href onClick Event in React
-
Setting an
onClick
Action on a Link (<a></a>
) in React -
Setting an
onClick
Action on the Link Component From React Router -
Setting an
onClick
Action Inline Using an Arrow Function in React - Conclusion
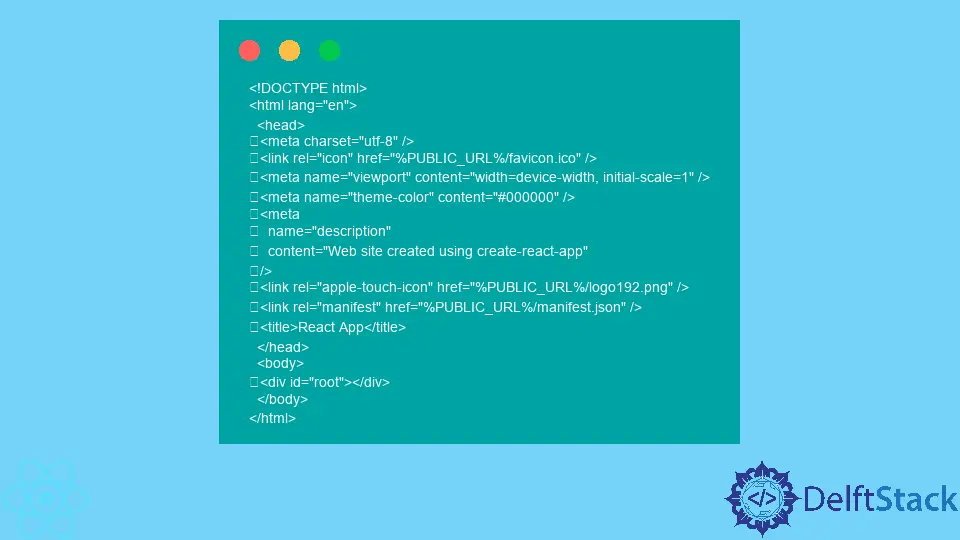
In modern web development, crafting interactive and compelling user interfaces is valuable for delivering a seamless user experience.
React, a powerful JavaScript library, empowers developers to effortlessly create versatile UI (User Interface) components for various use cases. One prevalent scenario involves the need to execute specific actions when a user interacts with a link (<a>
).
In React, handling user interactions is pivotal for constructing dynamic and responsive web applications. Capturing click events on HTML elements, notably the <a>
(Anchor) tag commonly used for navigation, is a fundamental aspect of this process.
Setting an onClick
Action on a Link (<a></a>
) in React
The Anchor tag, denoted by <a>
, serves as a primary vehicle for navigation within web applications.
In React, user interactions are handled through event-driven programming, and the onClick
event is pivotal for capturing mouse clicks on HTML elements.
To initiate an onClick
action for an Anchor tag, developers can employ the following syntax:
<a href="#" onClick={handleClick}>
Click me!
</a>
Here, the href="#"
attribute ensures that the link stays on the current page, while the onClick
attribute references a function, handleClick
, signaling React to execute specific logic when the user clicks the link.
The crucial part here is when developers define the handleClick
function. This function encapsulates the desired behavior upon link interaction.
For this example, consider a scenario where clicking the link triggers the display of a short message:
const handleClick = () => {
alert("You clicked the link!");
};
In this example, the handleClick
arrow function utilizes the alert
method to showcase a simple pop-up with a message that says, "You clicked the link!"
.
To implement this in full action, take a look at the React code snippet below.
import React from "react";
const CustomLink = () => {
const handleClick = () => {
alert("You clicked the link!");
};
return (
<div>
<p>Click the link below:</p>
<a href="#" onClick={handleClick}>
Click me!
</a>
</div>
);
};
export default CustomLink;
The given code defines a React functional component named CustomLink
. This component is designed to render a simple user interface that includes a paragraph and a clickable link.
The primary purpose of the component is to display a message when the user clicks on the link.
Within the component, there is a function called handleClick
. This function is responsible for showing an alert with the message "You clicked the link!"
when invoked.
The handleClick
function is linked to the onClick
event of the anchor (<a>
) tag, meaning it will be executed when the user clicks on the link.
