How to Set Up onClick Event Handler on Link Component
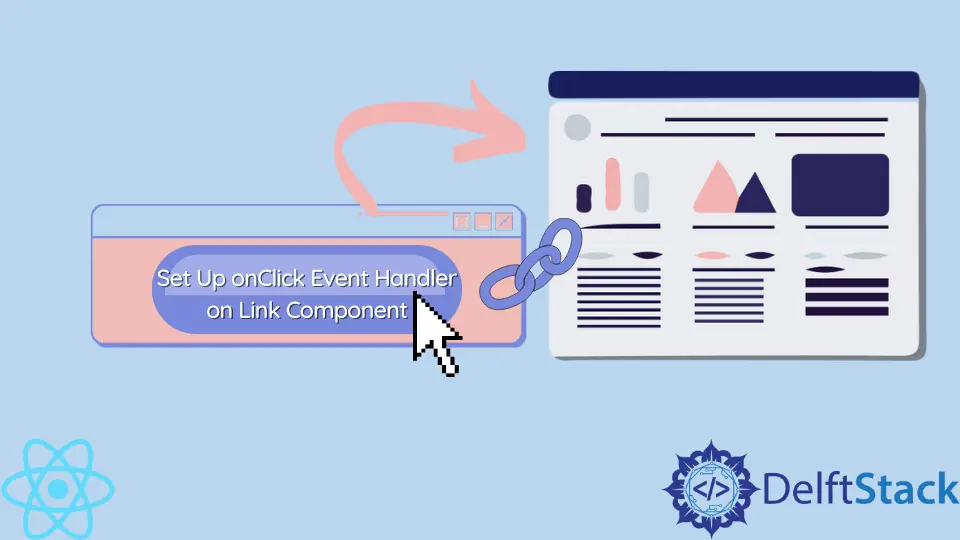
Setting up event handlers is an important part of building a React application.
onClick
is one of the most common events, usually defined on <button>
and <span>
elements. In this article, we’ll go through setting up an event handler for the Link
component.
Link
Component in React
React Router is the main library for configuring navigation in React.
It gives you all the building blocks necessary to build a navigation system. Link
is one of the most important components made available by React Router.
This component is a much more advanced alternative to the <a>
tag in HTML. People often use Link
components to build navigation menus or one-off links to pages within the app.
The component accepts many props, but the to
is probably the most important. This prop allows us to specify the path relative to the homepage, where the Link
component should navigate.
Let’s look at an example:
<Link to="/contact">Contact Us</Link>
Assuming our homepage is www.homepage.com
, clicking this link would take us to www.homepage.com/contact
. When the click event occurs, the default behavior of the Link
component is to navigate to the specified path.
However, what if we want to perform another operation besides the default behavior?
onClick
Attribute
Link
component also accepts an onClick
attribute, which should be set equal to a handler function (or a reference to it) within curly braces. Let’s look at a simple example:
import "./styles.css";
import { Link, BrowserRouter } from "react-router-dom"
export default function App() {
return (
<BrowserRouter>
<div className="App">
<Link to="/page" onClick={() => alert("boo")}>Clickable Link</Link>
</div>
</BrowserRouter>
);
}
In this simple example, we have a Link
component.
According to the default behavior, clicking it will take us to the /page
route. However, since we specified the onClick
attribute, it will also act as the event handler.
In this case, it will alert a simple text. You can take a look at codesandbox to try it out yourself.
Note that curly braces are necessary to ensure that the expression between them is treated as a valid JavaScript.
Handle onClick
Event for Link
Component in React
Unless you are 100% certain that your Link
component needs an onClick
event handler, it’s better to operate some other way.
Setting up a separate event handler will delay the navigation and defeat the purpose of using Link
components in the first place. The navigation will be delayed because the component will run the event handler first and navigate the path afterward.
Still, sometimes you need to perform a certain operation when the user reaches a specific page of your app. You can do that by changing the new component’s componentDidMount()
lifecycle method (or useEffect()
hook) instead.
This way, you can still execute a function when the user reaches a page. And there will be no delays to the navigation.
If you check out the example link yourself, you’ll notice the delay.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn