Lista mutable en Scala
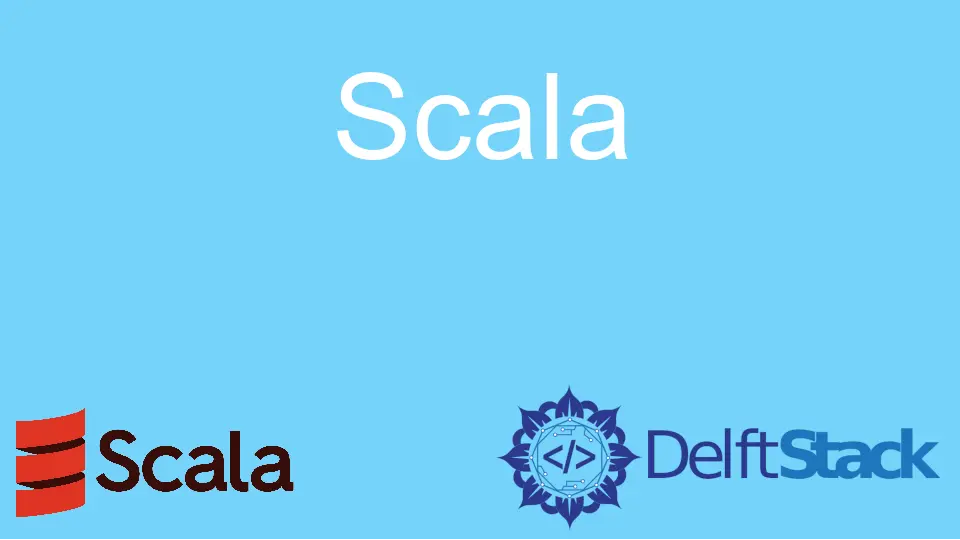
En este artículo, aprenderemos sobre las listas mutables en Scala. Las listas predeterminadas en Scala son medios inmutables una vez inicializados, no se pueden actualizar, pero Scala proporciona muchas listas mutables.
Veámoslos uno por uno.
DoubleLinkedList
en Scala
DoubleLinkedList
en Scala es una lista enlazada con dos referencias, prev
y next
, para ir al nodo anterior o siguiente; tener dos referencias nos permite recorrer la lista de un lado a otro.
Veamos un ejemplo.
object MyClass {
class Node(var data: Int,var prev: Node, var next: Node)
{
def this(data: Int)
{
this(data, null, null)
}
}
class DoublyLinkedList(var head:Node,var tail: Node)
{
def this()
{
this(null, null)
}
def insert(x: Int): Unit = {
var node:Node = new Node(x);
if (this.head == null)
{
this.head = node;
this.tail = node;
return;
}
this.tail.next = node;
node.prev = this.tail;
this.tail = node;
}
def show(): Unit = {
if (this.head == null)
{
println("List is empty");
}
else
{
print("\nPrinting list from front to back :\n");
var first: Node = this.head;
while (first!= null)
{
print(" " + first.data);
first = first.next;
}
print("\nPrinting list from back to front:\n");
var last: Node = this.tail;
while (last!= null)
{
print(" " + last.data);
last = last.prev;
}
}
}
}
def main(args: Array[String])
{
var dll: DoublyLinkedList = new DoublyLinkedList();
dll.insert(100);
dll.insert(200);
dll.insert(300);
dll.insert(400);
dll.show(); //to print the list
}
}
Producción :
Printing list from front to back :
100 200 300 400
Printing list from back to front:
400 300 200 100
Lista enlazada
en Scala
LinkedList
en Scala se usa para crear una sola lista enlazada, por lo que no tenemos un puntero prev
aquí. Se usa cuando queremos atravesar al siguiente nodo que está solo en una dirección.
Así que aquí, podemos crear enlaces y modificarlos según sea necesario.
Código de ejemplo:
case class LinkedList[obj]()
{
var head: node[obj] = null;
def insert(myData: obj) = {
head match {
case null => head = new node(myData,null)
case _ =>
{
var end:node[obj] = head;
while (end.next!= null) {
end = end.next;
}
end.next = new node[obj](myData, null);
}
}
}
def display()
{
if (head != null) {
head.displayLinkedList();
}
println();
}
}
sealed case class node[obj](var data: obj, var next: node[obj])
{
def myData: obj = this.data
def nextPtr: node[obj] = this.next;
def displayLinkedList(): Unit = {
print(data)
if (next!= null) {
print(" -> ")
next.displayLinkedList();
}
}
}
object Main extends App {
var list: LinkedList[Int] = new LinkedList();
list.insert(100);
list.insert(200);
list.insert(300);
list.display();
}
Producción :
100 -> 200 -> 300
DoubleLinkedList
y LinkedList
se utilizan para crear estructuras de listas más complejas, como mutable.Queues
y mutable.lists
.
‘ListBuffer’ en Scala
ListBuffer
, como sugiere el nombre, es una implementación de Buffer respaldada por una lista. La principal ventaja de usarlo es que realiza la mayoría de las operaciones de tiempo lineal.
Operaciones como agregar y anteponer. Por lo tanto, se usa mucho para implementar una lista cuando se quiere que sea mutable.
Dado que la lista es inmutable, usamos ListBuffer
cuando queremos tener una lista que cambia con frecuencia. Para usar ListBuffer
, tenemos que importar scala.collection.mutable.ListBuffer
en nuestro programa.
Sintaxis:
var one = new ListBuffer[data_type]() //this creates empty buffer
OR
var two = new ListBuffer("apple","orange","watermelon")
Algunas de las operaciones comunes que se realizan en un ListBuffer
son:
- Podemos usar
LB+=elemento
para agregar un elemento en tiempo constante. - Podemos usar
LB+=:Buffer
para anteponer un elemento en tiempo constante. - Una vez que hayamos terminado con nuestra lista, podemos usar
LB.toList
para convertirListBuffer
(mutable) aList
(inmutable) en tiempo constante.
Código de ejemplo:
import scala.collection.mutable.ListBuffer
object MyClass {
def main(args: Array[String]) {
var lb = ListBuffer[String]()
lb+= "Apple";
lb+= "Orange";
"dragonFruit"+=:lb //prepending
println(lb);
var result: List[String] = lb.toList
println(result)
}
}
Producción :
ListBuffer(dragonFruit, Apple, Orange)
List(dragonFruit, Apple, Orange)