The return
statement in the component contains JSX (JavaScript XML) code specifying the structure of the rendered UI. It includes a div
element wrapping a paragraph (<p>
) with the text "Click the link below:"
and an anchor (<a>
) tag with the text "Click me!"
.
Output before clicking the link:
When a user clicks on the "Click me!"
link, the handleClick
function will be triggered, displaying an alert with the specified message.
Output after clicking the link:
The final output confirms that when the user clicks the "Click me"
link, an alert box will pop up with the message "You clicked the link!"
.
Setting an onClick
Action on the Link Component From React Router
In React, the <Link>
component from the react-router-dom
library is commonly used for navigation within a single-page application.
While it seamlessly handles navigation, there are scenarios where you might want to introduce custom behavior when a user clicks on a link, such as triggering an action before navigating. One such customization involves setting an onClick
action on a link.
This section guides developers through the process of attaching a custom onClick
action to a <Link>
component in React.
Sample code:
import React from "react";
import { Link } from "react-router-dom";
const CustomLink = () => {
// Custom click event handler
const handleClick = (e) => {
e.preventDefault(); // Prevent default link behavior
alert("You clicked the link!");
};
return (
<div>
<p>Click the link below:</p>
<Link to="/" onClick={handleClick}>
Click me!
</Link>
</div>
);
};
export default CustomLink;
Output before clicking the link:
The React code above defines a functional React component called CustomLink
. Inside this component, the necessary dependencies are imported, including React and the Link
component from react-router-dom
.
Next, a function named handleClick
is declared for handling the custom click event. This function takes an event (e
) as a parameter.
Within the handleClick
function, the e.preventDefault()
code is used to prevent the default behavior of the link. This ensures that the browser doesn’t navigate to the specified route immediately.
The return
statement includes JSX code. Inside, a paragraph is created with the text "Click the link below:"
and a <Link>
component. The to
prop of the <Link>
specifies the route (/
in this case), and the onClick
prop is set to our custom handleClick
function.
Output after clicking the link:
Once the link is clicked, an alert will appear with the message "You clicked the link!"
This demonstrates that the custom onClick
action is successfully triggered, showcasing the effectiveness of this method in React application development.
Setting an onClick
Action Inline Using an Arrow Function in React
In React development, creating interactive user interfaces often involves handling events triggered by user actions. The onClick
event is commonly used to execute specific actions when an element, such as a button or link, is clicked.
One concise and effective approach is setting the onClick
action inline using an arrow function.
This method streamlines the process, especially for small-scale actions, and contributes to more readable and maintainable code.
Example code:
import React from "react";
const CustomLink = () => {
return (
<div>
<p>Click the link below:</p>
<a href="#" onClick={() => alert("You clicked the link!")}>
Click me!
</a>
</div>
);
};
export default CustomLink;
Output before clicking the link:
In this React functional component named CustomLink
, the <a>
(anchor) element represents the clickable link.
The href
attribute is set to "#"
to make it a non-navigating link, ensuring it doesn’t impact the page’s URL.
The crucial part is the onClick
attribute of the link. Instead of referencing a separate function, an arrow function is used directly within the onClick
attribute.
This inline arrow function contains the desired action—in this case, triggering an alert with the message "You clicked the link!"
Output after clicking the link:
When a user clicks the Click me!
link, an alert will promptly appear with the message You clicked the link!
This showcases the immediate response achieved by employing the inline onClick
action, demonstrating a straightforward and effective way to handle click events in a React application.
Conclusion
In conclusion, the onClick
action empowers developers to execute custom JavaScript logic when users click on a link (<a>
), opening opportunities for seamless integration of additional functionalities.
Employing an onClick
action on a React <Link>
component offers a direct method to introduce custom behavior during user interaction with links. This customization enables the execution of specific actions before navigation, enhancing the user experience while maintaining the advantages of React’s client-side routing.
Utilizing an inline arrow function for the onClick
action provides a concise and readable solution for handling click events in React components, which is particularly beneficial for small, focused actions.
This article has illustrated a streamlined implementation of the onClick
action with an anchor tag in React. Developers can extend this functionality for diverse purposes, including page navigation or executing complex actions with data.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn