Difference Between Sequence and List in Scala
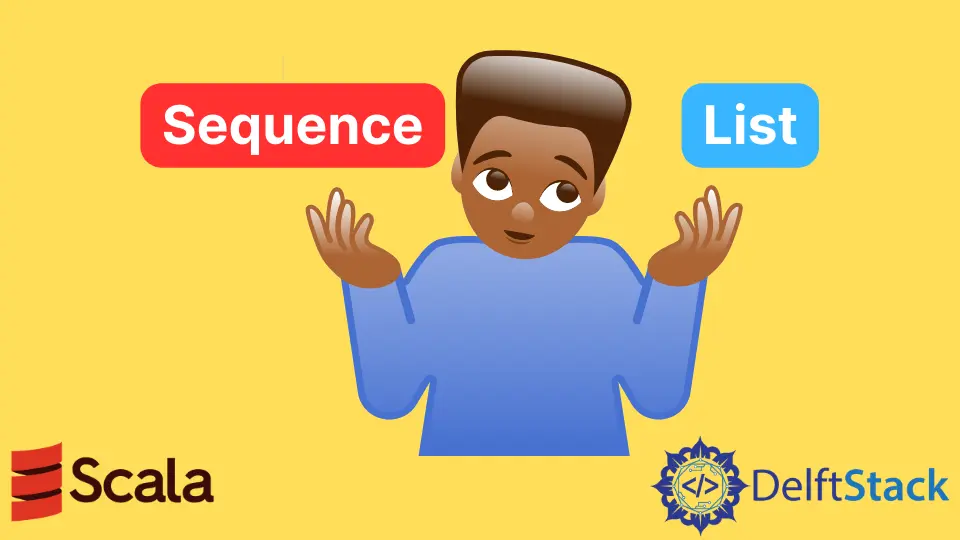
Scala is a compiler-based programming language invented in the year 2003. Scala supports functional programming and object-oriented programming.
A container data structure in Scala that contains one or more elements is known as a collection
. The article will explain the difference between Scala’s Collections, the Sequence
and List
.
Using Sequence
and List
in Scala
Sequence
is a trait that List
implements, a type of collection where the elements are stored in an indexed manner. Sequence
is guaranteed immutable.
List
is a collection that works like a linked list data structure. Lists
are immutable, but if we want a list that keeps changing constantly, ListBuffer
can be used, which is mutable.
Scala’s sequence
is equivalent to Java’s List
, while Scala’s list
is equivalent to Java’s LinkedList
.
Syntax:
Sequence : var mySeq = Seq(elements)
list : var myList = List(elements)
Both collections can store information, yet the sequence
has a few extra highlights over the list
. In Scala, a list
is a particular collection that is streamlined and regularly utilized in functional programming.
List Sample Code:
object MyClass {
def main(args: Array[String]) {
val myList : List[Int] = List(11,22,33,44,55,66,77,88,99)
println("The list is "+ myList)
}
}
Output:
The list is List(11, 22, 33, 44, 55, 66, 77, 88, 99)
Sequence Sample Code:
object MyClass {
def main(args: Array[String]) {
val mySequence : Seq[Int] = Seq(101,2020,303,404,505,606,770,808,909)
println("The Sequence is "+ mySequence)
}
}
Output:
The Sequence is List(101, 2020, 303, 404, 505, 606, 770, 808, 909)
Based on the above output, we can observe that in Scala, by default, sequence
is initialized as a list
.