The for Loop in React Native
- Understanding the for Loop
- Example 1: Iterating Over an Array
- Example 2: Generating a Series of Numbers
- Example 3: Conditional Rendering with for Loop
- Conclusion
- FAQ
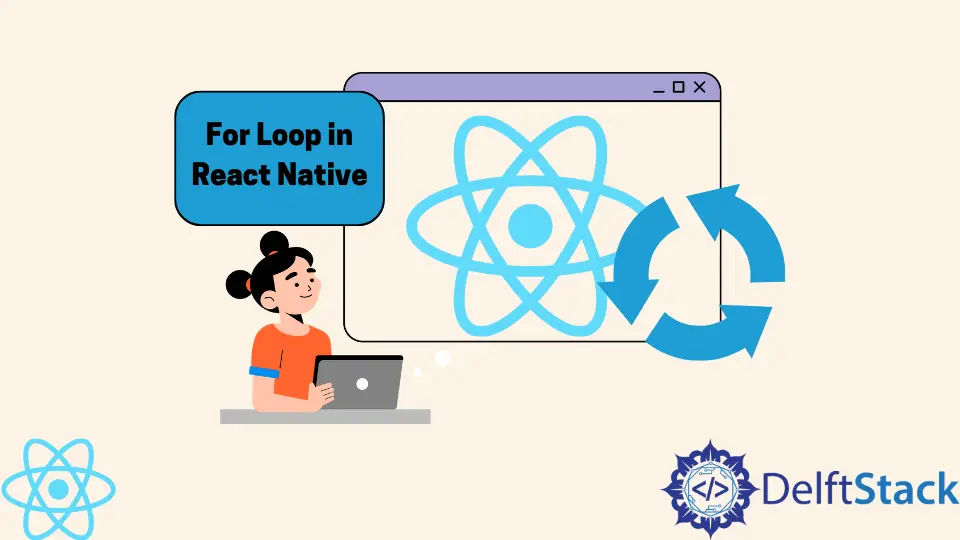
When it comes to building dynamic applications with React Native, understanding loops is essential for managing repetitive tasks efficiently. Among the various looping constructs available in JavaScript, the for
loop stands out for its versatility and straightforward syntax.
This tutorial will discuss how to implement the for
loop in React Native, providing you with practical examples and insights to enhance your coding skills. Whether you’re iterating through arrays or managing component states, mastering the for
loop will significantly improve your development workflow. So, let’s dive in and explore the power of the for
loop in React Native!
Understanding the for Loop
The for
loop in JavaScript is a control structure that allows you to execute a block of code a specific number of times. It consists of three main components: initialization, condition, and increment. The basic syntax looks like this:
for (initialization; condition; increment) {
// code block to be executed
}
In the context of React Native, you can leverage the for
loop to manipulate arrays, render lists of components, or perform repetitive tasks efficiently. Let’s explore some practical examples to see how the for
loop can be effectively used in React Native applications.
Example 1: Iterating Over an Array
One of the most common uses of the for
loop in React Native is to iterate over an array. Suppose you have an array of user names and you want to display them in a list. Here’s how you can achieve this using the for
loop:
const users = ['Alice', 'Bob', 'Charlie', 'David'];
const UserList = () => {
const userElements = [];
for (let i = 0; i < users.length; i++) {
userElements.push(<Text key={i}>{users[i]}</Text>);
}
return <View>{userElements}</View>;
};
Output:
Alice
Bob
Charlie
David
In this example, we declare an array of user names. The for
loop iterates through the array, and for each user, we create a <Text>
component displaying the user’s name. Each component is pushed into the userElements
array, which is then rendered within a <View>
. This approach is particularly useful when you need to display a dynamic list of items based on data.
Example 2: Generating a Series of Numbers
Another practical application of the for
loop in React Native is generating a series of numbers, which can be useful for creating pagination or displaying a range of values. Let’s say you want to display numbers from 1 to 10:
const NumberList = () => {
const numberElements = [];
for (let i = 1; i <= 10; i++) {
numberElements.push(<Text key={i}>{i}</Text>);
}
return <View>{numberElements}</View>;
};
Output:
1
2
3
4
5
6
7
8
9
10
In this example, the for
loop runs from 1 to 10, generating a series of <Text>
components displaying each number. This method is efficient for creating lists of sequential items, and it can easily be adapted to different ranges or conditions.
Example 3: Conditional Rendering with for Loop
The for
loop can also be combined with conditional statements to render components based on specific criteria. For instance, if you want to display only even numbers from a given array, you can do so as follows:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const EvenNumberList = () => {
const evenNumberElements = [];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
evenNumberElements.push(<Text key={i}>{numbers[i]}</Text>);
}
}
return <View>{evenNumberElements}</View>;
};
Output:
2
4
6
8
10
In this example, we check if each number in the numbers
array is even using the modulus operator. When the condition is met, we push the number into the evenNumberElements
array. This technique allows you to filter and display data dynamically based on your application’s requirements.
Conclusion
The for
loop is a powerful tool in React Native that can help you manage repetitive tasks effectively. Whether you are iterating over arrays, generating sequences, or conditionally rendering components, mastering the for
loop will enhance your coding capabilities. By incorporating these examples into your projects, you can create more dynamic and responsive applications. As you continue to explore React Native, remember that understanding fundamental programming concepts like the for
loop will serve as a solid foundation for your development journey.
FAQ
-
What is a for loop in React Native?
A for loop is a control structure that allows you to execute a block of code multiple times, often used for iterating over arrays or generating components. -
How do you use a for loop with arrays in React Native?
You can use a for loop to iterate through an array and render components based on its elements, pushing each component into an array and returning it within a parent component. -
Can you combine for loops with conditional statements?
Yes, you can use conditional statements within a for loop to filter data and render components based on specific criteria.
-
What are some alternatives to the for loop in React Native?
Alternatives include themap
method,forEach
, and other array iteration methods, which can often lead to more concise and readable code. -
Is the for loop the only way to render lists in React Native?
No, while the for loop is a valid method, using themap
function is often preferred for rendering lists as it is more idiomatic in React.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop