How to Append or Prepend Elements to Sequence in Scala
-
Use the
:+
Operator to Append an Element to Sequence in Scala -
Use
++
to Append Multiple Elements to Sequence in Scala -
Use
+:
to Prepend an Element to Sequence in Scala -
Use
++:
to Prepend Multiple Elements to Sequence in Scala - Conclusion
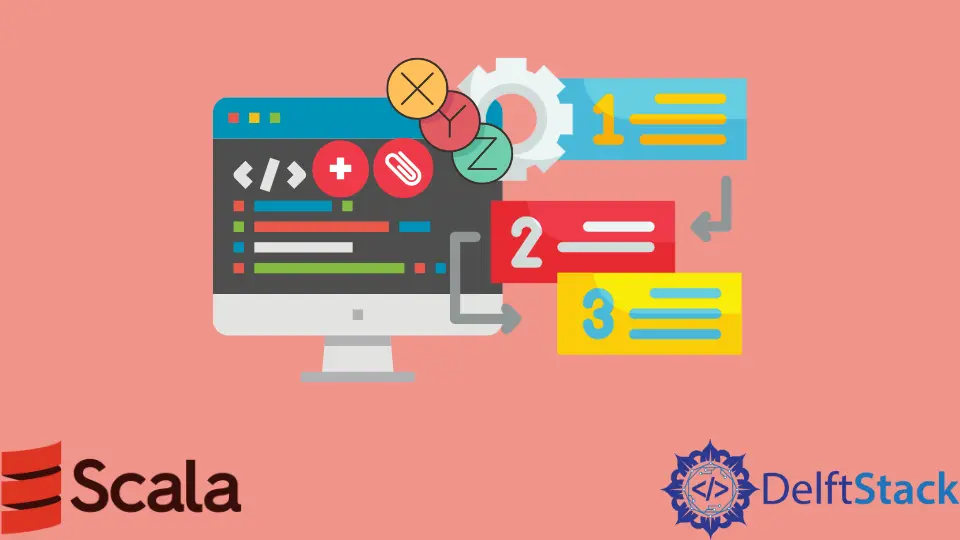
Scala, a powerful and versatile programming language, offers a rich set of tools for manipulating collections. One common operation is to append and prepend elements to a sequence.
In Scala, a Seq
is a trait (similar to an interface in Java) that represents an ordered collection of elements. It extends the Iterable
trait and defines a specific order for elements.
It is immutable by nature, which means we cannot modify it. Since they are immutable, the result of appending or prepending elements to a sequence should be assigned to a new variable.
There are multiple ways to append or prepend elements to a sequence; let’s see them one by one.
Use the :+
Operator to Append an Element to Sequence in Scala
The :+
operator is specifically designed for appending elements to sequences in Scala. It is applicable to a wide range of sequence types, including lists, arrays, vectors, and more.
This operator provides a concise and efficient way to extend collections dynamically.
Syntax:
val modifiedSequence = originalSequence :+ elementToAdd
originalSequence
: The sequence to which we want to append an element.elementToAdd
: The new element that will be added to the end of the sequence.
Example code:
object MyClass {
def main(args: Array[String])
{
val a = Seq("Apple", "Orange", "Mango")
val temp = a :+ "Watermelon"
println(temp)
}
}
This code defines an object named MyClass
with a main
method, which serves as the entry point of the program. Inside the main
method, a val
named a
is declared and initialized as a sequence (represented by Seq
) containing three strings: "Apple"
, "Orange"
, and "Mango"
.
Next, a new variable named temp
is created. This variable is assigned the result of appending (using the :+
operator) the string "Watermelon"
to the sequence a
.
Essentially, this operation creates a new sequence that includes all the elements of a
plus the additional "Watermelon"
element. Finally, the program prints out the contents oftemp
to the console.
When executed, this code will output a sequence containing "Apple"
, "Orange"
, "Mango"
, and "Watermelon"
, demonstrating the successful appending of a new element to the original sequence.
List(Apple, Orange, Mango, Watermelon)
We can see the string Watermelon
is appended.
Use ++
to Append Multiple Elements to Sequence in Scala
The ++
operator is a versatile tool in Scala used for concatenating sequences. It can be applied to a variety of sequence types, including lists, arrays, vectors, and more.
This operator allows you to combine multiple sequences, making it a valuable asset for managing collections in your Scala projects.
Syntax:
val combinedSequence = sequence1 ++ sequence2
sequence1
andsequence2
: The sequences that you want to concatenate.combinedSequence
: The resulting sequence after concatenation.
In Scala, we can use the ++
operator to append multiple elements to the sequence or a vector. The collection of elements could mean another vector, sequence, or list.
Example code:
object MyClass {
def main(args: Array[String])
{
val fruits = Seq("Apple", "Orange", "Mango")
val vegetables = Seq("Onion","tomato","potato")
val temp = fruits ++ vegetables
println(temp)
}
}
In this example, the main
method has two sequences: fruits
and vegetables
.
fruits
is initialized as a sequence containing the strings "Apple"
, "Orange"
, and "Mango"
. Similarly, vegetables
is initialized as a sequence with the strings "Onion"
, "tomato"
, and "potato"
.
Next, the ++
operator is used to concatenate these two sequences. The ++
operator is designed for concatenating sequences in Scala.
In this case, it combines the elements from fruits
and vegetables
, resulting in a new sequence called temp
. This new sequence now contains all the elements from both fruits
and vegetables
.
Finally, the program prints out the content of the temp
sequence. When executed, this code will output a combined list of fruits
and vegetables
:
List(Apple, Orange, Mango, Onion, tomato, potato)
This demonstrates how the ++
operator can be utilized to efficiently concatenate sequences in Scala, providing a concise and effective way to combine collections.
Use +:
to Prepend an Element to Sequence in Scala
The +:
operator is specifically designed for prepending elements to sequences in Scala. It is applicable to various sequence types, including lists, arrays, vectors, and more.
This operator offers a concise and efficient way to extend collections dynamically.
Syntax:
val modifiedSequence = elementToAdd +: originalSequence
elementToAdd
: The new element that will be added to the beginning of the sequence.originalSequence
: The sequence to which we want to prepend an element.
We can use the +:
operator to prepend an element to a sequence or vector in Scala.
This code demonstrates how the +:
operator efficiently adds an element to the beginning of a sequence, enabling dynamic modification of collections in Scala.
object MyClass {
def main(args: Array[String])
{
val fruits = Seq("Apple", "Orange", "Mango")
val temp = "dragonFruit" +: fruits
println(temp)
}
}
First, a val
named fruits
is declared and assigned a sequence of strings: "Apple"
, "Orange"
, and "Mango"
. This sequence represents a collection of fruits.
Next, a new sequence is created using the +:
operator. This operator is used to prepend an element to a sequence.
In this case, the element being added is the string "dragonFruit"
. As a result, the new sequence temp
will contain "dragonFruit"
followed by the original elements from fruits
.
Finally, the println
statement is used to output the contents of the temp
sequence. When executed, this program will print:
List(dragonFruit, Apple, Orange, Mango)
We can see the string dragonFruit
is prepended.
Use ++:
to Prepend Multiple Elements to Sequence in Scala
The ++:
operator is a concise way to concatenate two sequences, with the elements of the second sequence being added at the beginning of the first sequence. This operator is especially useful when you need to add multiple elements to the beginning of a sequence in a single operation.
Syntax:
val modifiedSequence = elementsToPrepend ++: originalSequence
elementsToPrepend
: The sequence containing the elements you want to add at the beginning.originalSequence
: The sequence to which you want to prepend elements.
In Scala, we can use the ++:
operator to prepend multiple elements to the sequence or a vector. The collection of elements could mean another vector, sequence, or list.
This code demonstrates how sequences like Seq
and Vector
can be combined in Scala, showcasing the flexibility and power of the language’s collection operations.
object MyClass {
def main(args: Array[String])
{
val fruits = Seq("Apple", "Orange", "Mango")
val data = Vector(1,2,3,4,5)
val temp = data ++: fruits
println(temp)
}
}
First, a Seq
named fruits
is created, containing the strings "Apple"
, "Orange"
, and "Mango"
. A Seq
in Scala is a collection type that maintains a specific order of elements. In this case, it holds strings representing different types of fruits.
Next, a Vector
named data
is initialized with the numbers 1
through 5
. Vectors in Scala are immutable, indexed sequences that offer efficient access to elements.
The line val temp = data ++: fruits
combines the contents of data
and fruits
using the ++:
operator. This operator appends the elements of fruits
to the beginning of data
.
The result is a new Vector
named temp
, which now contains the numbers 1
through 5
followed by the fruits.
Finally, println(temp)
is used to output the contents of temp
to the console. When executed, this code will produce the following output:
List(1, 2, 3, 4, 5, Apple, Orange, Mango)
Conclusion
In conclusion, Scala provides a powerful set of tools for manipulating collections. A Seq
in Scala represents an ordered collection of elements and is immutable by nature. This means that when appending or prepending elements, the result should be assigned to a new variable.
There are several ways to append or prepend elements to a sequence:
- The
:+
operator is specifically designed for appending elements to sequences, providing a concise and efficient way to extend collections dynamically. - The
++
operator is a versatile tool for concatenating sequences, allowing you to combine multiple sequences efficiently. - The
+:
operator is used to prepend elements to sequences, offering a concise and efficient way to extend collections dynamically. - The
++:
operator concatenates two sequences, with the elements of the second sequence being added at the beginning of the first sequence. This is especially useful when adding multiple elements to the beginning of a sequence in a single operation.
These operations showcase the flexibility and power of Scala’s collection operations, allowing for dynamic modification and manipulation of sequences.