Scala의 가변 목록
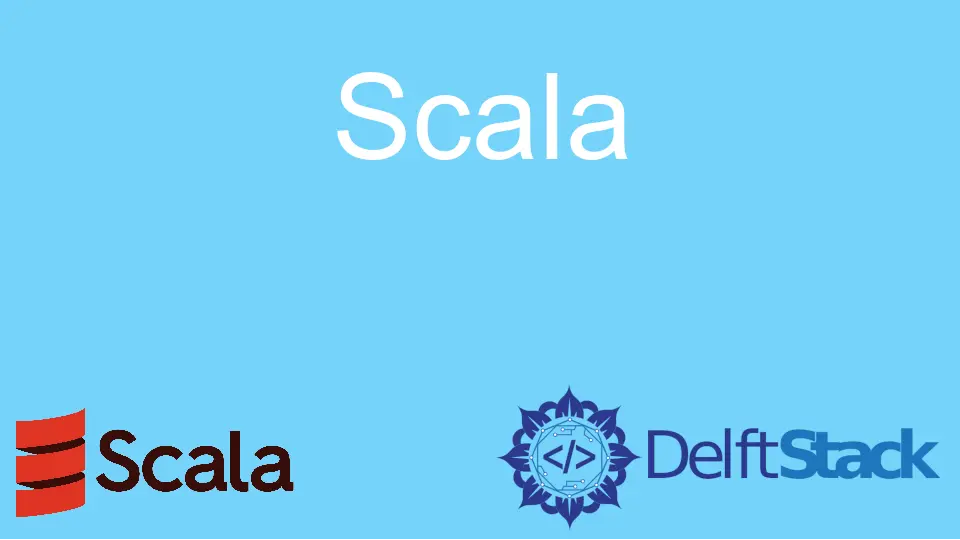
이번 글에서는 Scala의 가변 리스트에 대해 알아보겠습니다. Scala의 기본 목록은 변경할 수 없다는 의미로 초기화되면 업데이트할 수 없지만 Scala는 많은 변경 가능한 목록을 제공합니다.
하나씩 살펴보겠습니다.
스칼라의 ‘DoubleLinkedList’
Scala의 ‘DoubleLinkedList’는 이전 노드 또는 다음 노드로 이동하기 위해 ‘prev’ 및 ’next’라는 두 개의 참조가 있는 연결 목록입니다. 두 개의 참조가 있으면 목록을 앞뒤로 탐색할 수 있습니다.
예를 들어 보겠습니다.
object MyClass {
class Node(var data: Int,var prev: Node, var next: Node)
{
def this(data: Int)
{
this(data, null, null)
}
}
class DoublyLinkedList(var head:Node,var tail: Node)
{
def this()
{
this(null, null)
}
def insert(x: Int): Unit = {
var node:Node = new Node(x);
if (this.head == null)
{
this.head = node;
this.tail = node;
return;
}
this.tail.next = node;
node.prev = this.tail;
this.tail = node;
}
def show(): Unit = {
if (this.head == null)
{
println("List is empty");
}
else
{
print("\nPrinting list from front to back :\n");
var first: Node = this.head;
while (first!= null)
{
print(" " + first.data);
first = first.next;
}
print("\nPrinting list from back to front:\n");
var last: Node = this.tail;
while (last!= null)
{
print(" " + last.data);
last = last.prev;
}
}
}
}
def main(args: Array[String])
{
var dll: DoublyLinkedList = new DoublyLinkedList();
dll.insert(100);
dll.insert(200);
dll.insert(300);
dll.insert(400);
dll.show(); //to print the list
}
}
출력:
Printing list from front to back :
100 200 300 400
Printing list from back to front:
400 300 200 100
스칼라의 ‘LinkedList’
Scala의 ‘LinkedList’는 단일 연결 목록을 만드는 데 사용되므로 여기에는 ‘prev’ 포인터가 없습니다. 한 방향으로만 있는 다음 노드로 이동하고 싶을 때 사용합니다.
따라서 여기에서 링크를 생성하고 필요에 따라 수정할 수 있습니다.
예제 코드:
case class LinkedList[obj]()
{
var head: node[obj] = null;
def insert(myData: obj) = {
head match {
case null => head = new node(myData,null)
case _ =>
{
var end:node[obj] = head;
while (end.next!= null) {
end = end.next;
}
end.next = new node[obj](myData, null);
}
}
}
def display()
{
if (head != null) {
head.displayLinkedList();
}
println();
}
}
sealed case class node[obj](var data: obj, var next: node[obj])
{
def myData: obj = this.data
def nextPtr: node[obj] = this.next;
def displayLinkedList(): Unit = {
print(data)
if (next!= null) {
print(" -> ")
next.displayLinkedList();
}
}
}
object Main extends App {
var list: LinkedList[Int] = new LinkedList();
list.insert(100);
list.insert(200);
list.insert(300);
list.display();
}
출력:
100 -> 200 -> 300
DoubleLinkedList
및 LinkedList
는 mutable.Queues
및 mutable.lists
와 같은 보다 복잡한 목록 구조를 만드는 데 사용됩니다.
스칼라의 ListBuffer
‘ListBuffer’는 이름에서 알 수 있듯이 목록이 지원하는 버퍼 구현입니다. 이를 사용하는 주요 이점은 대부분의 선형 시간 작업을 수행한다는 것입니다.
추가 및 앞에 추가와 같은 작업. 따라서 목록을 변경할 수 있기를 원할 때 목록을 구현하는 데 널리 사용됩니다.
목록은 변경할 수 없기 때문에 자주 변경되는 목록을 원할 때 ListBuffer
를 사용합니다. ListBuffer
를 사용하려면 프로그램에서 scala.collection.mutable.ListBuffer
를 가져와야 합니다.
통사론:
var one = new ListBuffer[data_type]() //this creates empty buffer
OR
var two = new ListBuffer("apple","orange","watermelon")
ListBuffer
에서 수행되는 일반적인 작업 중 일부는 다음과 같습니다.
LB+=요소
를 사용하여 일정한 시간에 요소를 추가할 수 있습니다.LB+=:Buffer
를 사용하여 상수 시간에 요소를 추가할 수 있습니다.- 목록이 완료되면
LB.toList
를 사용하여ListBuffer
(변경 가능)를List
(불변)로 변환할 수 있습니다.
예제 코드:
import scala.collection.mutable.ListBuffer
object MyClass {
def main(args: Array[String]) {
var lb = ListBuffer[String]()
lb+= "Apple";
lb+= "Orange";
"dragonFruit"+=:lb //prepending
println(lb);
var result: List[String] = lb.toList
println(result)
}
}
출력:
ListBuffer(dragonFruit, Apple, Orange)
List(dragonFruit, Apple, Orange)