
}
def main(args: Array[String])
{
var dll: DoublyLinkedList = new DoublyLinkedList();
dll.insert(100);
dll.insert(200);
dll.insert(300);
dll.insert(400);
dll.show(); //to print the list
}
}
Output:
Printing list from front to back :
100 200 300 400
Printing list from back to front:
400 300 200 100
LinkedList
in Scala
LinkedList
in Scala is used to create a single linked list, so we have no prev
pointer here. It is used when we want to traverse to the next node that is only in one direction.
So here, we can create links and modify them as required.
Example code:
case class LinkedList[obj]()
{
var head: node[obj] = null;
def insert(myData: obj) = {
head match {
case null => head = new node(myData,null)
case _ =>
{
var end:node[obj] = head;
while (end.next!= null) {
end = end.next;
}
end.next = new node[obj](myData, null);
}
}
}
def display()
{
if (head != null) {
head.displayLinkedList();
}
println();
}
}
sealed case class node[obj](var data: obj, var next: node[obj])
{
def myData: obj = this.data
def nextPtr: node[obj] = this.next;
def displayLinkedList(): Unit = {
print(data)
if (next!= null) {
print(" -> ")
next.displayLinkedList();
}
}
}
object Main extends App {
var list: LinkedList[Int] = new LinkedList();
list.insert(100);
list.insert(200);
list.insert(300);
list.display();
}
Output:
100 -> 200 -> 300
DoubleLinkedList
and LinkedList
are used to create more complex list structures such as mutable.Queues
and mutable.lists
.
ListBuffer
in Scala
ListBuffer
, as the name suggests, is a Buffer implementation backed by a list. The main advantage of using it is that it performs most linear-time operations.
Operations such as append and prepend. Hence, it is widely used to implement a list when it wants it to be mutable.
Since the list is immutable, we use ListBuffer
when we want to have a list that keeps changing frequently. To use ListBuffer
, we have to import scala.collection.mutable.ListBuffer
in our program.
Syntax:
var one = new ListBuffer[data_type]() //this creates empty buffer
OR
var two = new ListBuffer("apple","orange","watermelon")
Some of the common operations which are performed on a ListBuffer
are:
- We can use
LB+=element
to append an element in constant time. - We can use
LB+=:Buffer
to prepend an element in constant time. - Once we are done with our list, we can use
LB.toList
to convert theListBuffer
(mutable) toList
(immutable) in constant time.
Example code:
import scala.collection.mutable.ListBuffer
object MyClass {
def main(args: Array[String]) {
var lb = ListBuffer[String]()
lb+= "Apple";
lb+= "Orange";
"dragonFruit"+=:lb //prepending
println(lb);
var result: List[String] = lb.toList
println(result)
}
}
Output:
ListBuffer(dragonFruit, Apple, Orange)
List(dragonFruit, Apple, Orange)