How to Create a List in Scala
- Creating List Using List Class in Scala
-
Creating List and Filling Using
List.tabulate()
Method in Scala -
Creating List and Filling Using
List.fill()
Method in Scala
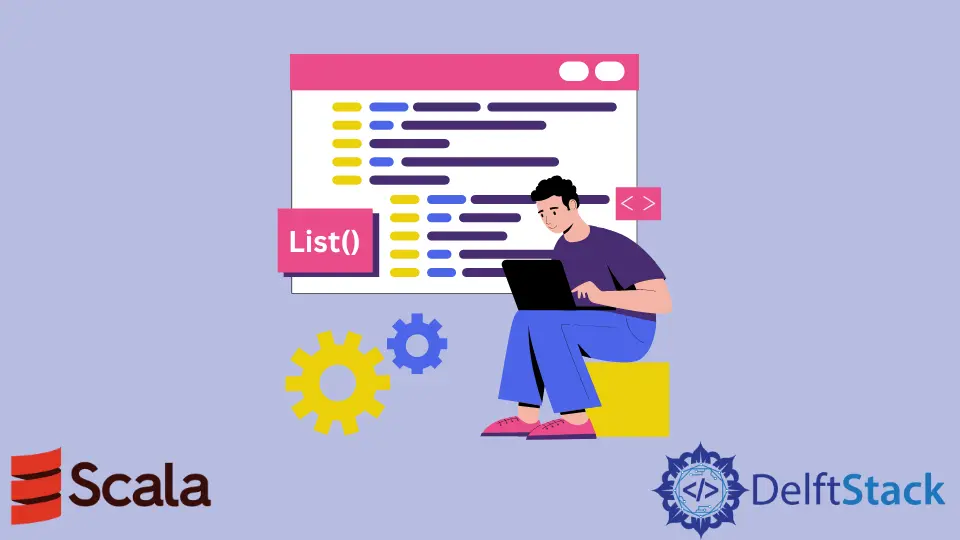
In Scala, implementing list provides List class and is good for last-in-first-out (LIFO), stack-like access pattern. It maintains the order of elements and can contain duplicated elements as well.
We’ll go through the List data structure and how to create and use it in Scala in this lesson.
Creating List Using List Class in Scala
In this example, we created the List class object that accepts integer type values, and then we assigned some values to it and displayed the list.
Code:
object MyClass {
def main(args: Array[String]) {
// creating empty list
var list = List[Integer]()
println(list);
// filling elements
list = List(1,9,5,6,8,58,23,15,4)
println(list)
}
}
Output:
List()
List(1, 9, 5, 6, 8, 58, 23, 15, 4)
We can do the above task using a single statement. Here, we created and assigned elements simultaneously to make the code more concise.
Example:
object MyClass {
def main(args: Array[String]) {
// creating a list
// filling elements
var list:List[Int] = List(1,9,5,6,8,58,23,15,4)
println(list)
}
}
Output:
List(1, 9, 5, 6, 8, 58, 23, 15, 4)
Creating List and Filling Using List.tabulate()
Method in Scala
We can use the tabulate()
method to fill or assign elements to the list. First, we create the list using the List()
constructor and then assign elements.
The tabulate()
method belongs to the List class and takes a function as an argument to make a list.
Example:
object MyClass {
def main(args: Array[String]) {
// creating a list
var list = List[Integer]()
println(list);
// filling elements
list = List.tabulate(5)(x => x * 2)
println(list)
}
}
Output:
List()
List(0, 2, 4, 6, 8)
Creating List and Filling Using List.fill()
Method in Scala
Using the fill()
method, we can fill or assign elements to the list. The fill()
method belongs to the List class and fills a list.
Example
object MyClass {
def main(args: Array[String]) {
// creating empty list
var list = List[Integer]()
println(list);
// filling elements
list = List.fill(5)(scala.util.Random.nextInt)
println(list)
}
}
Output:
List()
List(549949711, -1323692034, -1659330183, -1962543465, -1259033893)