How to Wrap Text in React Native
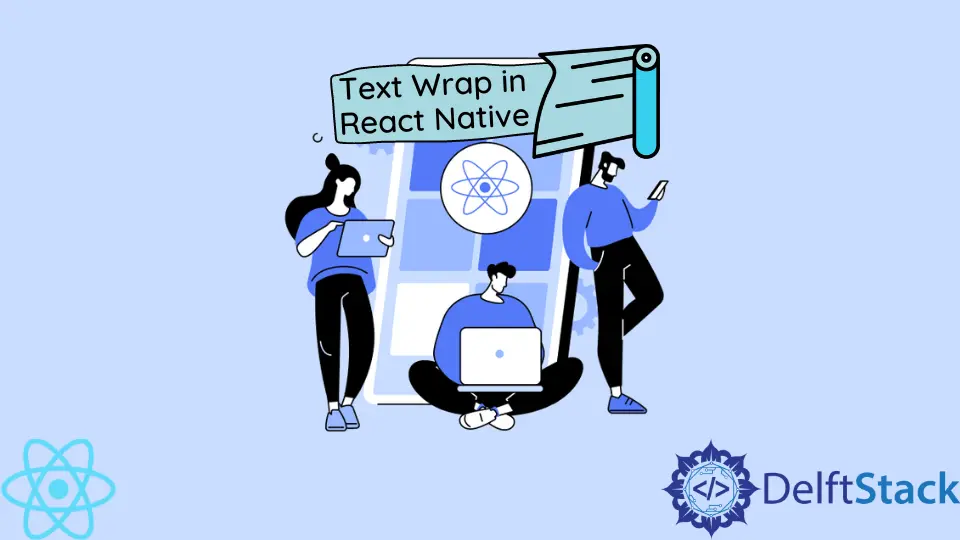
Today’s tutorial demonstrates wrapping text in React Native using different code examples.
Text Wrap in React Native
Since flex-wrap is the only option in React Native containing the word wrap
, you might be inclined to try it.
While some advocate utilizing such a style, its biggest drawback is that it can break flexbox children rather than text, not the text itself.
The practical solutions employed the flex-wrap option, although testing revealed that it was unnecessary. flex: 1
was utilized in a few of the solutions on the parent view.
We didn’t want to do that since it would have affected the design of my software. So instead, we desired my specific parent view to fill the remaining area.
Understanding flex: 1
will allow you to know how the dimension will be distributed among its siblings.
Let’s say you carry out the following:
import { View, Text } from "react-native";
import React from "react";
function App() {
return (
<>
<View>
<View style={{ flex: 1 }}>
<Text>Shiv</Text>
</View>
<View style={{ flex: 2 }}>
<Text>Kumar</Text>
</View>
<View style={{ flex: 1 }}>
<Text>Yadav</Text>
</View>
</View>
</>
);
}
export default App;
Output:
Since the default flexbox orientation in this situation is column (or vertical), the heights of each child will be divided evenly, making 1 + 2 + 1 = 4
.
The middle kid receives 2/4ths
of the height (or 1/2
), while the first and last children each receive 1/4
of the height.
Returning to the React Native text wrap problem. We wanted the text to break; we didn’t want the entire flexbox layout to be affected.
Therefore, you must add flex: 1
to the Text
component and flex-direction: row
to the parent.
This is how it appears:
import React from 'react';
import {SafeAreaView, Text, View} from 'react-native';
function App() {
return (<><SafeAreaView style = {
{
flex: 1, flexDirection: 'row', width: 160
}
}><View style = {
{
width: 50
}
}><Text>Shiv</Text>
</View><View style = {
{
flexGrow: 1, flexDirection: 'row'
}
}><Text style = {
{
flex: 1
}
}>This is how work</Text>
</View><View style = {
{
width: 50
}
}><Text>Yadav</Text>
</View></SafeAreaView>
</>);
}
export default App;
SafeAreaView
renders the content within a device’s boundary.
Output:
If you execute the code above, you’ll observe that it functions flawlessly on native devices but fails to wrap the text for the web properly. Add width: 1
to the Text
component as shown to correct that.
import React from 'react';
import {SafeAreaView, Text, View} from 'react-native';
function App() {
return (<><SafeAreaView style = {
{
flex: 1, flexDirection: 'row', width: 160
}
}><View style = {
{
width: 50
}
}><Text>Shiv</Text>
</View><View style = {
{
flexGrow: 1, flexDirection: 'row'
}
}><Text style = {
{
flex: 1, width: 1
}
}>This is how work</Text>
</View><View style = {
{
width: 50
}
}><Text>Yadav</Text>
</View></SafeAreaView>
</>);
}
export default App;
Output:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn